How to Use Python Dictionaries
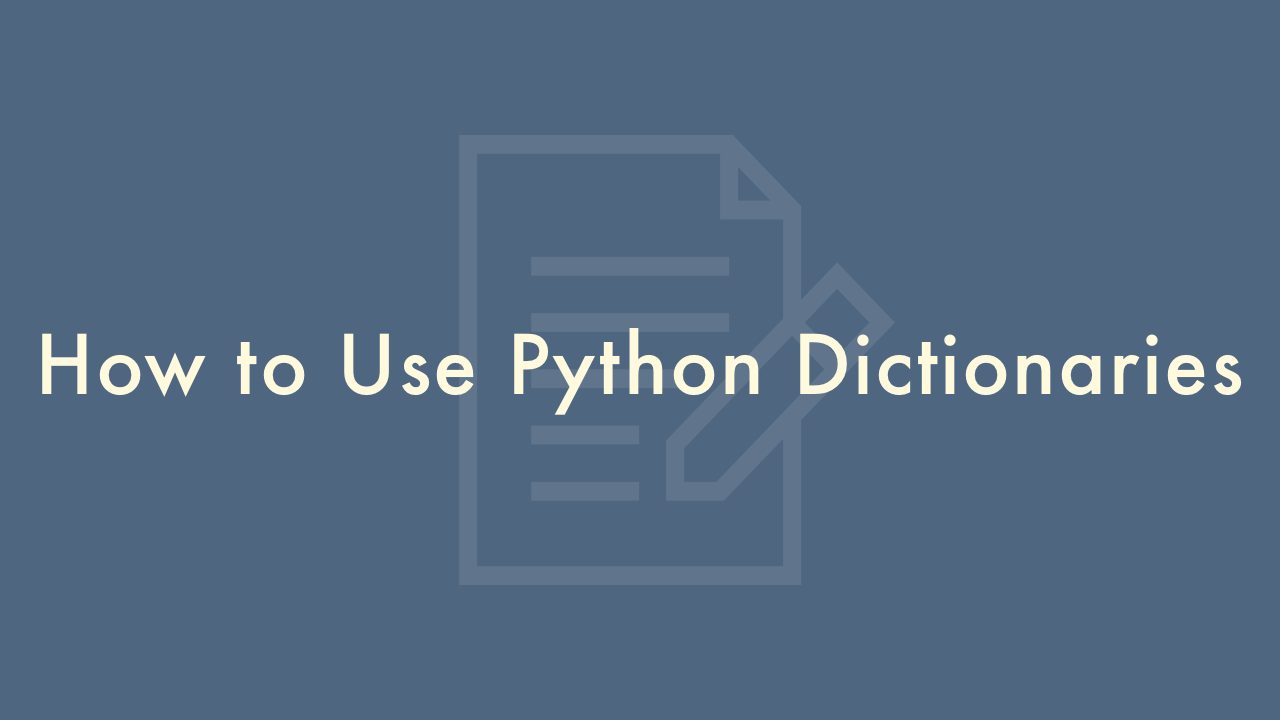
Contents
In this article, you will learn how to use Python dictionaries.
Python Dictionaries
Python dictionaries are key-value pairs that store values in an unordered and mutable way. You can use dictionaries to store and retrieve data in a flexible way.
Here are some common ways to use dictionaries in Python:
Creating a dictionary
You can create a dictionary using curly braces {} or the dict() constructor.
For example:
# Using curly braces
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Using dict() constructor
my_dict = dict(key1='value1', key2='value2')
Accessing values
You can access values stored in a dictionary using the square bracket syntax and the key name.
For example:
my_dict = {'key1': 'value1', 'key2': 'value2'}
print(my_dict['key1']) # Output: value1
Adding/Updating values
You can add a new key-value pair to the dictionary or update the value of an existing key using the square bracket syntax.
For example:
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Adding a new key-value pair
my_dict['key3'] = 'value3'
# Updating the value of an existing key
my_dict['key2'] = 'new_value2'
Deleting values
You can delete a key-value pair from a dictionary using the del statement or the pop method.
For example:
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Using del statement
del my_dict['key1']
# Using pop method
my_dict.pop('key2')
Looping through a dictionary
You can loop through the keys, values, or both of a dictionary using the items method.
For example:
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Looping through keys
for key in my_dict.keys():
print(key)
# Looping through values
for value in my_dict.values():
print(value)
# Looping through both keys and values
for key, value in my_dict.items():
print(key, value)
These are just a few of the ways you can use dictionaries in Python. There are many more methods and features available to make dictionaries even more flexible and useful.