How to Use the Python strip() Function
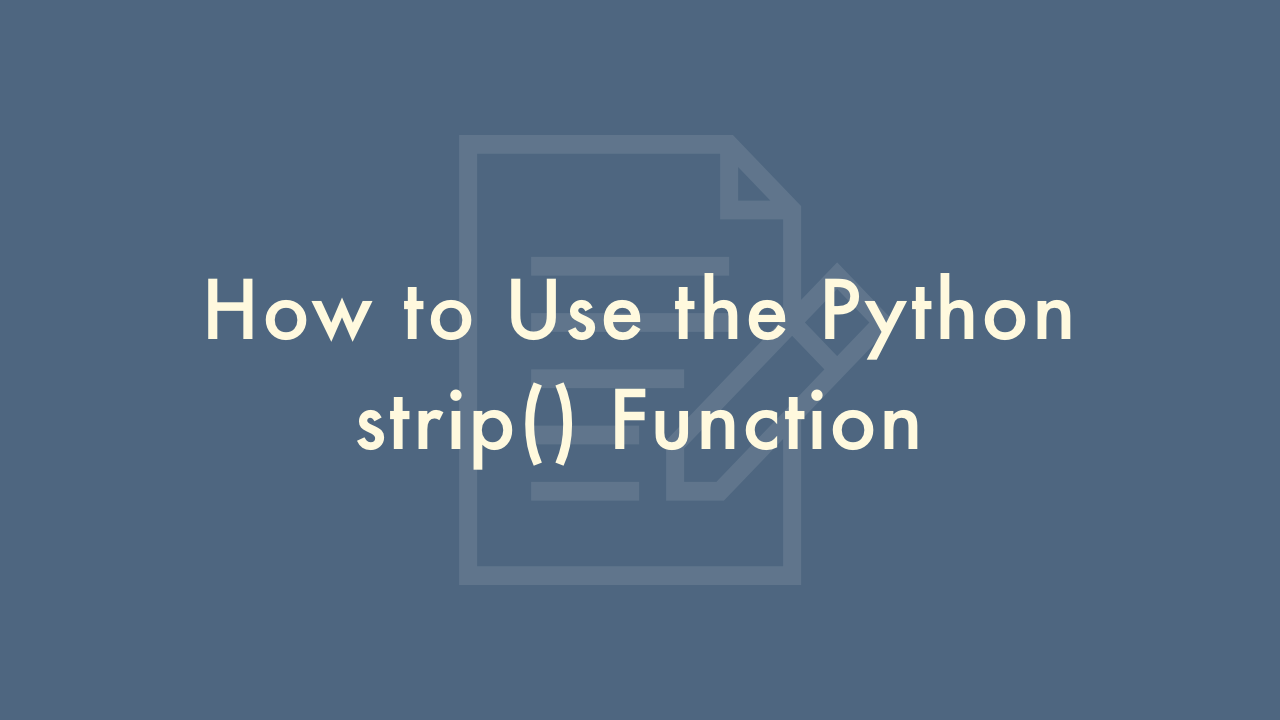
Contents
In this article, you will learn how to use the Python strip() function.
Python strip() Function
The strip() function in Python is used to remove whitespace characters from the beginning and end of a string. This function can be particularly useful when working with user input, as it can help remove any extra whitespace that the user may have accidentally included.
Syntax:
string.strip([chars])
Parameters:
chars
(optional): a string specifying the set of characters to be removed. If omitted or None, the function removes whitespace characters.
Return Value:
A new string with the leading and trailing characters removed.
The strip() function can remove any leading and trailing characters that are contained in the chars parameter, which can be any string. For example, you could use the strip() function to remove all leading and trailing hyphens from a string by calling strip(‘-‘).
Example:
Here are some examples of how to use the strip() function in various scenarios:
# Remove whitespace from both ends of a string
text = " hello, world! "
stripped_text = text.strip()
print(stripped_text) # Output: "hello, world!"
# Remove a specific character from both ends of a string
text = "~~~hello, world!~~~"
stripped_text = text.strip("~")
print(stripped_text) # Output: "hello, world!"
# Remove leading whitespace from a string
text = " hello, world! "
stripped_text = text.lstrip()
print(stripped_text) # Output: "hello, world! "
# Remove trailing whitespace from a string
text = " hello, world! "
stripped_text = text.rstrip()
print(stripped_text) # Output: " hello, world!"
Note that the strip() function does not modify the original string; it returns a new string with the leading and trailing characters removed.