How to Use the Python getattr() Function
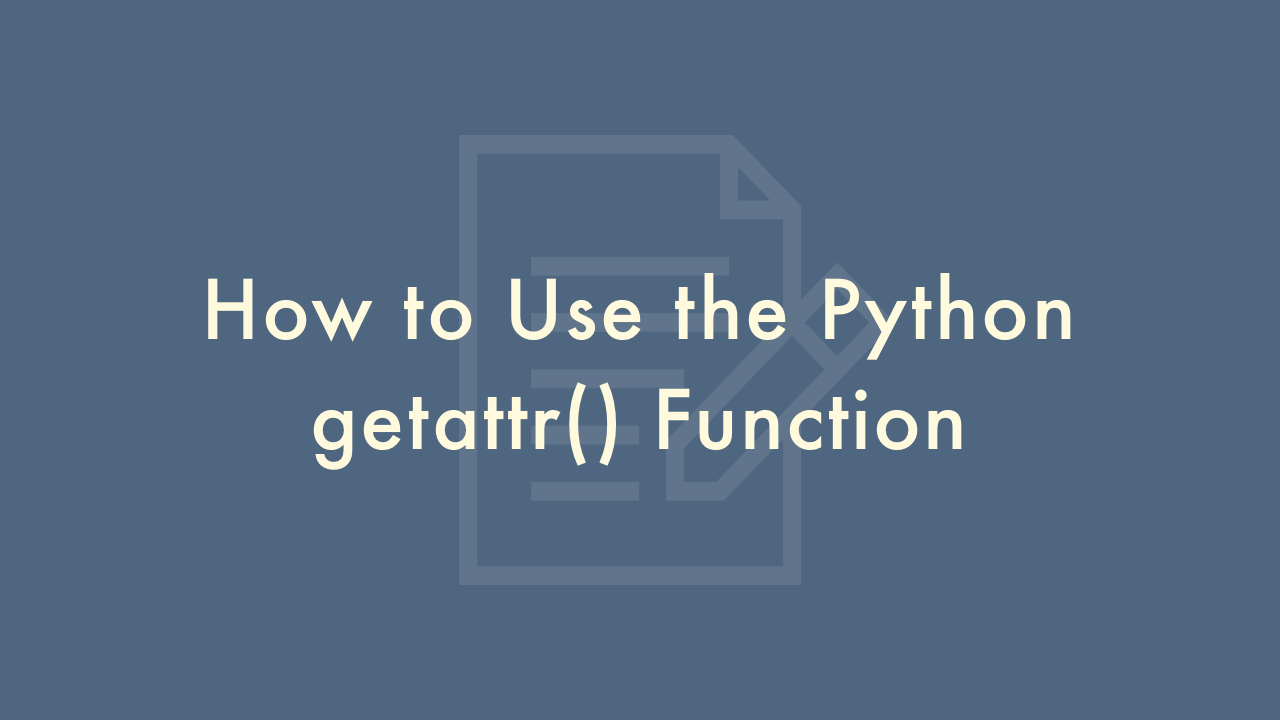
09/18/2021
Contents
In this article, you will learn how to use the Python getattr() function.
Python getattr() Function
The Python getattr() function is a built-in function that returns the value of a named attribute of an object. Here’s how you can use the getattr() function in Python:
Syntax
getattr(object, name[, default])
Parameters
object
: This is the object whose attribute you want to access.name
: This is the name of the attribute you want to access.default
(optional): This is the default value to be returned if the named attribute is not found.
Example
Here are some examples of using getattr():
Accessing a class attribute
class MyClass:
x = 10
def __init__(self):
self.y = 20
obj = MyClass()
print(getattr(MyClass, 'x')) # Output: 10
Accessing an instance attribute
class MyClass:
x = 10
def __init__(self):
self.y = 20
obj = MyClass()
print(getattr(obj, 'y')) # Output: 20
Specifying a default value
class MyClass:
x = 10
def __init__(self):
self.y = 20
obj = MyClass()
print(getattr(obj, 'z', 'Not Found')) # Output: Not Found
In this example, the named attribute z does not exist in the obj object. Therefore, the getattr() function returns the default value ‘Not Found’.