The Difference between Map() and Filter() in Python
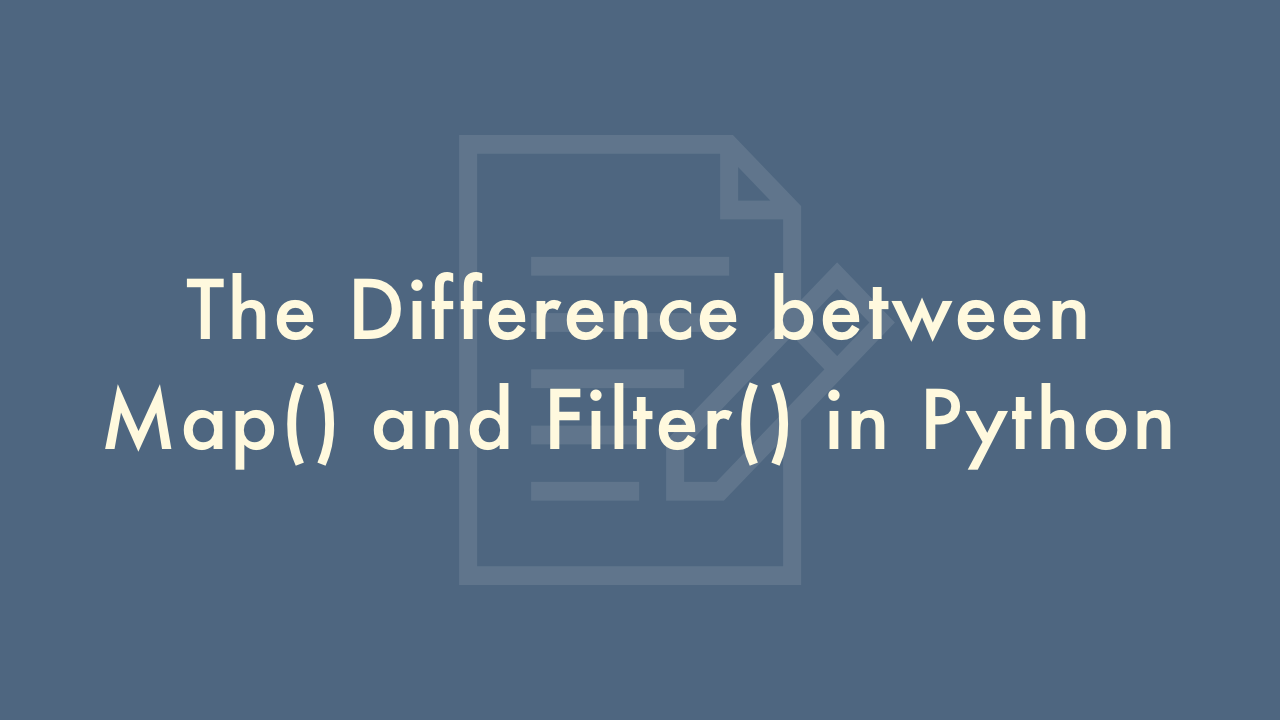
Contents
In this article, you will learn about the difference between map() and filter() in Python.
The difference between map() and filter()
map()
and filter()
are both built-in Python functions that can be used to transform and filter sequences such as lists, tuples, and sets.
The main difference between map() and filter() is what they do with the elements in the input sequence:
-
map() applies a function to each element in the sequence and returns a new sequence with the transformed elements. The transformed elements can be of a different type than the original elements. For example, if we have a list of numbers and we want to square each number, we can use map() as follows:
numbers = [1, 2, 3, 4, 5] squares = map(lambda x: x**2, numbers) print(list(squares)) # Output: [1, 4, 9, 16, 25]
-
filter() returns a new sequence containing only the elements from the input sequence that satisfy a given condition. The condition is specified as a function that returns True or False for each element in the sequence. For example, if we have a list of numbers and we want to filter out the even numbers, we can use filter() as follows:
numbers = [1, 2, 3, 4, 5] odds = filter(lambda x: x % 2 == 1, numbers) print(list(odds)) # Output: [1, 3, 5]
In summary, map() is used to apply a function to each element of a sequence and return a new sequence with the transformed elements, while filter() is used to return a new sequence containing only the elements from the input sequence that satisfy a given condition.