How to Use Python Bisect Module
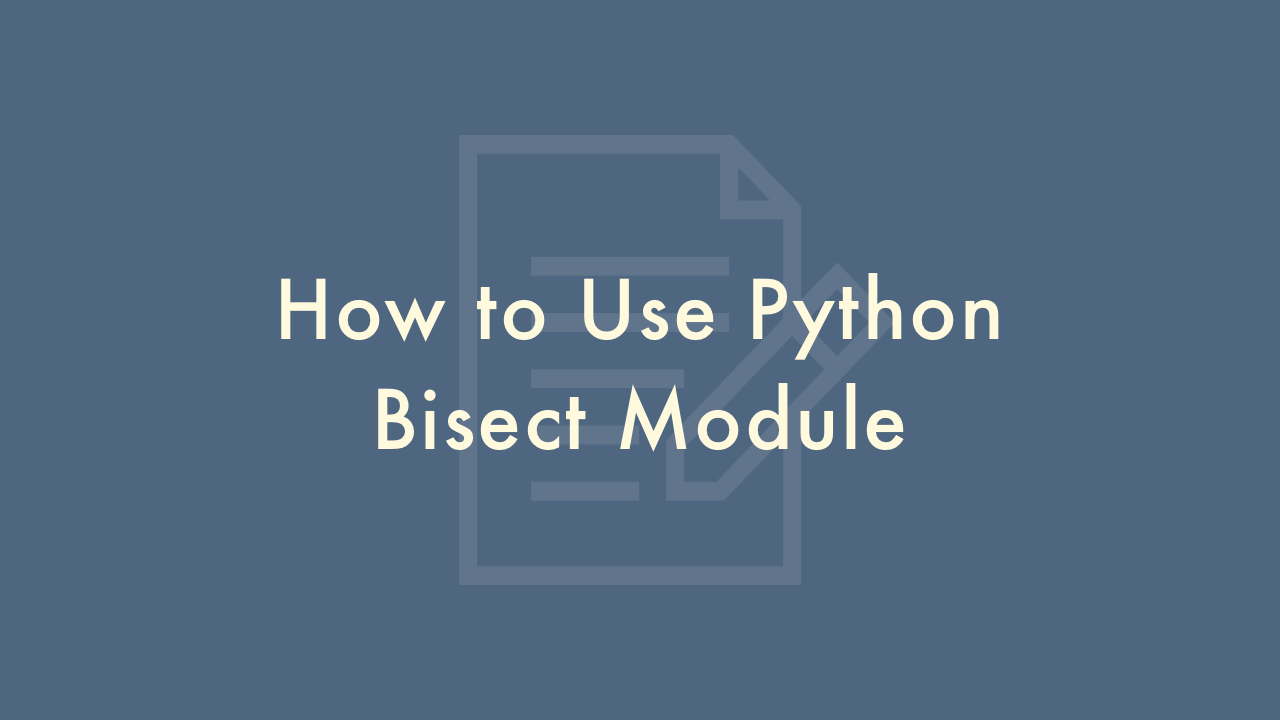
Contents
In this article, you will learn how to use Python bisect module.
Python Bisect Module
The bisect module in Python provides a fast and efficient way to search and insert items into sorted lists. Here’s how you can use it:
Searching a Sorted List
To search for an item in a sorted list, you can use the bisect_left() or bisect_right() functions, which will return the index at which the item should be inserted to maintain the sorted order of the list. If the item is already in the list, bisect_left() will return its leftmost index, and bisect_right() will return its rightmost index.
Here’s an example:
import bisect
my_list = [1, 3, 4, 4, 6, 8, 9]
item = 4
index = bisect.bisect_left(my_list, item)
if index != len(my_list) and my_list[index] == item:
print(f"Found {item} at index {index}")
else:
print(f"{item} not found in the list")
# Output: Found 4 at index 2
Inserting into a Sorted List
To insert an item into a sorted list, you can use the insort_left() or insort_right() functions, which will insert the item at the correct index to maintain the sorted order of the list.
Here’s an example:
import bisect
my_list = [1, 3, 4, 6, 8, 9]
item = 5
bisect.insort_left(my_list, item)
print(my_list)
# Output: [1, 3, 4, 5, 6, 8, 9]