How to Use the Python __str__() Method
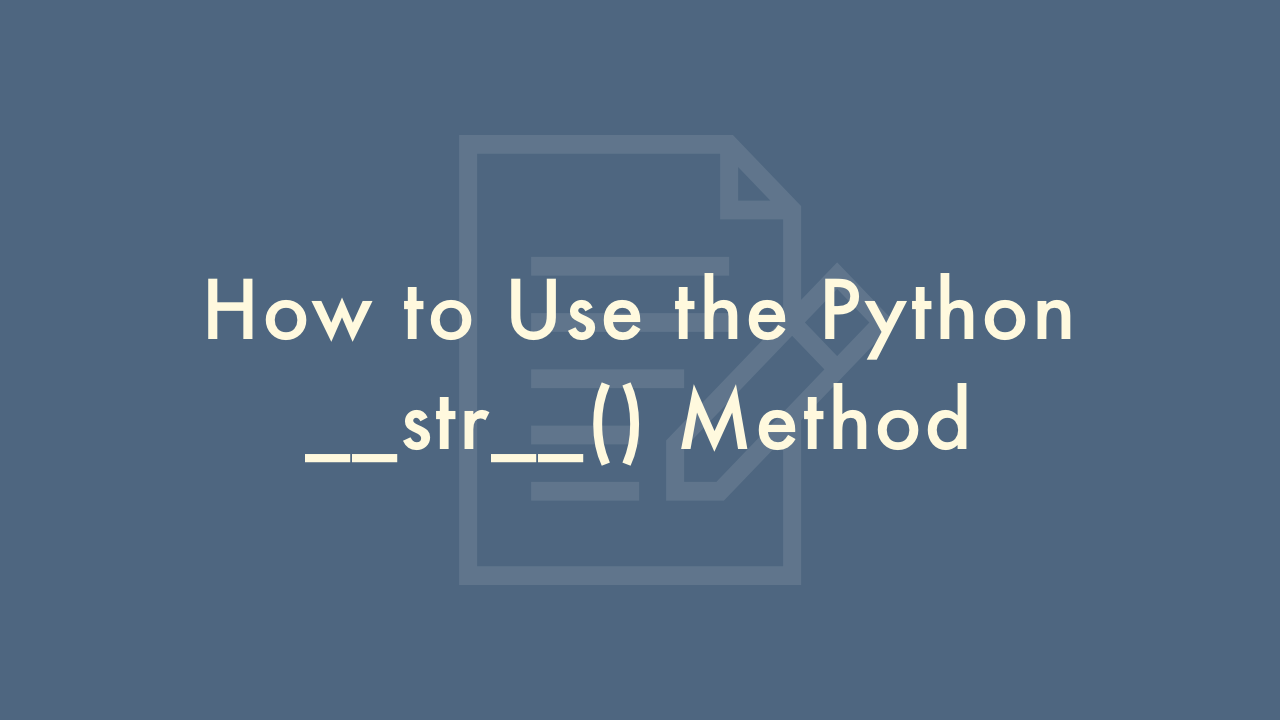
Contents
In this article, you will learn how to use the Python __str__() method.
Python __str__() Method
The __str__() method is a special method in Python that is used to define how an object should be converted to a string representation. This method is called by the built-in str() function and also by the print() function when an object is passed to it. Here’s an example of how to use the __str__() method in Python:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"{self.name} is {self.age} years old"
In this example, we define a class called Person with an __init__() method that initializes the name and age attributes of a Person object. We also define a __str__() method that returns a string representation of the Person object.
To create an instance of the Person class and print it using the print() function, we can do the following:
person = Person("John Doe", 30)
print(person)
# Output:
# John Doe is 30 years old
In this example, the __str__() method is called when the person object is passed to the print() function. The __str__() method returns a formatted string representation of the Person object, which is then printed to the console.
By implementing the __str__() method in a class, you can customize the string representation of objects created from that class, making it easier to debug and read.