How to Use the Python File writelines() Method
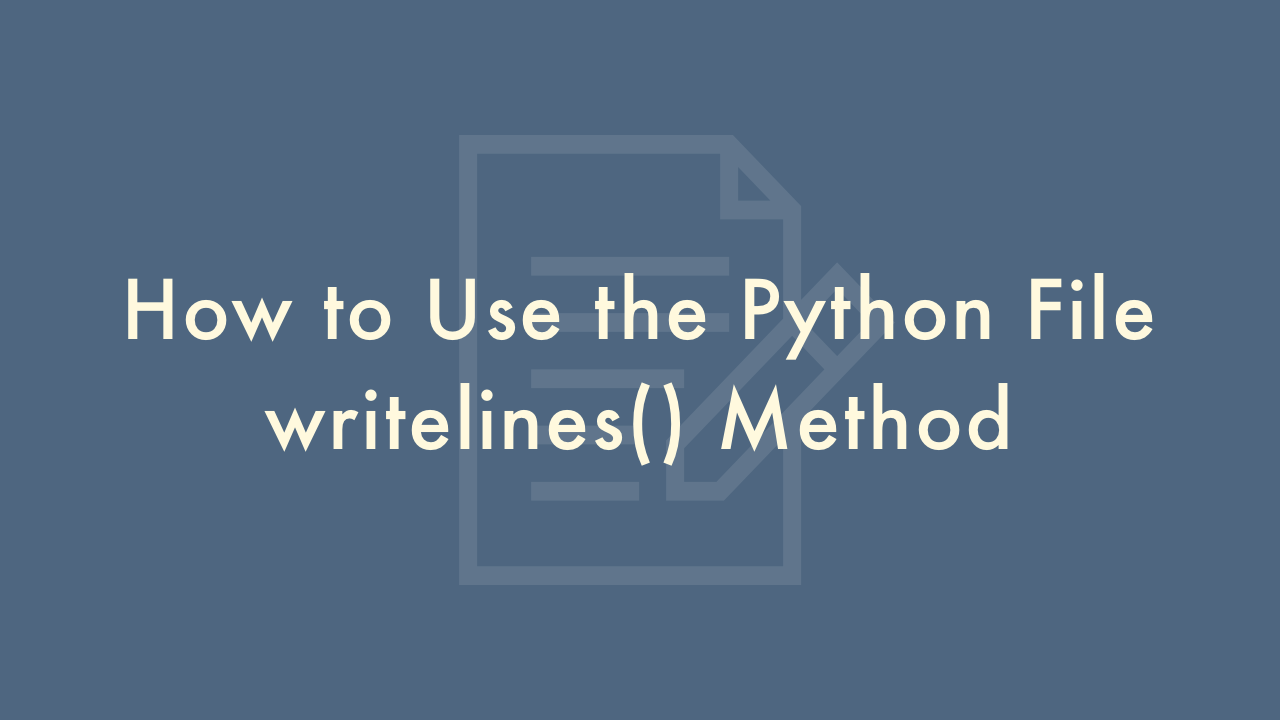
Contents
In this article, you will learn how to use the Python File writelines() method.
File writelines() Method
The writelines() method is used to write a list of strings to a file. In Python, this method can be used to write multiple lines to a file at once. Here is how to use the writelines() method in Python:
Open the file you want to write to using the open() function with the appropriate mode parameter. For example, to open a file named example.txt in write mode, you can use the following code:
file = open("example.txt", "w")
Create a list of strings that you want to write to the file. For example:
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
Note that each string in the list should end with a newline character (\n) if you want each line to be written on a separate line in the file.
Use the writelines() method to write the list of strings to the file. For example:
file.writelines(lines)
Close the file when you’re done using the close() method. For example:
file.close()
Here’s the complete example code:
file = open("example.txt", "w")
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
file.writelines(lines)
file.close()
This will write the three lines of text to the file example.txt.