How to Zip and Unzip Files in Python
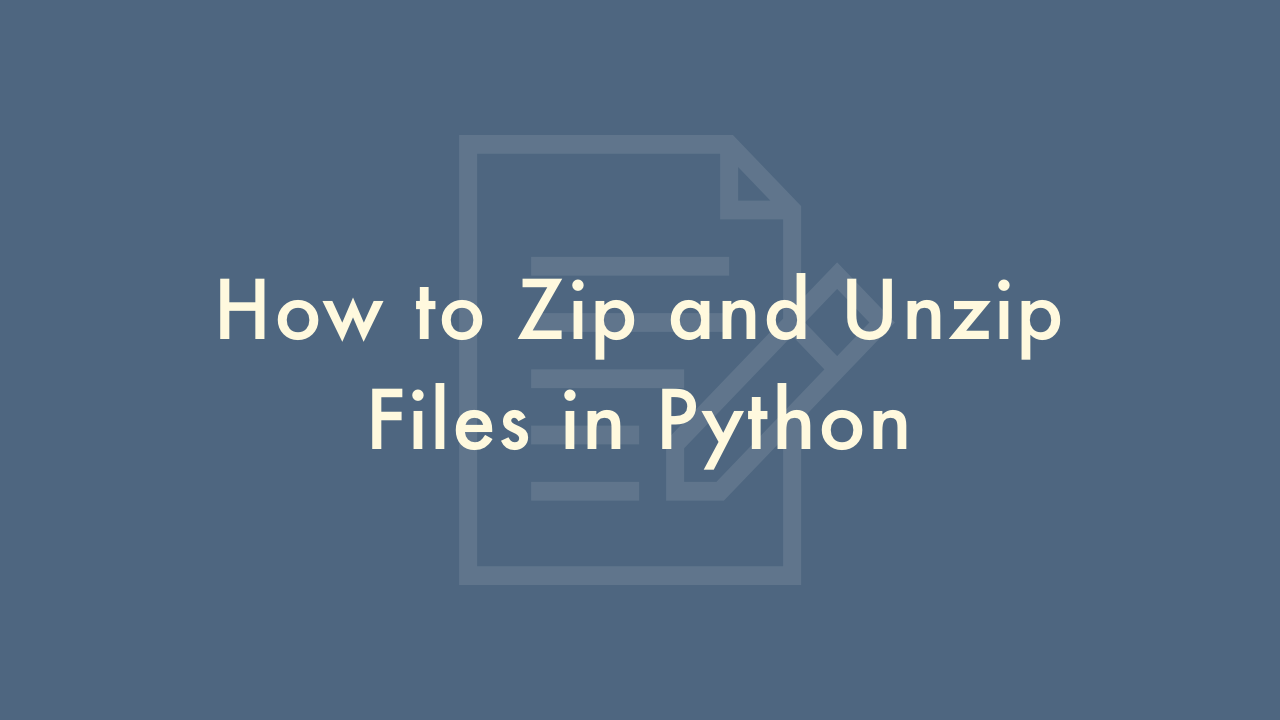
Contents
In this article, you will learn how to zip and unzip files in Python.
Zipping files
To compress a file or a directory into a ZIP file, you can use the ZipFile class from the zipfile module. Here’s an example:
import zipfile
# Create a new ZIP file and add some files to it
with zipfile.ZipFile('my_archive.zip', 'w') as zip_file:
zip_file.write('file1.txt')
zip_file.write('file2.txt')
zip_file.write('directory1')
# The ZIP file has been created
In this example, we use the ZipFile class to create a new ZIP file called my_archive.zip. We then use the write() method to add two files (file1.txt and file2.txt) and a directory (directory1) to the archive.
Unzipping files
To extract files from a ZIP archive, you can again use the ZipFile class. Here’s an example:
import zipfile
# Extract all files from the ZIP archive
with zipfile.ZipFile('my_archive.zip', 'r') as zip_file:
zip_file.extractall('extracted_files')
# The files have been extracted to the "extracted_files" directory
In this example, we use the ZipFile class to open an existing ZIP file called my_archive.zip. We then use the extractall() method to extract all files from the archive to a directory called extracted_files.
If you want to extract only certain files from the archive, you can use the extract() method instead of extractall(), and specify the filenames to extract as arguments.
import zipfile
# Extract specific files from the ZIP archive
with zipfile.ZipFile('my_archive.zip', 'r') as zip_file:
zip_file.extract('file1.txt', 'extracted_files')
zip_file.extract('directory1/file3.txt', 'extracted_files')
# The specified files have been extracted to the "extracted_files" directory
In this example, we use the extract() method to extract two specific files (file1.txt and directory1/file3.txt) from the archive to the extracted_files directory.