How to Play Music with Python Winsound
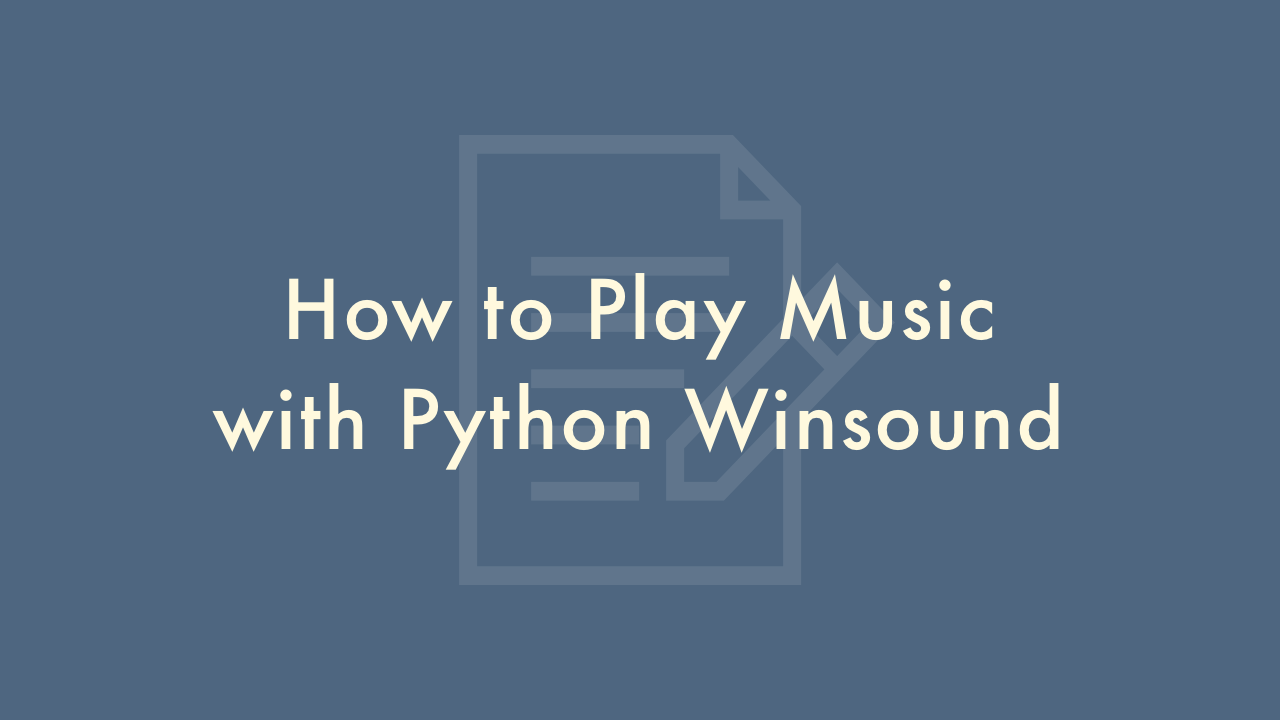
Contents
In this article, you will learn how to play music with Python winsound.
Play music with Python winsound
The Python winsound module allows you to play sound files and tones on Windows operating systems. Here’s how you can use it to play music in Python:
-
Import the winsound module:
import winsound
-
Call the winsound.PlaySound() function, passing the filename of the music file you want to play as the first argument. You can use either a WAV file or a sound event from the list of predefined sound events provided by the winsound module.
Here’s an example of playing a WAV file:
winsound.PlaySound('C:\\Users\\Username\\Music\\example.wav', winsound.SND_FILENAME)
In this example, replace “C:\\Users\\Username\\Music\\example.wav” with the actual path to the WAV file you want to play.
-
You can also use predefined sound events provided by the winsound module. Here’s an example of playing a system sound:
winsound.PlaySound(winsound.SND_ALIAS_SYSTEMASTERISK, winsound.SND_ALIAS_ID)
In this example, SND_ALIAS_SYSTEMASTERISK specifies the system sound event to play, and SND_ALIAS_ID specifies that we’re using a sound event rather than a filename.
With these steps, you can play music in Python using the winsound module.