How to Use Python “in” Operator
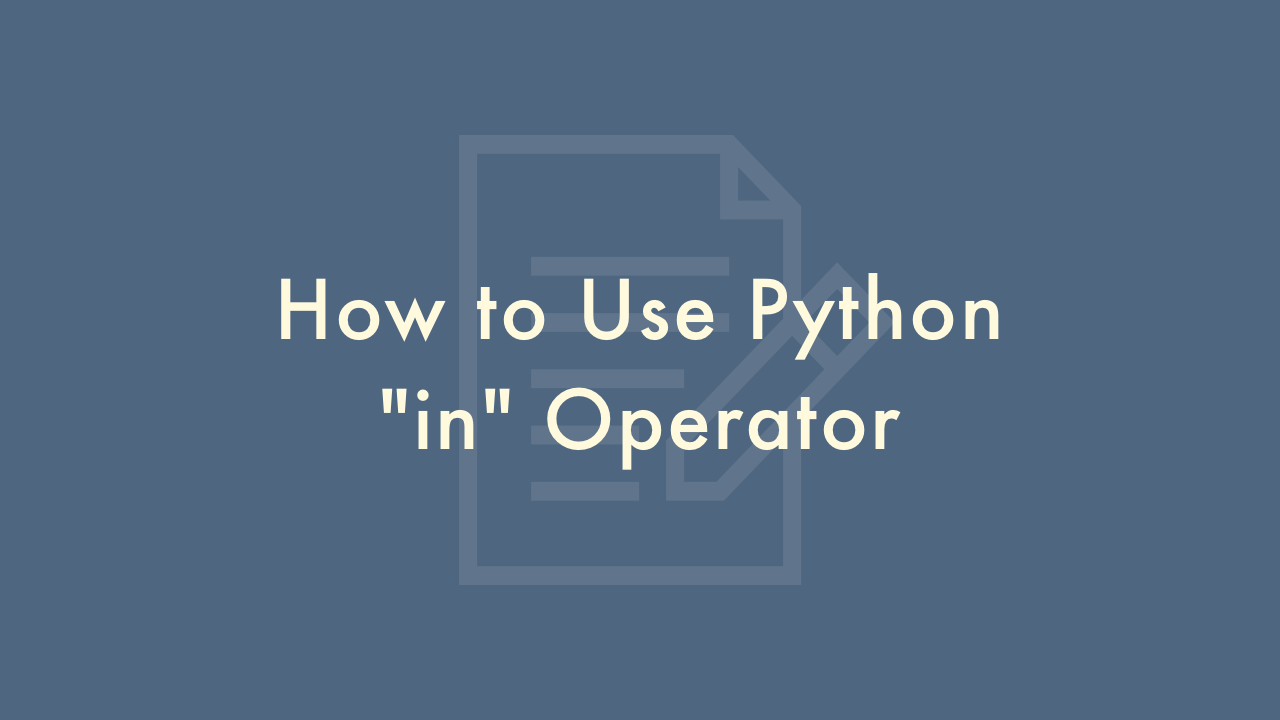
Contents
In this article, you will learn how to use Python “in” operator.
How to use Python “in” operator
In Python, the in operator is used to check whether a value is present in a sequence or collection such as a list, tuple, or string. The syntax for the in operator is as follows:
value in sequence
Here, value is the item you want to check for, and sequence is the collection you want to search in. The in operator returns a Boolean value of True if value is present in sequence, and False otherwise.
Here are some examples of using the in operator in Python:
# Check if a value is present in a list
fruits = ['apple', 'banana', 'orange']
print('banana' in fruits) # True
print('grape' in fruits) # False
# Check if a character is present in a string
name = 'John Doe'
print('e' in name) # True
print('z' in name) # False
# Check if a key is present in a dictionary
student = {'name': 'Alice', 'age': 21, 'major': 'Computer Science'}
print('age' in student) # True
print('gender' in student) # False
Note that the in operator can also be used with other collections such as sets and tuples, and it can also be negated using the not in operator.
Here are some more details about the in operator in Python:
- The in operator works by iterating over the elements of the sequence and checking if the given value is equal to any of the elements in the sequence.
- The in operator can be used with any sequence or collection that supports iteration, including lists, tuples, strings, sets, and dictionaries.
- When used with dictionaries, the in operator checks if the given value is present in the keys of the dictionary. To check if a value is present in the values of a dictionary, you can use the values() method.
- The in operator can also be used with custom objects, as long as the objects define the __contains__() method. This method should return True if the given value is present in the object, and False otherwise.
- In addition to the in operator, Python also provides the not in operator, which is used to check if a value is not present in a sequence or collection.
- When used with strings, the in operator checks if the given substring is present in the string. For example, ‘hello’ in ‘hello world’ would return True.
Overall, the in operator is a very useful tool in Python for checking whether a value is present in a sequence or collection, and it can be used in a variety of different contexts.