How to Get the Conjugate of a Complex Number in Python
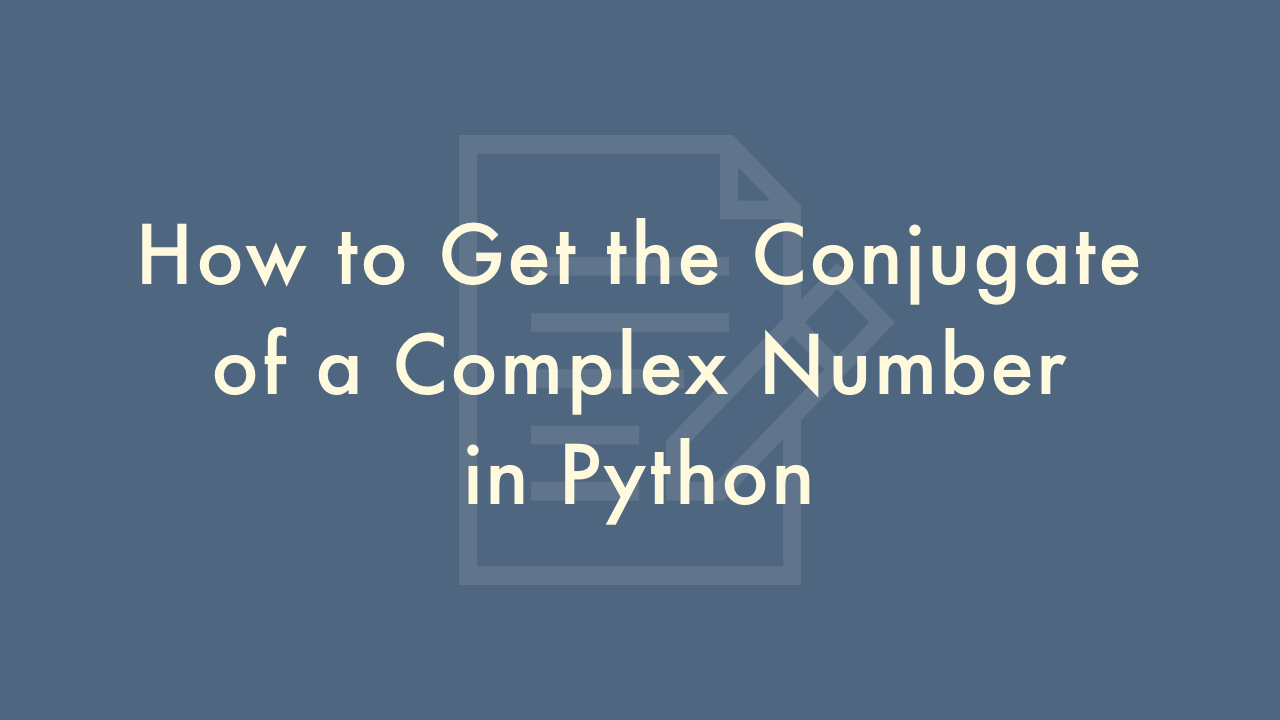
09/16/2021
Contents
In this article, you will learn how to get the conjugate of a complex number in Python.
Get the conjugate of a complex number
In Python, you can use the conjugate() method to get the conjugate of a complex number. Here’s an example:
# Define a complex number
z = 2 + 3j
# Get the conjugate of z
z_conj = z.conjugate()
# Print the original complex number and its conjugate
print("z =", z)
print("Conjugate of z =", z_conj)
# Output:
# z = (2+3j)
# Conjugate of z = (2-3j)
As you can see, the conjugate() method returns a new complex number which is the conjugate of the original complex number. The real part remains the same while the imaginary part changes sign.
Here are some additional things to keep in mind when working with complex numbers in Python:
- Python represents complex numbers using the complex data type. To create a complex number, you can use the syntax a + bj, where a and b are real numbers and j represents the imaginary unit. For example, 2 + 3j represents the complex number 2 + 3i.
- Python provides several built-in functions for working with complex numbers. Some of the most commonly used functions are abs() (returns the absolute value or modulus of a complex number), real() (returns the real part of a complex number), and imag() (returns the imaginary part of a complex number).
-
To perform arithmetic operations on complex numbers, you can use the standard arithmetic operators like +, -, *, and /. For example:
# Define two complex numbers z1 = 2 + 3j z2 = 4 - 2j # Add the two complex numbers z_sum = z1 + z2 # Multiply the two complex numbers z_product = z1 * z2 # Print the results print("Sum of z1 and z2 =", z_sum) print("Product of z1 and z2 =", z_product) # Output: # Sum of z1 and z2 = (6+1j) # Product of z1 and z2 = (14+8j)
-
To access the real and imaginary parts of a complex number separately, you can use the real and imag attributes. For example:
# Define a complex number z = 2 + 3j # Get the real and imaginary parts of z real_part = z.real imag_part = z.imag # Print the results print("Real part of z =", real_part) print("Imaginary part of z =", imag_part) # Output: # Real part of z = 2.0 # Imaginary part of z = 3.0