How to Use the Python List append() Method
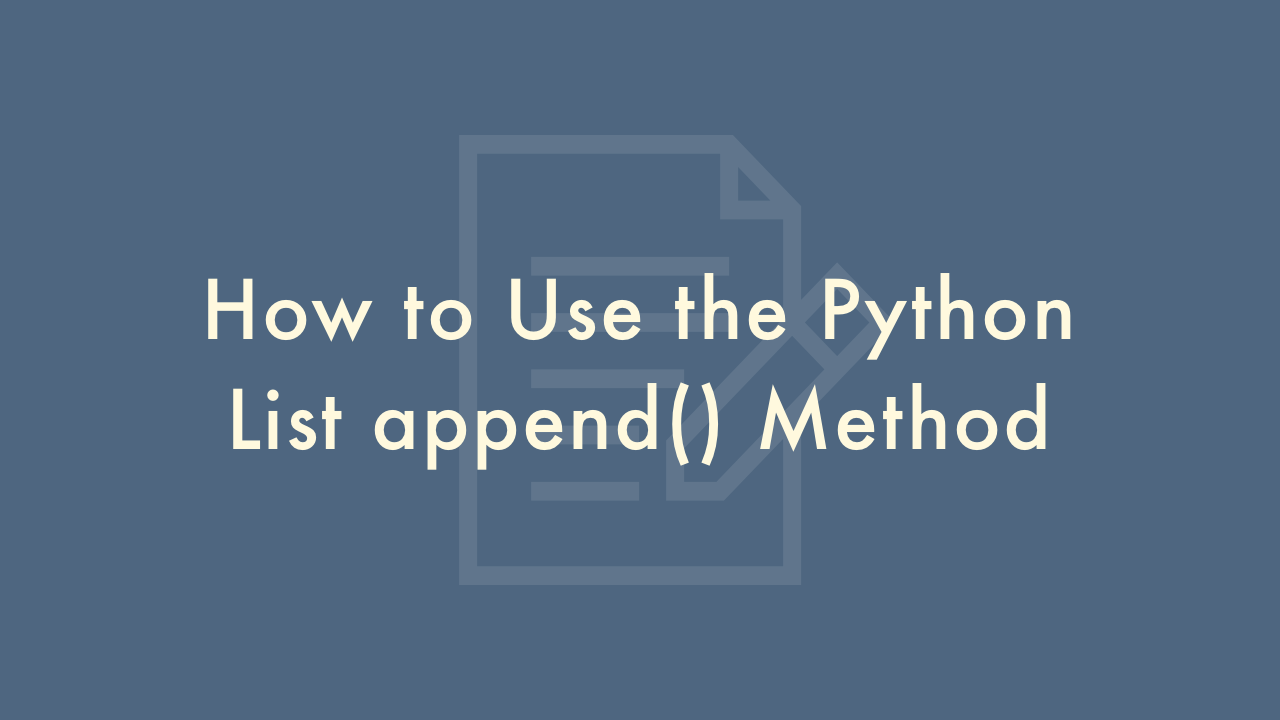
09/07/2021
Contents
In this article, you will learn how to use the Python list append() method.
Python append() Method
The append() method is a built-in method in Python, and it is used to add an element to the end of a list. It takes one argument, which is the element that you want to add to the list. The element can be of any data type, including numbers, strings, or even other lists.
Syntax:
list.append(element)
Example:
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange')
print(fruits)
//Output
//['apple', 'banana', 'cherry', 'orange']
Here’s an example that demonstrates how to use the append() method with different data types:
# Adding a string to a list
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange')
print(fruits)
# Adding a number to a list
numbers = [1, 2, 3, 4]
numbers.append(5)
print(numbers)
# Adding a list to a list
lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
lists.append([10, 11, 12])
print(lists)
//Output
//['apple', 'banana', 'cherry', 'orange']
//[1, 2, 3, 4, 5]
//[[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
It’s important to note that the append() method modifies the list in place and returns None. This means that the original list is changed and the method does not return the updated list. To see the updated list, you need to print the list after using the append() method.