How to Use the Python staticmethod() Function
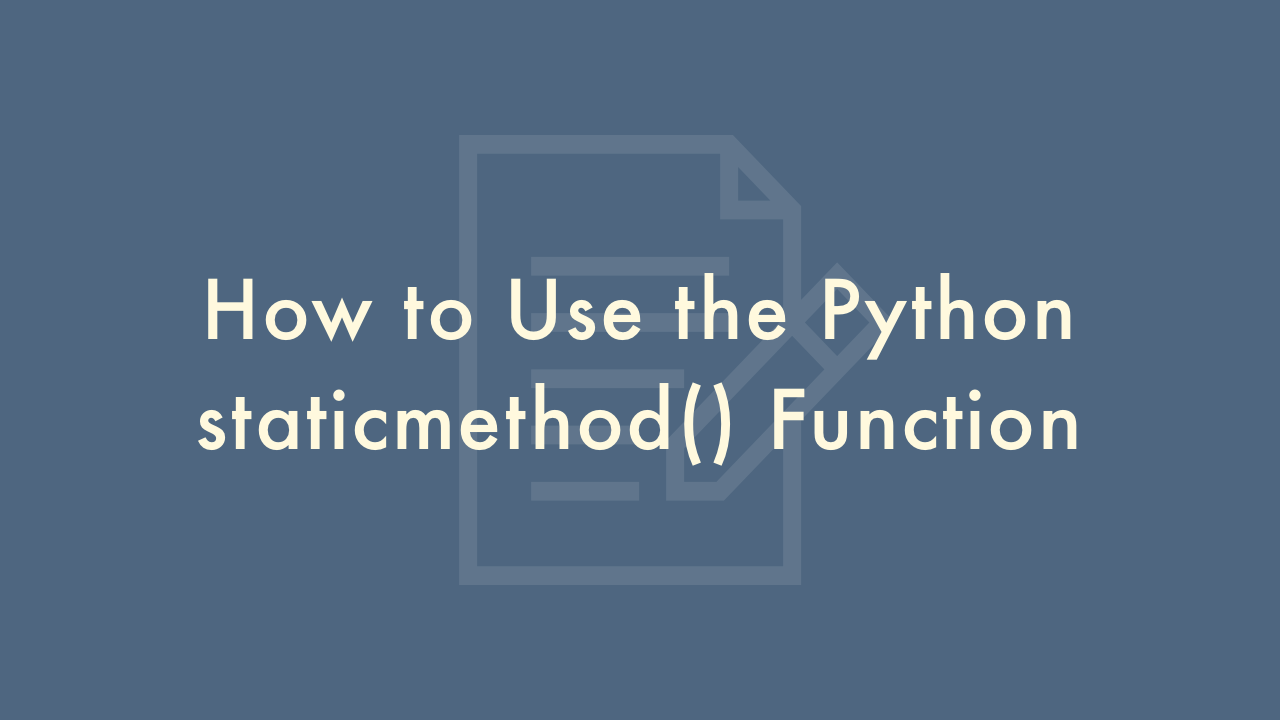
Contents
In this article, you will learn how to use the Python staticmethod() function.
Python staticmethod() Function
The staticmethod() function is a built-in function in Python that is used to declare a static method in a class. A static method is a method that belongs to a class rather than an instance of the class, and it can be called without creating an instance of the class. Here’s how to use the staticmethod() function in Python:
Declare the static method inside a class using the @staticmethod decorator:
class MyClass:
@staticmethod
def my_static_method():
# Method logic here
Call the static method using the class name:
MyClass.my_static_method()
Note that when calling a static method, you do not need to create an instance of the class first. The static method is called directly from the class.
Here’s an example that demonstrates the use of a static method to calculate the area of a circle:
class Circle:
PI = 3.14
def __init__(self, radius):
self.radius = radius
@staticmethod
def calculate_area(radius):
return Circle.PI * radius ** 2
print(Circle.calculate_area(5)) # Output: 78.5
In the example above, the calculate_area() method is a static method that takes a radius as an argument and calculates the area of a circle using the PI constant and the radius provided. The static method can be called directly from the class, as shown in the print() statement.