How to Use Pandas DataFrame sort_values() Function in Python
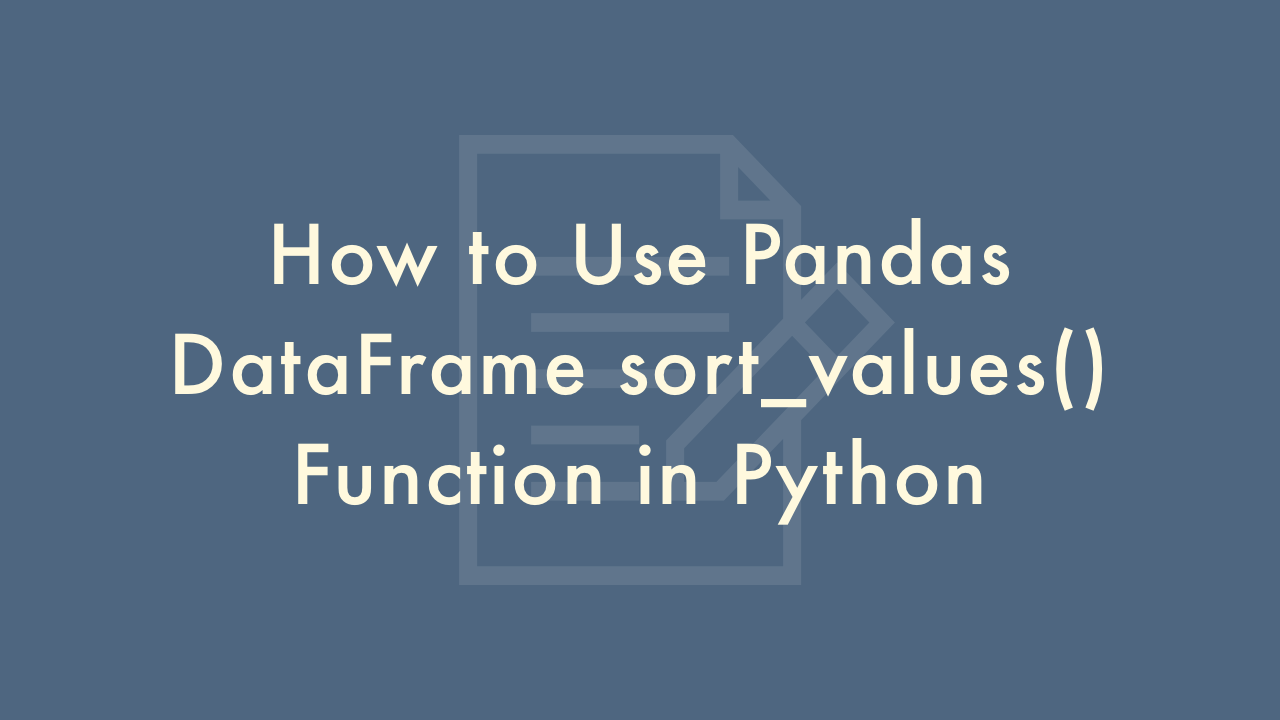
Contents
In this article, you will learn how to use Pandas DataFrame sort_values() function in Python.
Pandas DataFrame sort_values() Function
Pandas DataFrame sort_values() function is used to sort a DataFrame by one or more columns. Here’s how to use the function in Python:
Import the pandas library:
import pandas as pd
Create a DataFrame:
df = pd.DataFrame({'Name': ['John', 'Mary', 'James', 'Sara'],
'Age': [25, 30, 27, 22],
'Salary': [50000, 60000, 55000, 45000]})
The DataFrame df has three columns: Name, Age, and Salary.
Sort the DataFrame by a single column:
sorted_df = df.sort_values('Name')
This will sort the DataFrame by the ‘Name’ column in ascending order.
To sort the DataFrame in descending order, use the ascending parameter:
sorted_df = df.sort_values('Name', ascending=False)
Sort the DataFrame by multiple columns:
To sort the DataFrame by multiple columns, pass a list of column names to the sort_values() function:
sorted_df = df.sort_values(['Age', 'Salary'])
This will first sort the DataFrame by the ‘Age’ column in ascending order, and then by the ‘Salary’ column in ascending order.
To sort the DataFrame in descending order by one or more columns, use the ascending parameter as before:
sorted_df = df.sort_values(['Age', 'Salary'], ascending=False)
This will sort the DataFrame by the ‘Age’ column in descending order, and then by the ‘Salary’ column in descending order.
View the sorted DataFrame:
print(sorted_df)
This will print the sorted DataFrame.
Here are some more details on how to use the sort_values() function in Pandas DataFrame:
Syntax:
DataFrame.sort_values(by, axis=0, ascending=True, inplace=False, ignore_index=False, key=None)
Parameters:
by
: The column(s) to sort by. This can be a single column name (string), a list of column names (list), or a Series.axis
: The axis to sort along. 0 for rows, 1 for columns. The default value is 0.ascending
: Whether to sort in ascending or descending order. The default value is True (ascending order).inplace
: Whether to modify the original DataFrame or return a new sorted DataFrame. The default value is False, which means a new DataFrame is returned.ignore_index
: Whether to reset the index of the returned DataFrame. The default value is False, which means the original index is preserved.key
: A function to customize the sort order. This is useful when sorting by a computed value, such as the absolute value of a column.
Return:
A sorted DataFrame with the same columns and index as the original DataFrame.
Notes:
- If the DataFrame contains NaN values, they will be placed at the end by default when sorting in ascending order, and at the beginning when sorting in descending order.
- If multiple columns are used to sort the DataFrame, the sorting is performed in order of the columns listed.
Example:
Here’s an example of using the key parameter to sort a DataFrame by the absolute value of a column:
import pandas as pd
df = pd.DataFrame({'A': [-3, 2, -1, 4, 0],
'B': [5, 2, 1, 6, 4]})
sorted_df = df.sort_values(by='A', key=lambda x: abs(x))
print(sorted_df)
Output:
A B
2 -1 1
4 0 4
1 2 2
3 4 6
0 -3 5
The key function takes each value in the ‘A’ column, computes its absolute value, and returns that as the sorting key. This sorts the DataFrame by the absolute values of the ‘A’ column, while preserving the original values in ‘A’.