How to Use the Python NumPy arange() Function
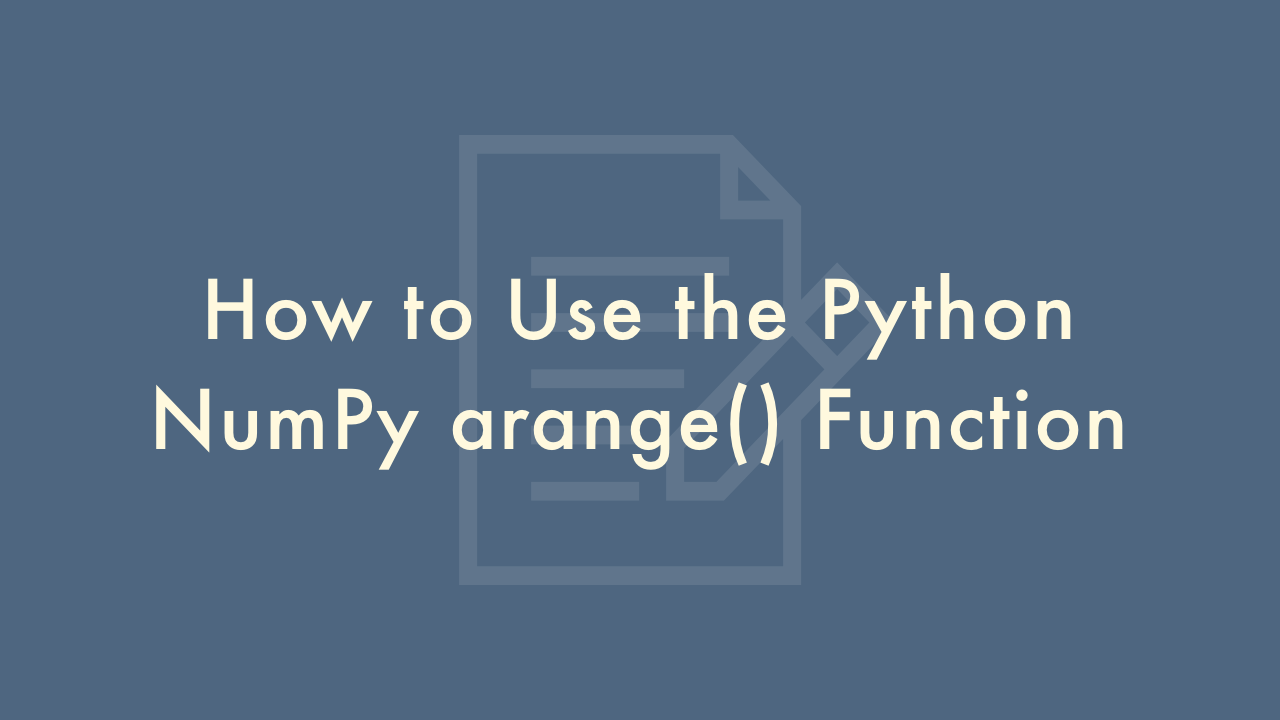
Contents
In this article, you will learn how to use the Python numPy arange() function.
Python NumPy arange() Function
The numpy.arange() function is a function in the NumPy library that is used to generate evenly spaced values within a given range. It is similar to the range() function in Python but with some additional features and functionality.
Syntax
The basic syntax of the numpy.arange() function is as follows:
numpy.arange(start, stop, step, dtype)
Parameters
Here is a brief explanation of each of the parameters:
start
: The start value of the sequence. It is an optional parameter and default value is 0.stop
: The end value of the sequence, i.e., the sequence will include values up to but not including stop. It is a required parameter.step
: The step size between each value in the sequence. It is an optional parameter and the default value is 1.dtype
: Specifies the data type of the output array. It is an optional parameter and the default value is float.
Example
Here is an example that demonstrates how to use the numpy.arange() function to generate an array of evenly spaced values:
import numpy as np
# Generate an array of evenly spaced values from 0 to 9 with a step size of 1
a = np.arange(0, 10)
print(a)
# Output
# [0 1 2 3 4 5 6 7 8 9]
Here is another example that generates an array of evenly spaced values from 2 to 11 with a step size of 2:
import numpy as np
# Generate an array of evenly spaced values from 2 to 11 with a step size of 2
b = np.arange(2, 12, 2)
print(b)
# Output
# [ 2 4 6 8 10]
In this way, you can use the numpy.arange() function to generate arrays of evenly spaced values based on your requirements.
The numpy.arange() function is a powerful tool for generating arrays in NumPy, and it has several advantages over the built-in range() function in Python.
For example, numpy.arange() allows you to specify a step size that is not an integer, which is not possible with the range() function. Additionally, the numpy.arange() function returns a NumPy array, which is a more powerful and versatile data structure than a Python list. NumPy arrays offer a wide range of mathematical operations that can be performed on the array elements, which can be useful for scientific and numerical computing.
Here’s another example that demonstrates the versatility of numpy.arange():
import numpy as np
# Generate an array of evenly spaced values from 0 to 1 with a step size of 0.1
c = np.arange(0, 1, 0.1)
print(c)
# Output
# [0. 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9]
You can also use the numpy.arange() function with complex numbers. For example:
import numpy as np
# Generate an array of evenly spaced complex numbers from 1 + 2j to 5 + 2j with a step size of 1 + 0j
d = np.arange(1 + 2j, 5 + 2j, 1 + 0j)
print(d)
# Output
# [1.+2.j 2.+2.j 3.+2.j 4.+2.j]
In summary, numpy.arange() is a useful function for generating arrays of evenly spaced values in NumPy. Its versatility and the ability to handle various data types make it a valuable tool for numerical and scientific computing.