How to Get the Day of the Week in Python
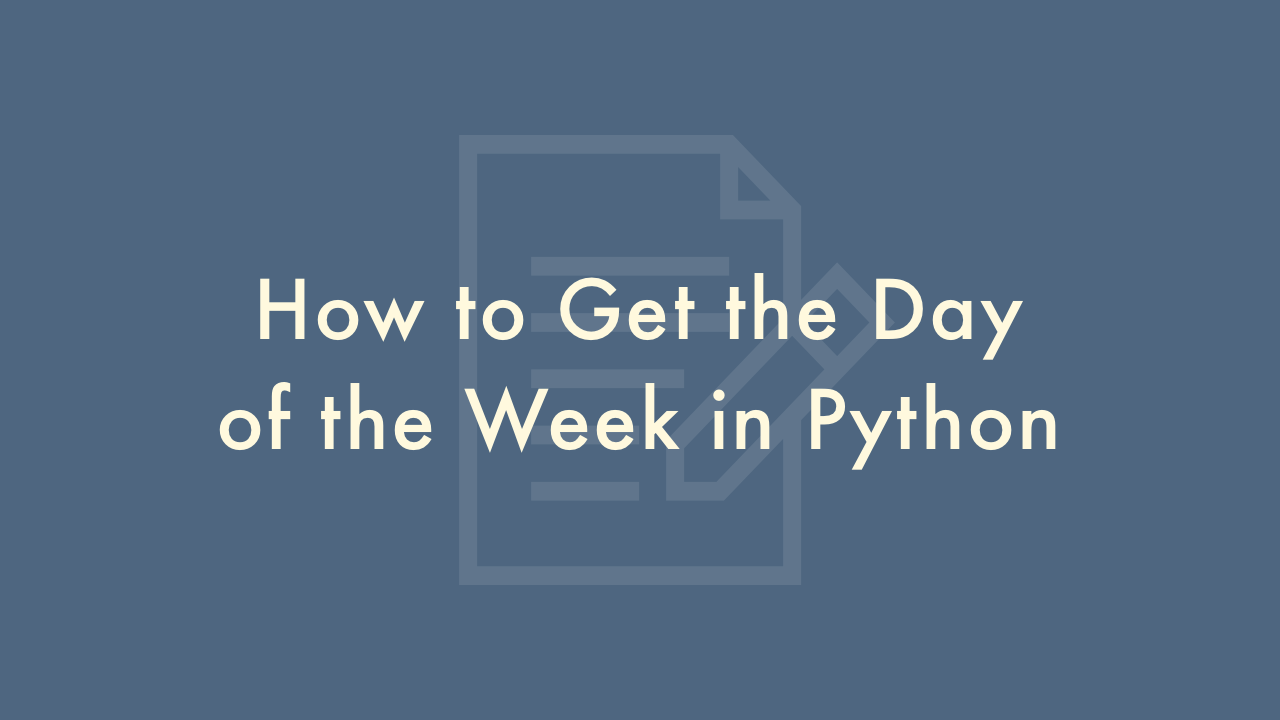
09/15/2021
Contents
In this article, you will learn how to get the day of the week in Python.
Get the Day of the Week
In Python, you can get the day of the week for a given date using the built-in datetime module. Here’s an example code snippet to get you started:
import datetime
# create a datetime object for a given date
date = datetime.date(2023, 2, 21)
# get the day of the week as an integer (Monday is 0 and Sunday is 6)
day_of_week = date.weekday()
# get the name of the day of the week
day_name = date.strftime("%A")
# print the results
print(day_of_week) # output: 0 (since Feb 21, 2023 is a Monday)
print(day_name) # output: Monday
In this example, we create a datetime object representing February 21, 2023, and then use the weekday() method to get the day of the week as an integer (with Monday as 0 and Sunday as 6). We also use the strftime() method to get the name of the day of the week in a more readable format.