How to Replace a String with the Python replace() Method
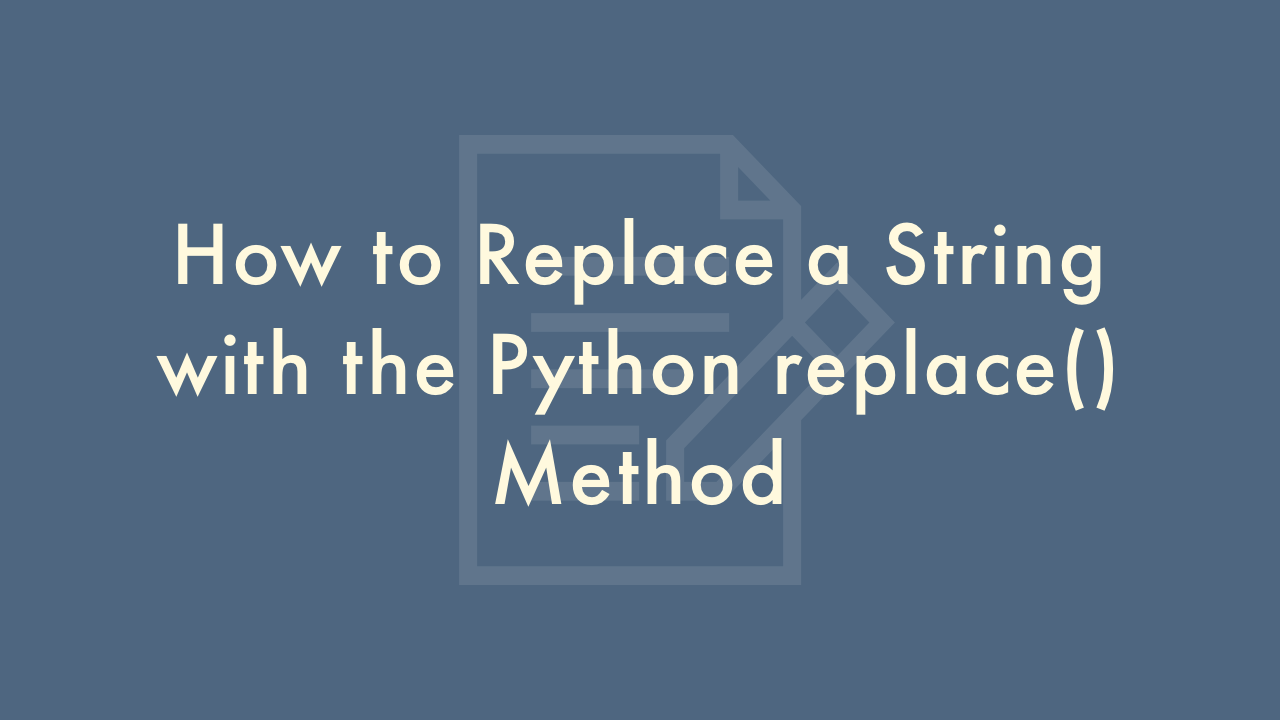
09/10/2021
Contents
In this article, you will learn how to replace a string with the Python replace() method.
Python replace() Method
You can replace a string in Python using the replace() method.
Syntax
The syntax for the method is as follows:
string.replace(old, new, count)
Parameters
old
: The string that you want to replacenew
: The string that you want to replace the old string withcount
(optional): The number of occurrences of the old string that you want to replace. If this parameter is not specified, all occurrences of the old string will be replaced.
Example
Here’s an example that demonstrates how to use the replace() method:
sentence = "Hello, my name is Plantpot."
new_sentence = sentence.replace("Plantpot", "John Doe")
print(new_sentence)
# Output
# "Hello, my name is John Doe."
Note that the replace() method does not modify the original string, it returns a new string with the desired replacements. If you want to modify the original string, you need to assign the result of the replace() method back to the original string, like this:
sentence = sentence.replace("Plantpot", "John Doe")