How to Use the Python List insert() Method
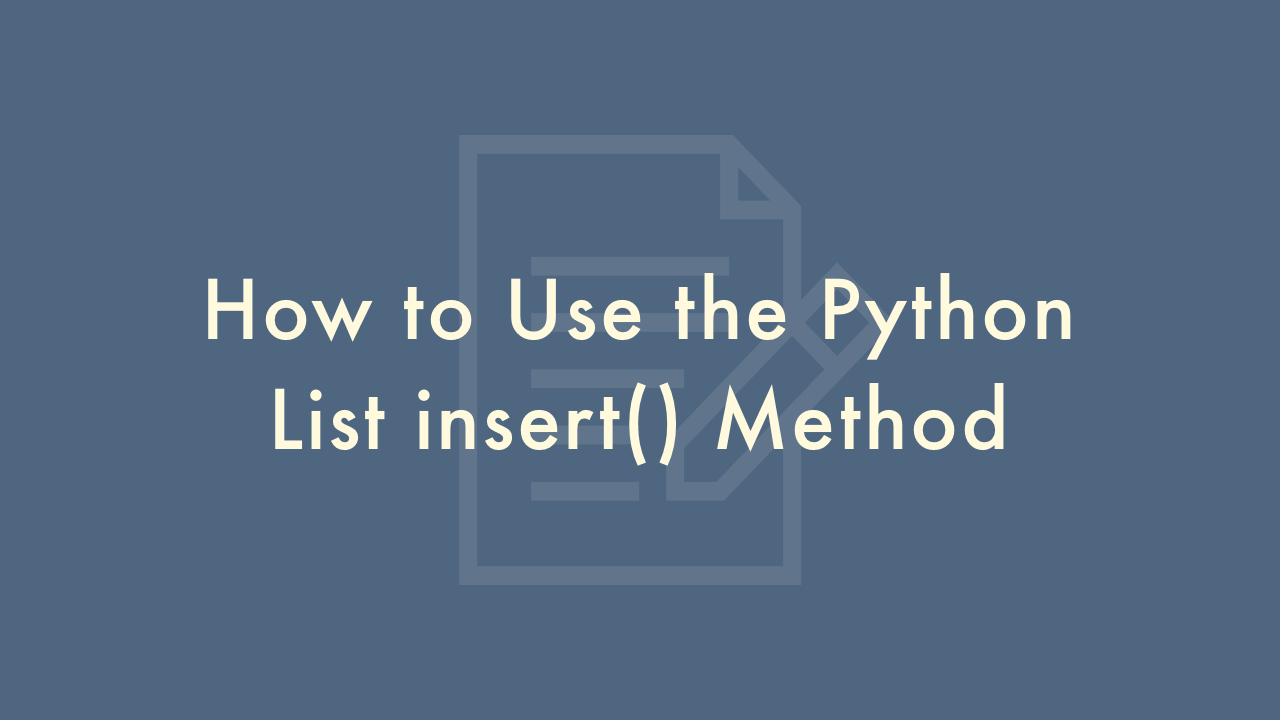
Contents
In this article, you will learn how to use the Python List insert() method.
List insert() Method
The insert() method in Python is used to insert an element at a specified index in a list. It takes two arguments: the first argument is the index at which the element should be inserted, and the second argument is the value that should be inserted. Here is the general syntax:
list_name.insert(index, value)
Here’s an example of how to use the insert() method:
fruits = ['apple', 'banana', 'cherry']
fruits.insert(1, 'orange')
print(fruits)
# Output: ['apple', 'orange', 'banana', 'cherry']
In the above example, the insert() method is used to insert the value ‘orange’ at index 1 in the fruits list. The resulting list after the insert() method is called is [‘apple’, ‘orange’, ‘banana’, ‘cherry’].
It is important to note that when an element is inserted at a particular index, the existing elements at that index and beyond are shifted to the right. Therefore, if you insert an element at an index that is greater than the length of the list, the new element will be added at the end of the list.