How to Generate Pseudo-Random Numbers in Python
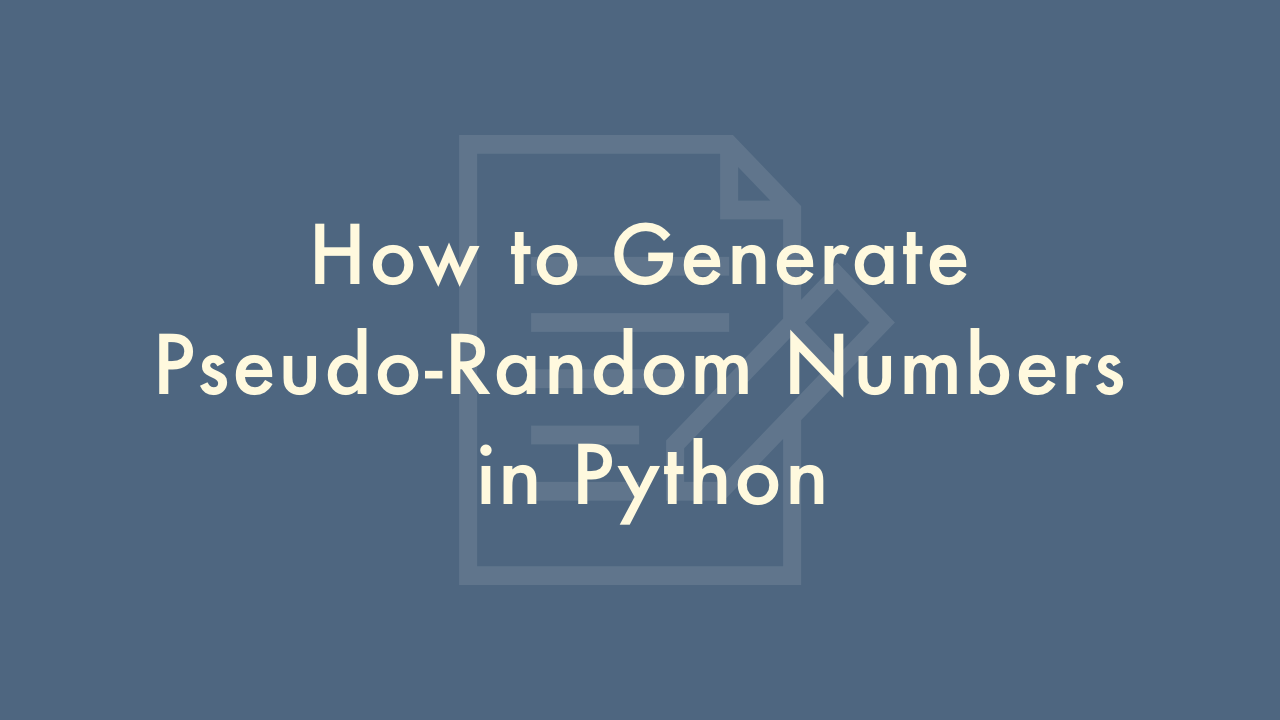
Contents
In this article, you will learn how to generate pseudo-random numbers in Python.
Generate pseudo-random numbers
Python has a built-in module called random that can be used to generate pseudo-random numbers. Here are a few examples:
Generate a random float between 0 and 1:
import random
num = random.random()
print(num)
Generate a random integer between a specified range:
import random
num = random.randint(1, 100)
print(num)
Shuffle a list in a random order:
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
Generate a random element from a list:
import random
my_list = [1, 2, 3, 4, 5]
num = random.choice(my_list)
print(num)
Here’s some additional information about generating pseudo-random numbers in Python using the random module:
- random.random() generates a pseudo-random float between 0 and 1, including 0 but not including 1.
- random.randint(a, b) generates a pseudo-random integer between a and b, inclusive of both a and b.
- random.uniform(a, b) generates a pseudo-random float between a and b, including both a and b.
- random.randrange(start, stop=None, step=1) generates a pseudo-random integer from the range specified by start, stop, and step. The start argument is required, and stop and step are optional. If stop is not specified, it defaults to start + 1.
- random.choice(sequence) generates a pseudo-random element from the given sequence.
- random.shuffle(sequence) shuffles the elements in a list in a pseudo-random order.
- random.sample(sequence, k) returns a new list with k elements randomly sampled from the given sequence.
It’s worth noting that the random module’s pseudo-random number generation can be affected by the seed value used to initialize the random number generator. By default, the seed value is based on the system time, so the sequence of random numbers generated by a script may be different each time it is run. However, you can set the seed value manually using the random.seed() function to ensure that the same sequence of random numbers is generated each time the script is run.
Also, for more advanced pseudo-random number generation needs, Python provides a separate module called numpy.random that provides more extensive functionality, including various probability distributions and statistical functions.