How to Save Images with Python Pillow
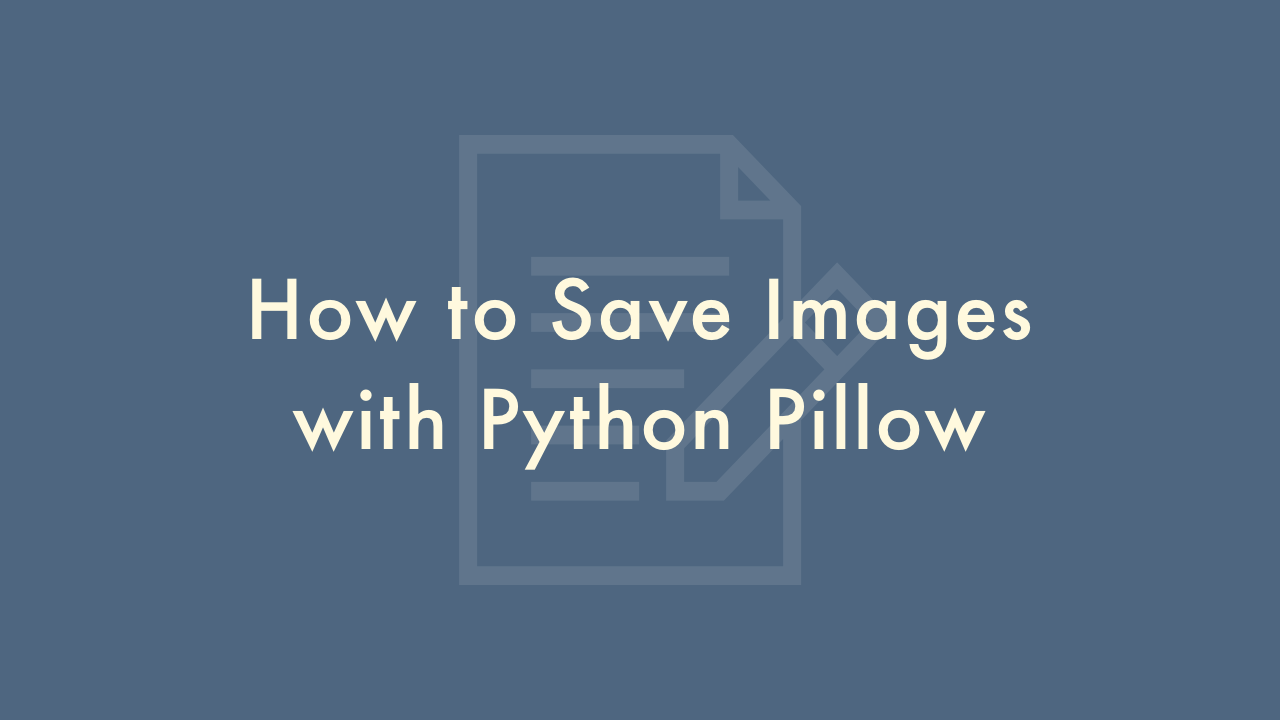
Contents
In this article, you will learn how to save images with Python Pillow.
Save Images with Python Pillow
To save images with Python Pillow, you can use the save() method of the Image class. Here’s an example of how to do it:
from PIL import Image
# Open the image file
image = Image.open('example.png')
# Modify the image as desired (e.g. resize, crop, filter)
# Save the modified image as a new file
image.save('modified_example.png')
In this example, the Image class from the Pillow module is used to open the file ‘example.png’ and assign it to the variable image. The image can then be modified using any of the methods available in the Image class.
Finally, the save() method is called on the image object, and the filename ‘modified_example.png’ is passed as an argument to save the modified image to a new file.
You can also specify the file format and quality when saving the image using additional arguments. For example, to save the image as a JPEG file with a quality of 90%, you can do:
image.save('modified_example.jpg', format='JPEG', quality=90)
In this case, the file extension is changed to ‘.jpg’ to indicate the JPEG file format, and the format and quality arguments are passed to the save() method to specify the file format and quality.