How to Transform List Elements with the Python map() Function
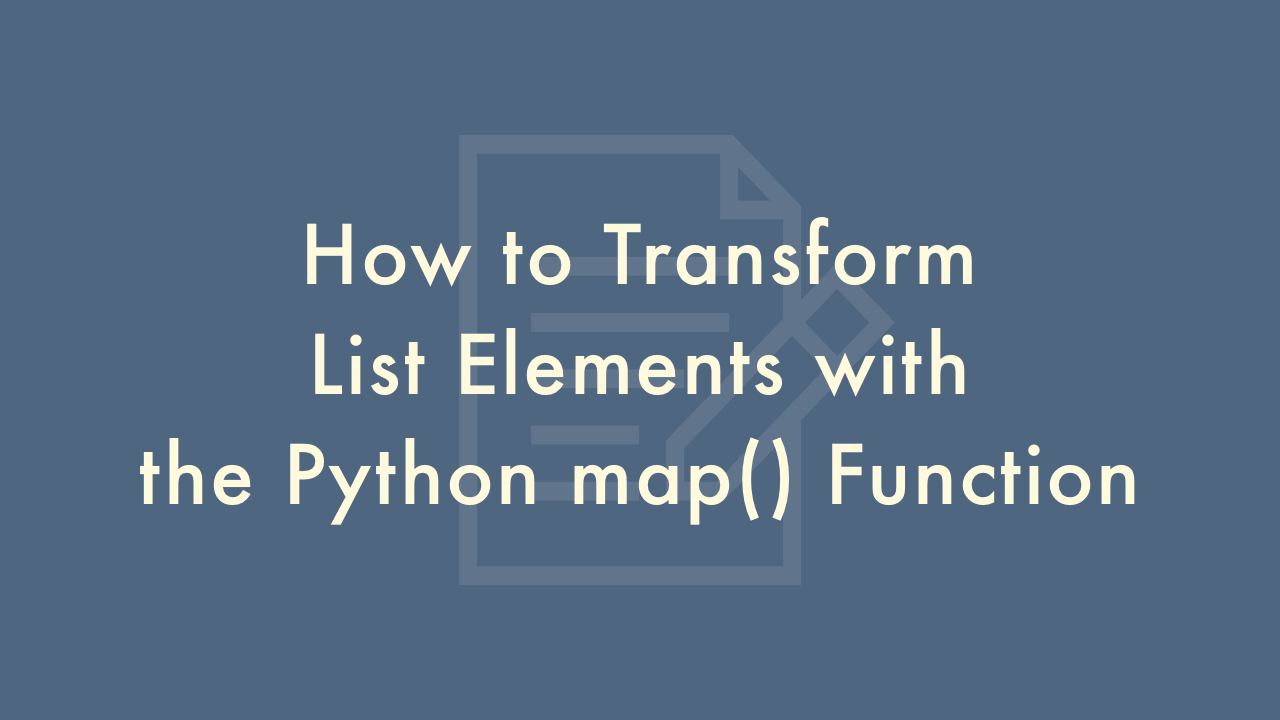
Contents
In this article, you will learn how to transform list elements with the Python map() function.
How to Transform List Elements
The map() function in Python is a built-in function that allows you to apply a function to each element of an iterable (e.g., list, tuple, etc.) and returns a new iterable with the results.
To transform list elements with the map() function in Python, you can follow these steps:
-
Define a function that performs the transformation you want. This function should take an element of the iterable as an input and return the transformed element.
For example, let’s say you have a list of integers and you want to square each element. You can define a function called square that takes an integer as an input and returns the square of that integer:
def square(x): return x**2
-
Use the map() function to apply the function to each element of the list. The map() function takes two arguments: the function to apply and the iterable to apply it to.
For example, you can use the map() function to apply the square() function to each element of a list of integers:
my_list = [1, 2, 3, 4, 5] squared_list = list(map(square, my_list))
-
The map() function returns an iterator, so you need to convert it to a list using the list() function.
In the above example, the map() function returns an iterator with the squared values of each element in my_list. We then use the list() function to convert the iterator into a list and store the result in squared_list.
The resulting squared_list will be [1, 4, 9, 16, 25], which is the original list with each element squared.