How to Use the Python lxml Library
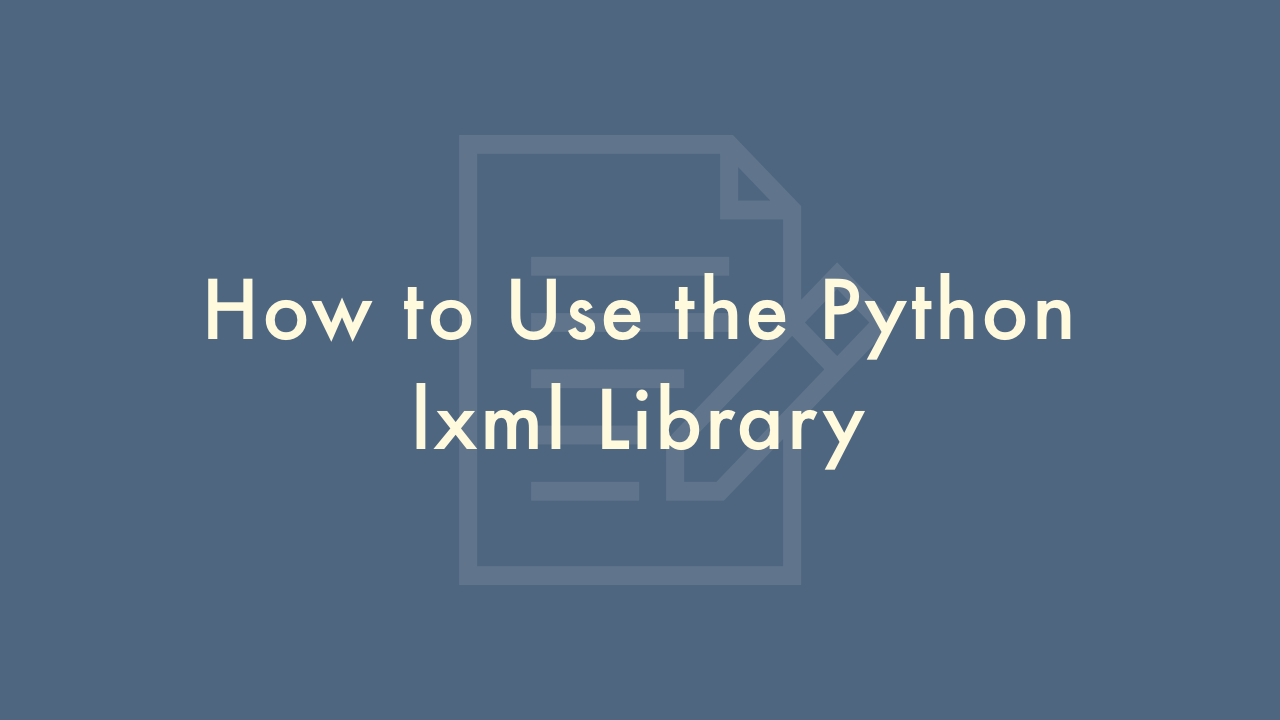
Contents
In this article, you will learn how to use the Python lxml library.
Python lxml Library
The Python lxml library is a powerful library for processing XML and HTML documents. Here’s a brief guide on how to use lxml:
Installation:
You can install lxml using pip by running the following command:
pip install lxml
Parsing XML or HTML Documents:
To parse an XML or HTML document using lxml, you need to create an Element object. Here’s an example of how to parse an XML document:
from lxml import etree
xml = "hello world "
root = etree.fromstring(xml)
Navigating the Document:
Once you have an Element object, you can navigate the document using various methods. Here are some examples:
# Get the root element
root = tree.getroot()
# Get the child elements of the root
children = root.getchildren()
# Get the value of an element
value = root.find("element").text
# Get all elements with a specific tag name
elements = root.findall("element")
Modifying the Document:
You can modify the XML or HTML document using various methods. Here are some examples:
# Add a new element to the document
new_element = etree.Element("new_element")
root.append(new_element)
# Remove an element from the document
element_to_remove = root.find("element")
root.remove(element_to_remove)
# Update the value of an element
element_to_update = root.find("element")
element_to_update.text = "new value"
Outputting the Document:
Once you’ve made changes to the XML or HTML document, you can output it as a string or write it to a file. Here are some examples:
# Output the XML as a string
xml_string = etree.tostring(root)
# Write the XML to a file
with open("output.xml", "wb") as f:
f.write(etree.tostring(root))
That’s a brief introduction to using the lxml library in Python. There are many more features and methods available, so be sure to consult the documentation for more information.