How to Send Email in Python
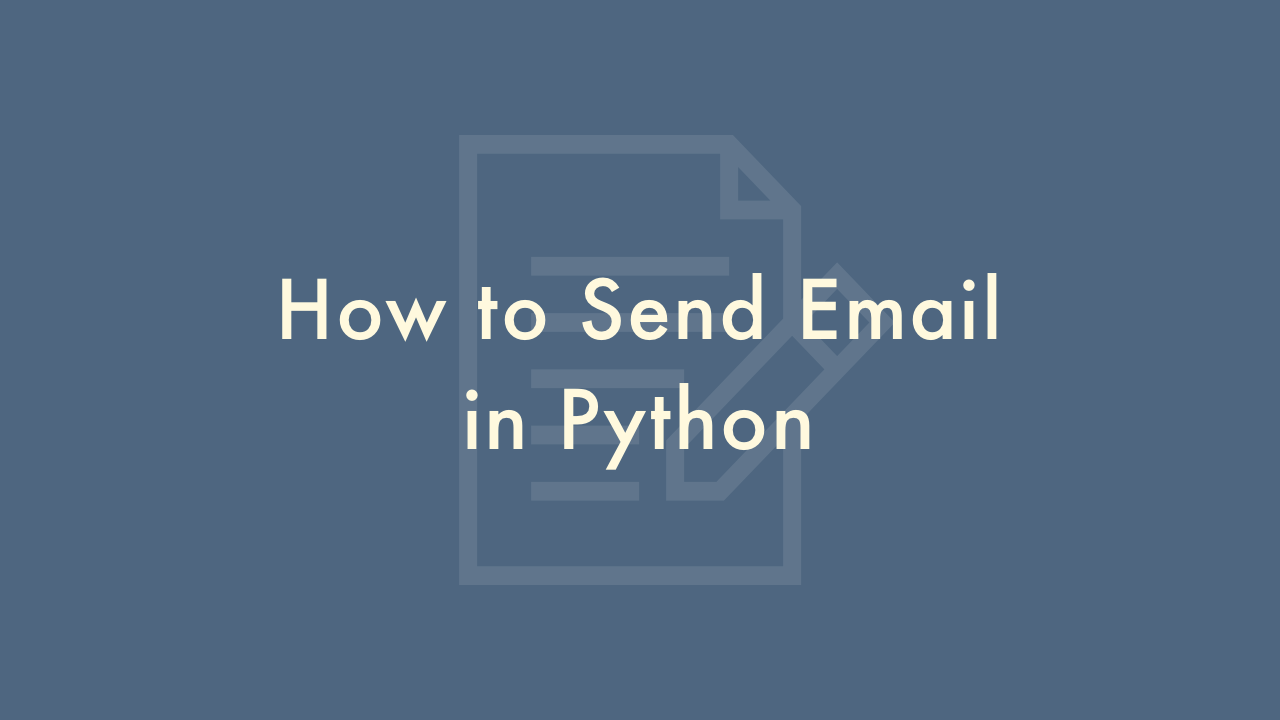
09/14/2021
Contents
In this article, you will learn how to dend email in Python.
How to Send Email in Python
You can send emails in Python using the built-in smtplib module, which provides a simple way to send email messages using the Simple Mail Transfer Protocol (SMTP).
Here’s an example code snippet that demonstrates how to send an email in Python:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# Define the sender's and recipient's email addresses
sender = 'sender@example.com'
recipient = 'recipient@example.com'
# Create a message object
msg = MIMEMultipart()
msg['From'] = sender
msg['To'] = recipient
msg['Subject'] = 'Test Email'
# Add the message body
body = 'This is a test email sent from Python'
msg.attach(MIMEText(body, 'plain'))
# Connect to the SMTP server
smtp_server = smtplib.SMTP('smtp.example.com', 587)
smtp_server.starttls()
# Login to the SMTP server (if needed)
smtp_server.login('username', 'password')
# Send the email
smtp_server.sendmail(sender, recipient, msg.as_string())
# Close the SMTP server connection
smtp_server.quit()
This code sends a plain text email with a subject line and a message body. You can modify the MIMEText constructor to create HTML or other types of messages.
Make sure to replace the sender, recipient, smtp_server, username, and password values with your own email and SMTP server details.