How to Use the Python String format() Method
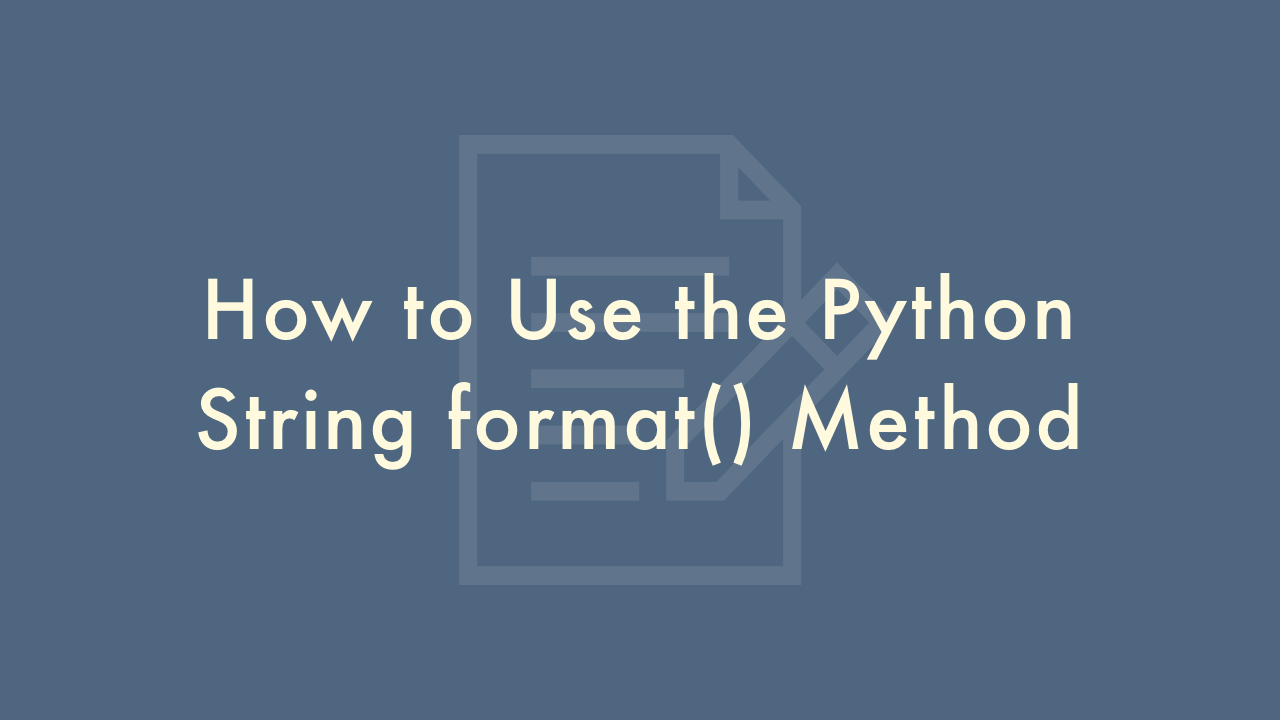
Contents
In this article, you will learn how to use the Python string format() method.
Python String format() Method
The format() method is a built-in function in Python that is used to format strings. It allows you to insert values into a string in a way that is flexible, powerful and easy to use. Here is how to use the format() method in Python:
Basic string formatting:
You can use the format() method by calling it on a string and passing the value you want to insert inside curly braces {}. For example:
name = "John"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
The output of this code will be:
My name is John and I am 25 years old.
Positional arguments:
You can also use positional arguments to specify the order in which the values should be inserted into the string. For example:
name = "John"
age = 25
print("My name is {0} and I am {1} years old.".format(name, age))
The output of this code will be the same as the previous example.
Named arguments:
You can also use named arguments to specify the values to be inserted into the string. For example:
name = "John"
age = 25
print("My name is {name} and I am {age} years old.".format(name=name, age=age))
The output of this code will be the same as the previous examples.
Formatting numbers:
You can also format numbers using the format() method. For example:
number = 3.14159265359
print("The value of pi is approximately {:.2f}.".format(number))
The output of this code will be:
The value of pi is approximately 3.14.
Padding strings:
You can also pad a string with a specified character and width using the format() method. For example:
string = "hello"
print("'{:<10}'".format(string))
print("'{:>10}'".format(string))
The output of this code will be:
'hello '
' hello'
These are just a few examples of how to use the format() method in Python. You can find more information about string formatting in the Python documentation.