How to Use the Numpy random.uniform() Function in Python
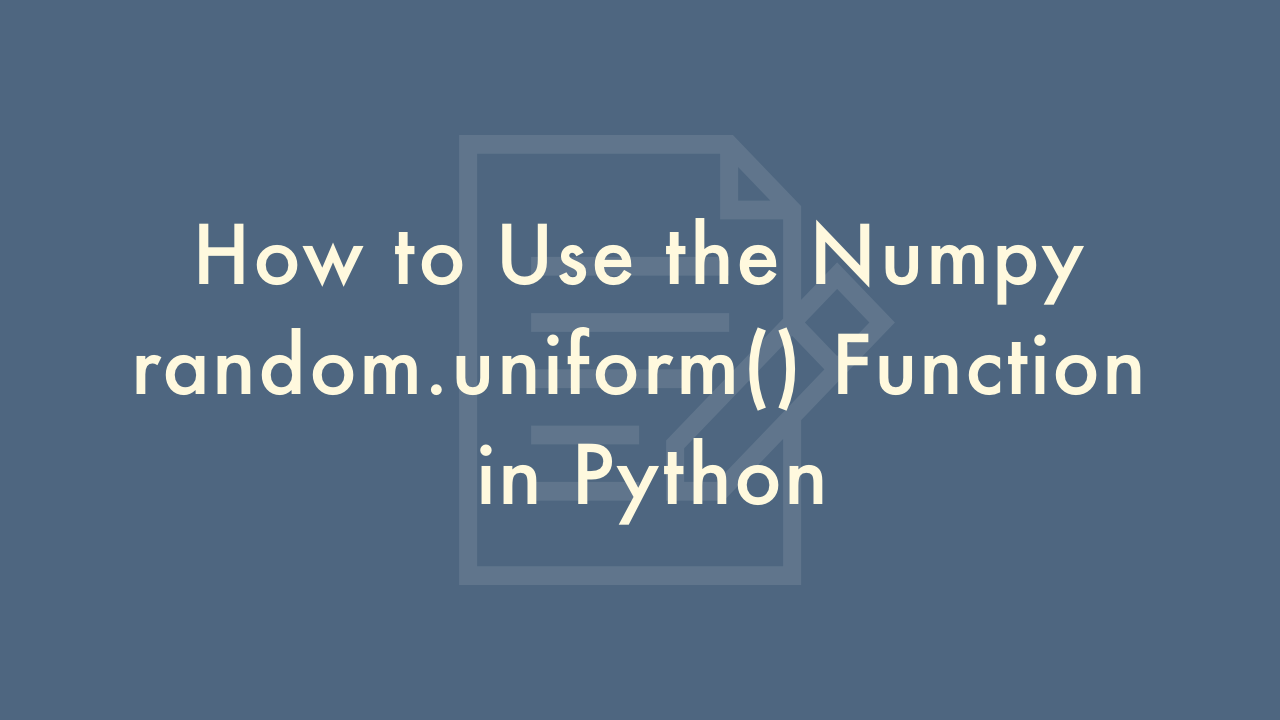
Contents
In this article, you will learn how to use the Numpy random.uniform() function in Python.
The Numpy random.uniform() Function
The Numpy random.uniform() function in Python is used to generate random numbers from a uniform distribution. The uniform distribution is a probability distribution where all values in the range are equally likely to occur. The function takes in several arguments that allow you to customize the distribution of the generated random numbers.
Here’s how to use the random.uniform() function in Python:
Import the Numpy module
import numpy as np
Call the random.uniform() function
np.random.uniform(low=0.0, high=1.0, size=None)
The function takes three arguments:
low
(optional): This specifies the lower bound of the range of numbers to be generated. The default value is 0.0.high
(optional): This specifies the upper bound of the range of numbers to be generated. The default value is 1.0.size
(optional): This specifies the shape of the output. If None (default), a single value is returned. If an integer, a 1D array of that length is returned. If a tuple, an array of that shape is returned.
Generate random numbers
To generate a single random number from the uniform distribution between 0 and 1, call the function with no arguments:
np.random.uniform()
To generate multiple random numbers, specify the size argument:
np.random.uniform(size=10)
This will generate an array of 10 random numbers between 0 and 1.You can also specify the low and high arguments to generate random numbers from a different range:
np.random.uniform(low=-1.0, high=1.0, size=5)
This will generate an array of 5 random numbers between -1 and 1.
Use the generated random numbers
The random.uniform() function returns an array of random numbers, which can be used in any way you like. For example, you can assign the generated numbers to a variable:
x = np.random.uniform(size=10)
You can then use this variable in mathematical operations or other calculations.