How to Use the Python isinstance() Function
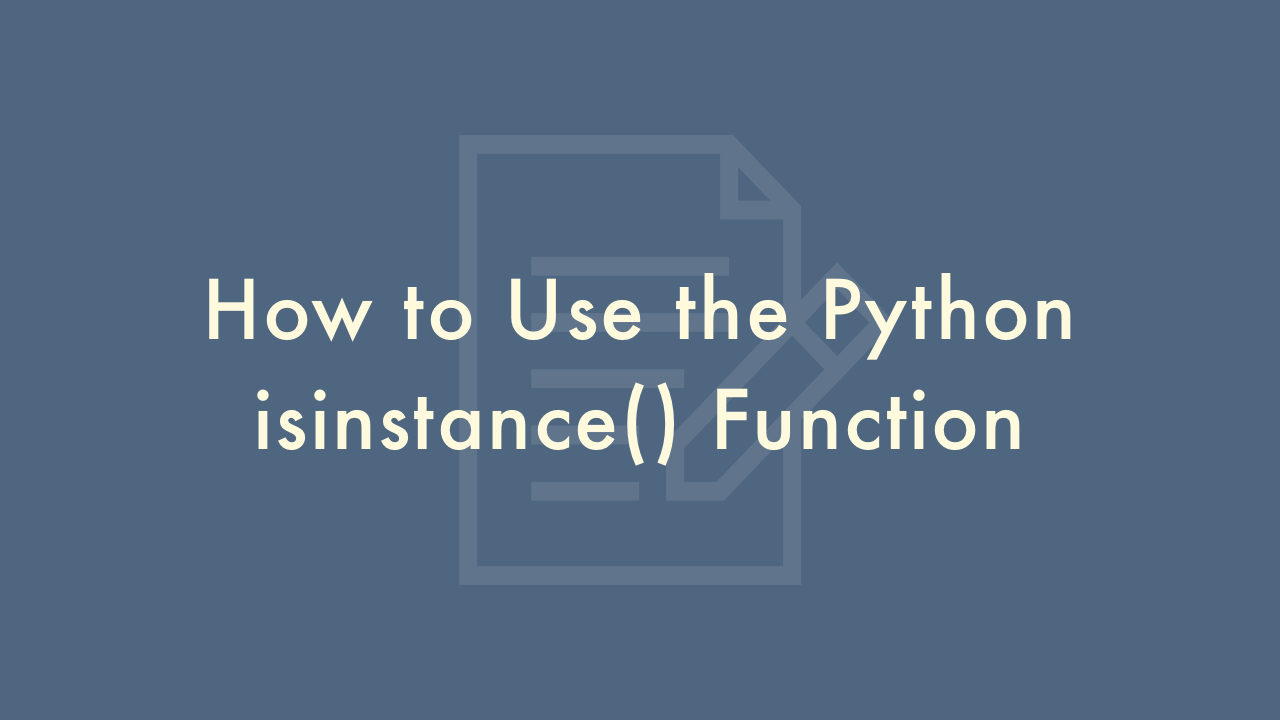
Contents
In this article, you will learn how to use the Python isinstance() function.
Python isinstance() Function
The isinstance() function in Python is used to check if an object belongs to a particular class or not. It takes two arguments: the first argument is the object that you want to check, and the second argument is the class that you want to check against.
Syntax
Here’s the syntax for using the isinstance() function:
isinstance(object, classinfo)
Parameters
object
: The object that you want to check.classinfo
: The class or type that you want to check against.
The classinfo parameter can be a tuple of classes or types, which means that you can check if an object is an instance of any of the classes in the tuple.
Example
Here’s an example:
class Dog:
pass
class Cat:
pass
class Bird:
pass
my_dog = Dog()
my_cat = Cat()
my_bird = Bird()
print(isinstance(my_dog, Dog)) # Output: True
print(isinstance(my_cat, Dog)) # Output: False
print(isinstance(my_bird, (Dog, Cat))) # Output: False
In this example, we define three classes: Dog, Cat, and Bird. We create objects of these classes, my_dog, my_cat, and my_bird. We then use the isinstance() function to check if each object is an instance of the Dog class, the Cat class, and a tuple of the Dog and Cat classes. The output shows that my_dog is an instance of Dog, my_cat is not an instance of Dog, and my_bird is not an instance of either Dog or Cat.
You can use the isinstance() function to check if an object is an instance of built-in Python types such as str, int, float, list, tuple, dict, set, etc. You can also use it to check if an object is an instance of a custom-defined class that you have created in your Python program.