How to Use the NumPy argmax() Function in Python
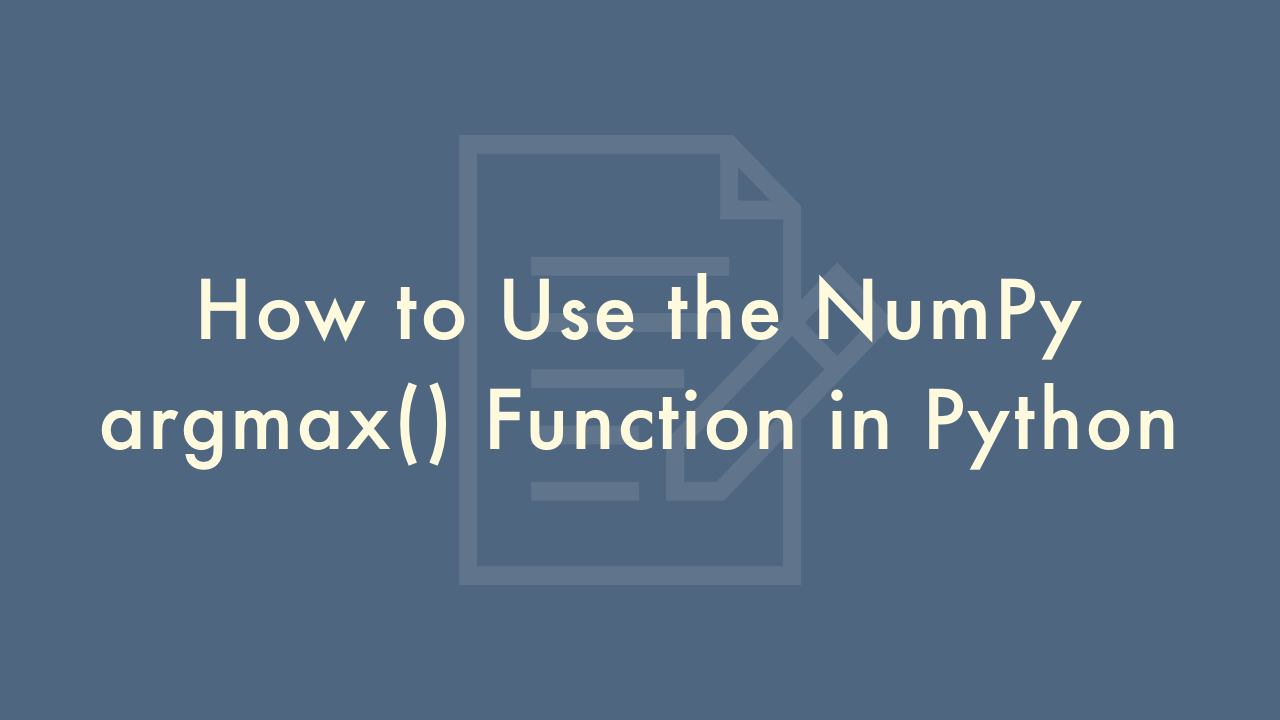
Contents
In this article, you will learn how to use the NumPy argmax() function in Python.
NumPy argmax() Function
The argmax() function in NumPy is used to return the indices of the maximum value in an array. Here is how you can use it:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
index_of_max_value = np.argmax(a)
print(index_of_max_value)
# Output: 4
In this example, the argmax() function returns the index of the maximum value in the a array, which is 4. This means that the maximum value in the array is located at the fourth position, with a value of 5.
Note that argmax() only returns the first occurrence of the maximum value. If there are multiple occurrences of the maximum value in the array, only the first one will be returned.
The argmax() function can be used along multiple axes in a multi-dimensional array. For example:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
index_of_max_value = np.argmax(a, axis=0)
print(index_of_max_value)
# Output: array([2, 2, 2])
In this example, the axis parameter is set to 0, which means that the maximum values are computed along the rows. The result is an array with the indices of the maximum values in each column. So the maximum value in the first column is 7 (located at index 2), the maximum value in the second column is 8 (located at index 2), and the maximum value in the third column is 9 (located at index 2).
You can also compute the maximum along the columns by setting the axis parameter to 1:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
index_of_max_value = np.argmax(a, axis=1)
print(index_of_max_value)
# Output: array([2, 2, 2])
In this case, the result is an array with the indices of the maximum values in each row. So the maximum value in the first row is 3 (located at index 2), the maximum value in the second row is 6 (located at index 2), and the maximum value in the third row is 9 (located at index 2).