How to Use the Python glob() Function
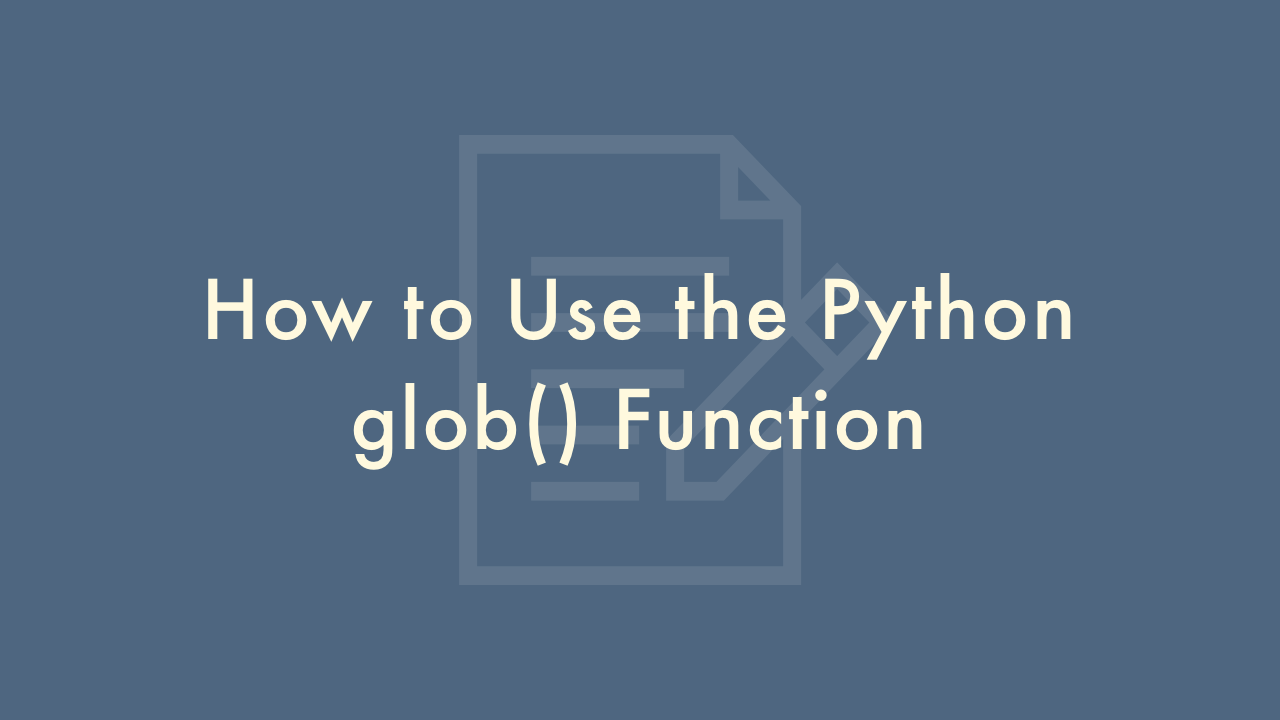
Contents
In this article, you will learn how to use the Python glob() function .
Python glob() Function
The glob module in Python provides a function glob() that allows you to search for files and directories matching a specified pattern. Here’s a simple example to demonstrate how to use the glob() function:
import glob
# Find all files ending with '.txt' in the current directory
files = glob.glob('*.txt')
# Print the list of matching files
print(files)
In this example, glob.glob(‘*.txt’) returns a list of all the files in the current directory that end with ‘.txt’.
You can also use wildcards to search for files and directories in subdirectories. For example:
import glob
# Find all files ending with '.py' in the current directory and its subdirectories
files = glob.glob('**/*.py', recursive=True)
# Print the list of matching files
print(files)
In this example, glob.glob(‘**/*.py’, recursive=True) returns a list of all the files ending with ‘.py’ in the current directory and its subdirectories. The “recursive=True” argument tells the glob() function to search recursively through all the subdirectories.
Here’s some additional information that can help you use the glob function more effectively:
Pattern matching
The glob() function uses Unix shell-style wildcard patterns to match filenames and directories. Some common wildcards are “*” (matches any number of characters), “?” (matches exactly one character), and “[]” (matches any character specified within the square brackets). For example, the pattern “*.txt” matches all files that end with “.txt”, “file?.txt” matches files such as “file1.txt” and “file2.txt”, and “file[0-9].txt” matches files such as “file1.txt” and “file2.txt”.
Sorting the results
By default, the glob() function returns the list of matching files and directories in the order in which they are found. However, you can sort the results by using the sorted() function in Python. For example:
import glob
# Find all files ending with '.txt' in the current directory
files = glob.glob('*.txt')
# Sort the list of matching files
files = sorted(files)
# Print the list of matching files
print(files)
Error handling
If the pattern passed to the glob() function does not match any files or directories, it returns an empty list. If there is an error while searching for files, such as a permission error or a non-existent directory, the glob() function raises an exception. You can handle these exceptions using a try…except block in Python. For example:
import glob
try:
# Find all files ending with '.txt' in the current directory
files = glob.glob('*.txt')
# Print the list of matching files
print(files)
except Exception as e:
# Print the error message
print(f'Error: {e}')
Note that the glob function only returns the names of the files and directories that match the pattern, not the contents of the files. To access the contents of a file, you can use the open function in Python.