How to Get Variable Name as String in Python
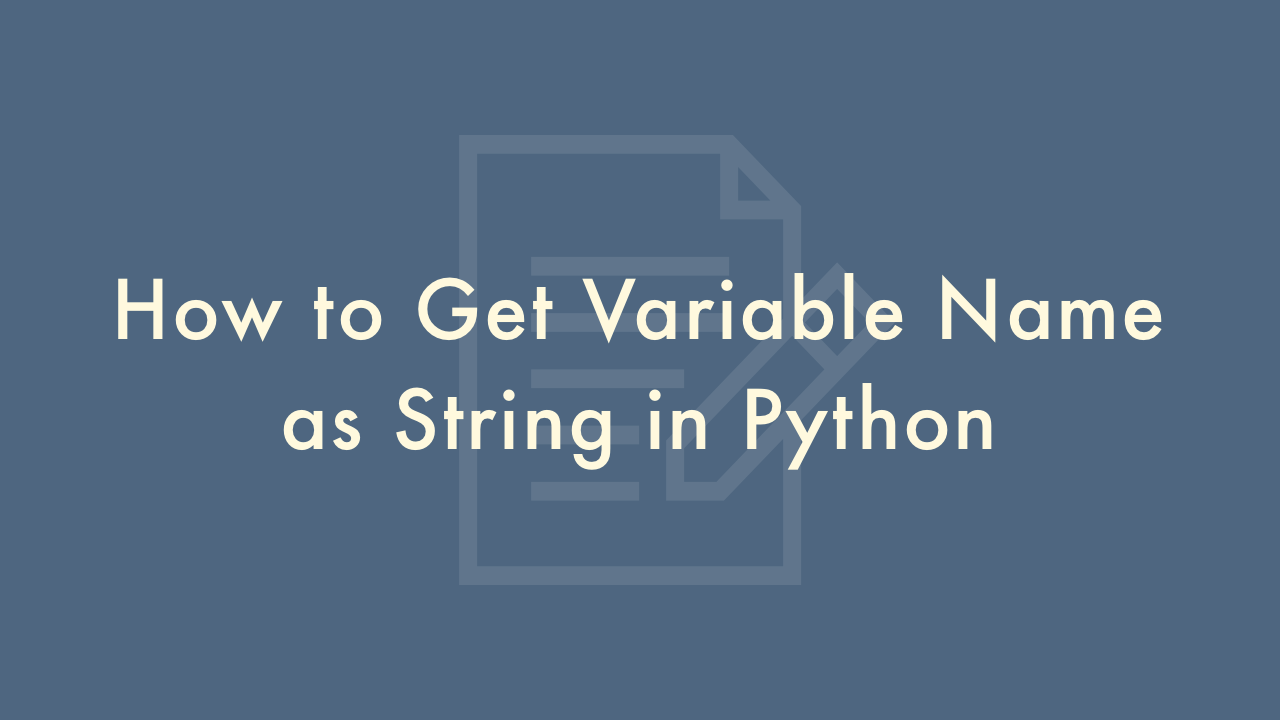
Contents
In this article, you will learn how to get variable name as string in Python.
Get variable name as string
In Python, variable names are just references to the memory location where the value of the variable is stored. Therefore, it is not possible to directly get the name of a variable in Python. However, there are some ways to get the name of a variable in Python code:
Using the locals() function:
The locals() function returns a dictionary containing the local variable names and their corresponding values in the current scope.
x = 5
for name in locals():
if locals()[name] is x:
print(name)
Using the globals() function:
The globals() function returns a dictionary containing the global variable names and their values.
x = 5
for name in globals():
if globals()[name] is x:
print(name)
Using the inspect module:
The inspect module provides more advanced ways of accessing the runtime context and introspecting the code, including getting the current frame and code context. This can be used to extract the variable name, although it can be more complex and not as reliable.
import inspect
def func(x):
print(inspect.getframeinfo(inspect.currentframe()).code_context[0].strip())
func(x)
Note that these methods will only work if the variable is in the current scope, and will not work for variables inside functions or classes.