How to Use the Python List index() Method
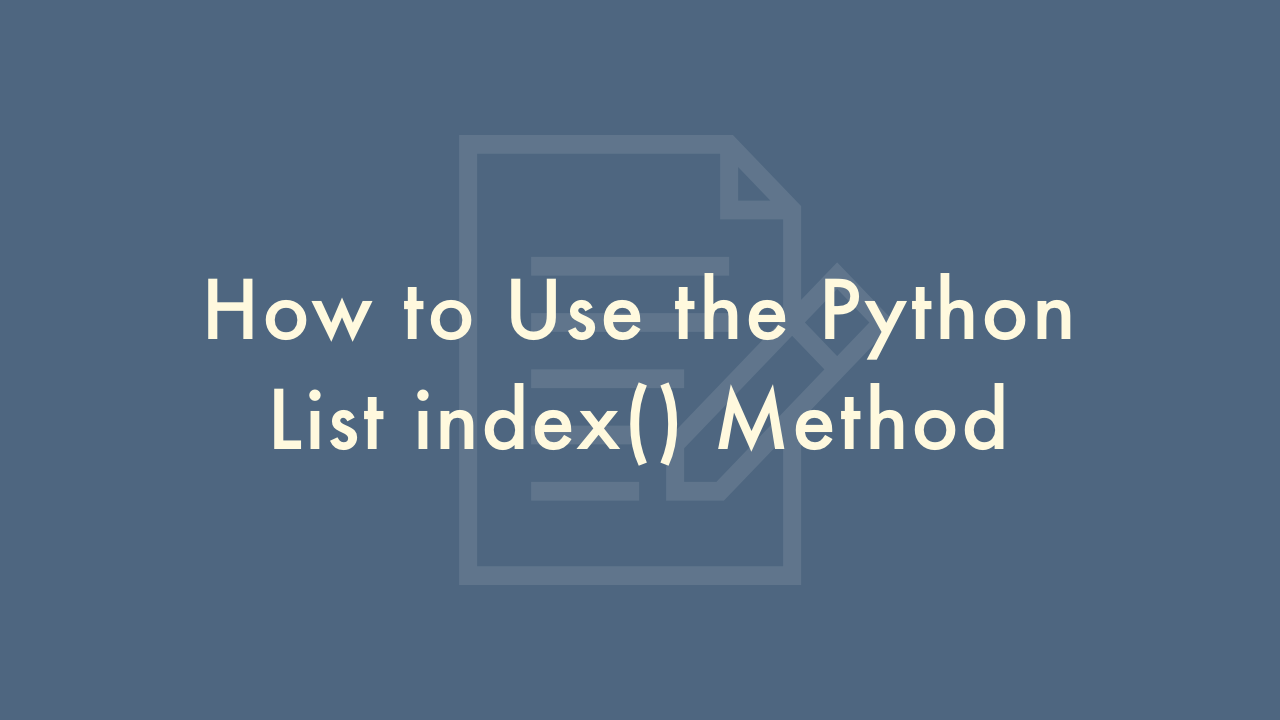
Contents
In this article, you will learn how to use the Python list index() method.
Python List index() Method
The list.index() method in Python is used to find the index of the first occurrence of an item in a list. If the item is not found in the list, a ValueError exception is raised.
Syntax
list.index(item, start, end)
The method takes in three optional arguments: item, which is the item you’re searching for; start, which is the start index from where you want to search (default is 0); and end, which is the end index till where you want to search (default is the end of the list).
Keep in mind that the index() method only returns the index of the first occurrence of the item in the list. If you want to find all the occurrences of an item, you can use a for loop to iterate over the list and check each item.
Example
Here’s an example of how you can use the index() method:
fruits = ['apple', 'banana', 'cherry']
index = fruits.index('banana')
print("The index of 'banana' is: ", index)
# Output: The index of 'banana' is: 1
You can also specify a start and end index to search within a portion of the list:
fruits = ['apple', 'banana', 'cherry', 'banana']
index = fruits.index('banana', 2)
print("The index of 'banana' is: ", index)
# Output: The index of 'banana' is: 3
Here’s an example that demonstrates the use of the start and end arguments:
numbers = [1, 2, 3, 4, 5, 1, 2, 3]
index = numbers.index(2, 3, 6)
print("The index of 2 is: ", index)
# Output: The index of 2 is: 6
The index() method only works on lists, not on other sequence data types like tuples or strings. If you need to find the index of an item in another data type, you can convert it to a list first.
The index() method searches for the item in the list in a linear manner, i.e., it starts from the first item and moves to the next one until it finds the item or reaches the end of the list. So, it has a time complexity of O(n), where n is the number of items in the list.