How to Measure the Execution Time of a Python Script
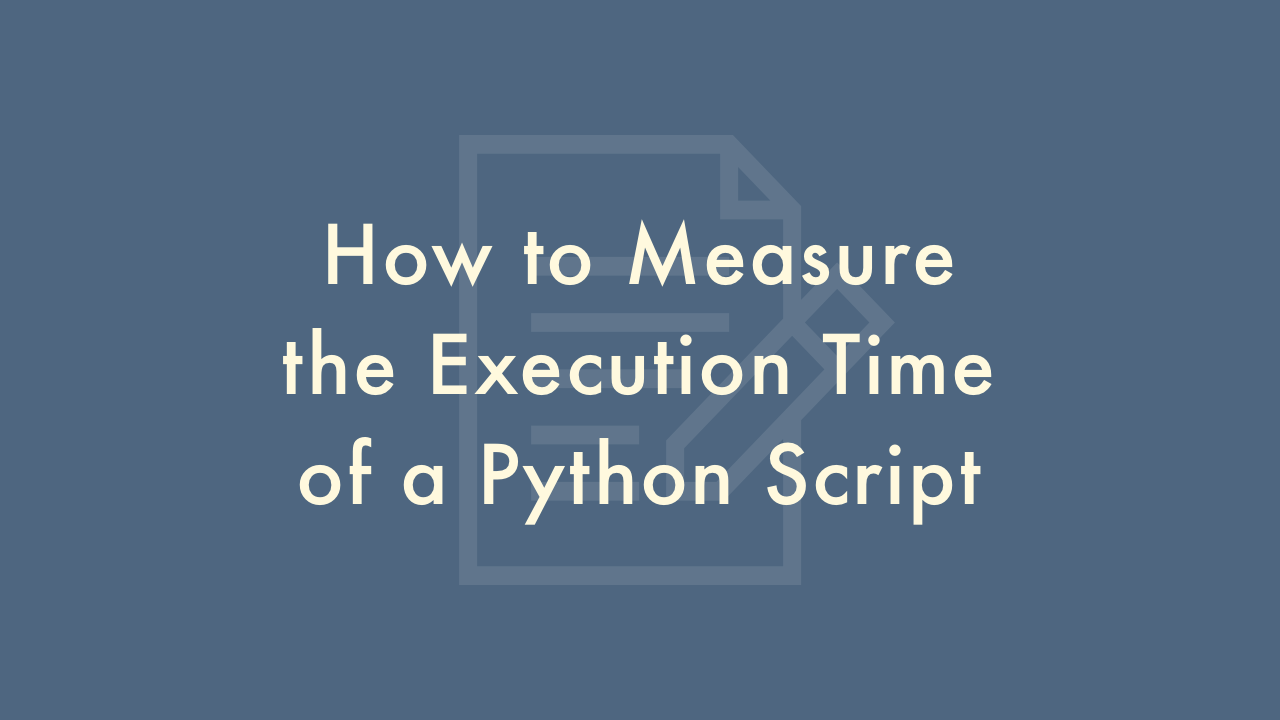
Contents
In this article, you will learn how to measure the execution time of a Python script.
Measure the execution time of a Python script
You can measure the execution time of a Python script using the time module. There are two ways to measure the execution time of a Python script:
Using the time function:
The time function from the time module can be used to measure the execution time of a Python script. You can use the time function to get the current time before and after the execution of the script and then calculate the difference to get the total execution time. Here’s an example:
import time
start_time = time.time()
# Your Python code here
end_time = time.time()
total_time = end_time - start_time
print("Total execution time:", total_time)
This code will print the total execution time in seconds.
Using the timeit module:
The timeit module provides a simple way to measure the execution time of small code snippets. You can use the timeit module to run a Python statement or expression multiple times and calculate the average execution time. Here’s an example:
import timeit
code_to_test = """
# Your Python code here
"""
total_time = timeit.timeit(code_to_test, number=1000)
print("Total execution time:", total_time)
This code will run the code snippet 1000 times and print the total execution time in seconds. You can adjust the number of times the code snippet is run by changing the number parameter.