How to Access Nested Dictionary in Python
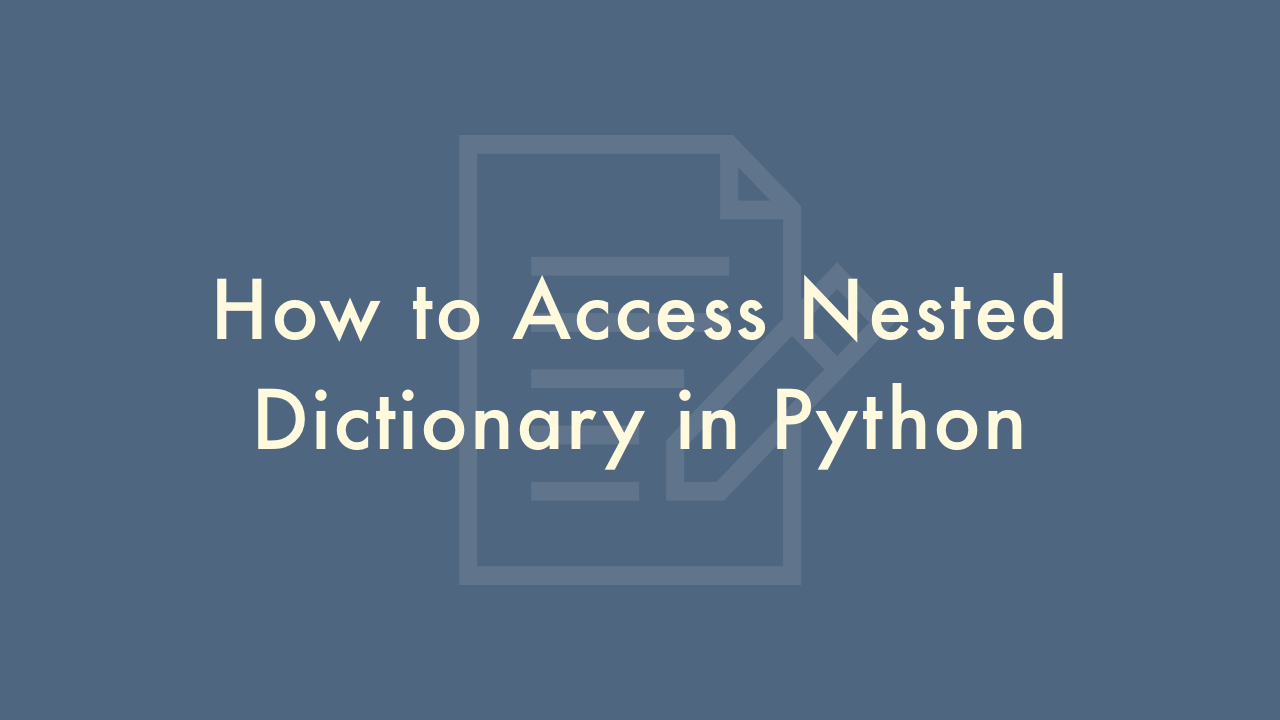
Contents
In this article, you will learn how to access nested dictionary in Python.
Access Nested Dictionary
In Python, a nested dictionary is a dictionary within another dictionary. To access values in a nested dictionary, you can use square bracket notation multiple times to access the keys of the nested dictionaries.
Here’s an example:
# Nested dictionary
nested_dict = {
"dict1": {
"key1": "value1",
"key2": "value2"
},
"dict2": {
"key3": "value3",
"key4": "value4"
}
}
# Accessing a value in a nested dictionary
value = nested_dict["dict1"]["key1"]
print(value) # Output: "value1"
In the above example, the first set of square brackets accesses the “dict1” key, and the second set of square brackets accesses the “key1” key within the nested dictionary.
If you’re not sure about the structure of the nested dictionary, you can use the get method to access values. The get method returns None if the key is not found, instead of raising a KeyError:
# Using get method to access a value in a nested dictionary
value = nested_dict.get("dict1", {}).get("key1")
print(value) # Output: "value1"
You can also use a combination of both techniques, using square brackets to access the top-level keys and the get method to access the nested keys, to make your code more robust to missing keys.