How to Use the Python File fileno() Method
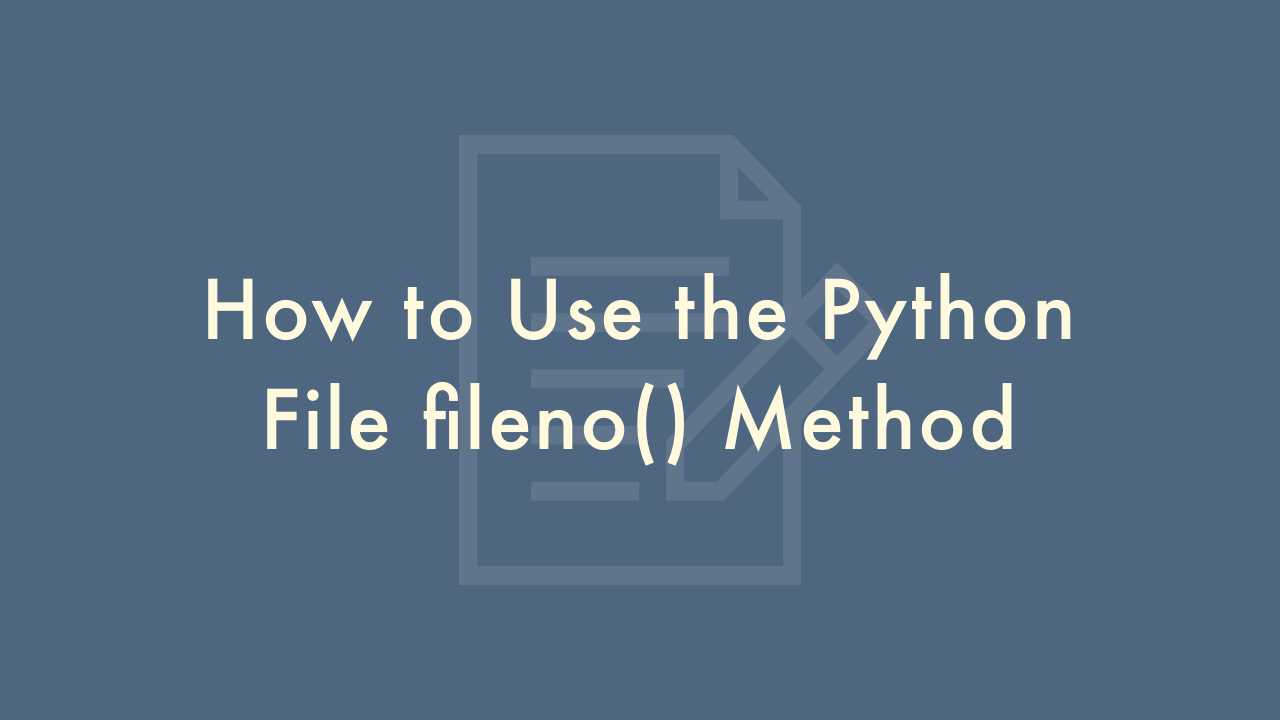
Contents
In this article, you will learn how to use the Python File fileno() method.
Python File fileno() Method
In Python, the fileno() method is used to get the integer file descriptor of a file. The file descriptor is an integer value that represents an open file and is used by the operating system to identify the file.
Here’s an example of how to use the fileno() method:
# Open a file in read mode
file = open('example.txt', 'r')
# Get the file descriptor using fileno()
fd = file.fileno()
# Print the file descriptor
print(fd)
# Close the file
file.close()
In this example, we first open a file named example.txt in read mode using the open() method. We then call the fileno() method on the file object to get the file descriptor, which is stored in the fd variable. Finally, we print the file descriptor and close the file using the close() method.
Here are some additional details about the fileno() method in Python:
- The fileno() method returns an integer file descriptor that can be used with various system calls, such as os.read() and os.write().
- The fileno() method is only available for file-like objects that have an underlying operating system file descriptor, such as files opened with the open() function.
- The file descriptor returned by fileno() is platform-dependent. On Unix-based systems, the file descriptor is a small integer, typically between 0 and 255. On Windows, the file descriptor is a larger integer that can be used with the msvcrt module.
- The file descriptor returned by fileno() is not the same as the file object itself. The file object is a Python object that provides a higher-level interface to the file, while the file descriptor is an integer value that represents a low-level operating system resource.
- It’s important to close the file after you’re done with it to free up system resources. You can do this by calling the close() method on the file object, as shown in the example above.
Here’s an example that demonstrates how to use the fileno() method with the os.read() and os.write() functions:
import os
# Open a file in read mode
file = open('example.txt', 'r')
# Get the file descriptor using fileno()
fd = file.fileno()
# Read the first 10 bytes from the file using os.read()
data = os.read(fd, 10)
# Write the data to stdout using os.write()
os.write(1, data)
# Close the file
file.close()
In this example, we first open a file named example.txt in read mode using the open() method. We then call the fileno() method on the file object to get the file descriptor, which is stored in the fd variable. We use the os.read() function to read the first 10 bytes from the file, passing in the file descriptor as the first argument. We then use the os.write() function to write the data to stdout, which has a file descriptor of 1. Finally, we close the file using the close() method.