How to Use Python PuLP Library
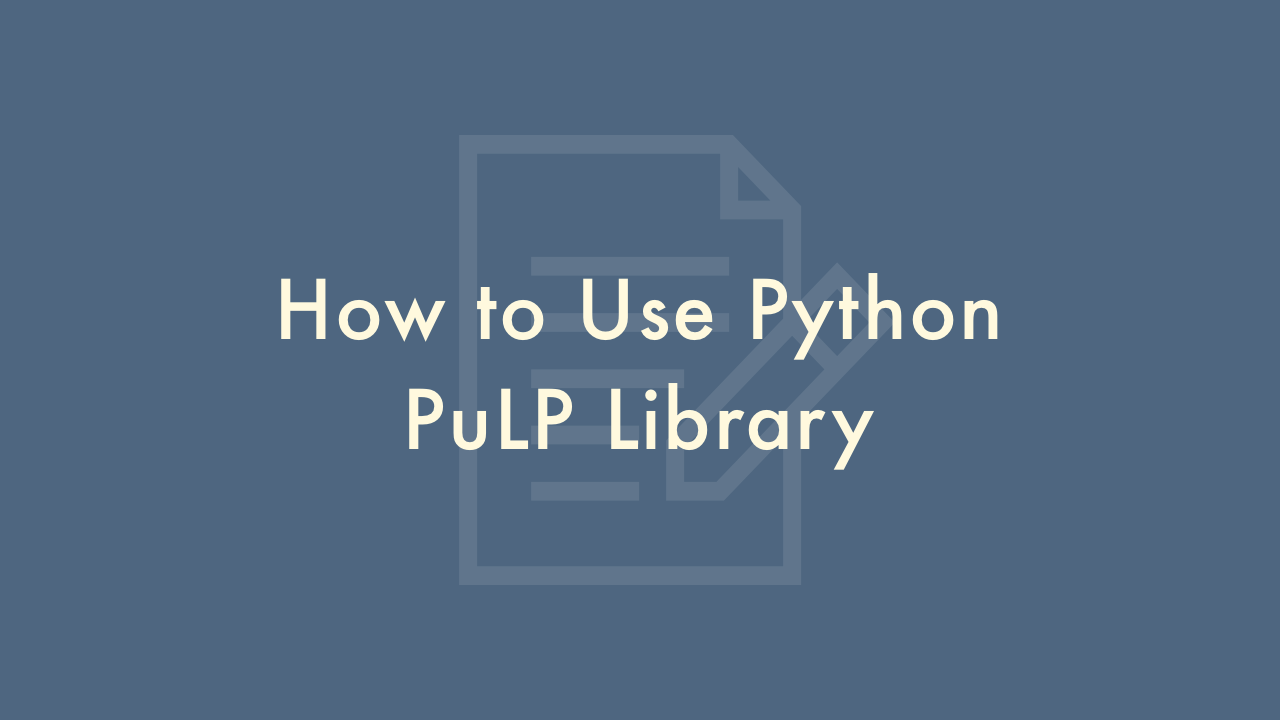
Contents
In this article, you will learn how to use Python PuLP library.
Python PuLP Library
PuLP is a popular open-source linear programming library in Python that allows you to solve optimization problems. It provides an intuitive interface to create linear programming models and can be used to solve a wide range of problems including resource allocation, scheduling, and transportation. Here’s a step-by-step guide to using the PuLP library in Python:
Install PuLP
You can install PuLP using pip, a package manager for Python. Open a terminal and type the following command:
pip install pulp
Import PuLP
To use PuLP in your Python code, you need to import it at the beginning of your script. You can do this with the following code:
import pulp
Create a Linear Programming Problem
To create a linear programming problem, you need to define the objective function, the constraints, and the decision variables. For example, consider the following optimization problem:
Maximize: 3x + 5y
Subject to:
x + 2y <= 10
3x + y <= 12
x >= 0, y >= 0
To create this problem in PuLP, you can use the following code:
# Create a maximization problem
problem = pulp.LpProblem('Maximizing Problem', pulp.LpMaximize)
# Define decision variables
x = pulp.LpVariable('x', lowBound=0)
y = pulp.LpVariable('y', lowBound=0)
# Define objective function
problem += 3*x + 5*y
# Define constraints
problem += x + 2*y <= 10
problem += 3*x + y <= 12
# Print the problem
print(problem)
Solve the Problem
To solve the problem, you can use the solve() method of the problem object. The solve() method will return the status of the solution (whether it's optimal, infeasible, or unbounded), and the optimal values of the decision variables.
# Solve the problem
status = problem.solve()
# Print the status of the solution
print(pulp.LpStatus[status])
# Print the optimal values of the decision variables
print('x:', pulp.value(x))
print('y:', pulp.value(y))
# Print the optimal value of the objective function
print('Optimal Value:', pulp.value(problem.objective))
The output of the code above will be:
Maximizing Problem:
MAXIMIZE
3*x + 5*y + 0
SUBJECT TO
_C1: x + 2 y <= 10
_C2: 3 x + y <= 12
VARIABLES
x Continuous
y Continuous
Optimal
x: 2.0
y: 4.0
Optimal Value: 22.0
As you can see, the optimal values of x and y are 2 and 4, respectively, and the optimal value of the objective function is 22.
This is just a simple example, but PuLP can be used to solve much more complex optimization problems. With some practice, you can use PuLP to solve a wide range of optimization problems.