How to Use the Python type() Function
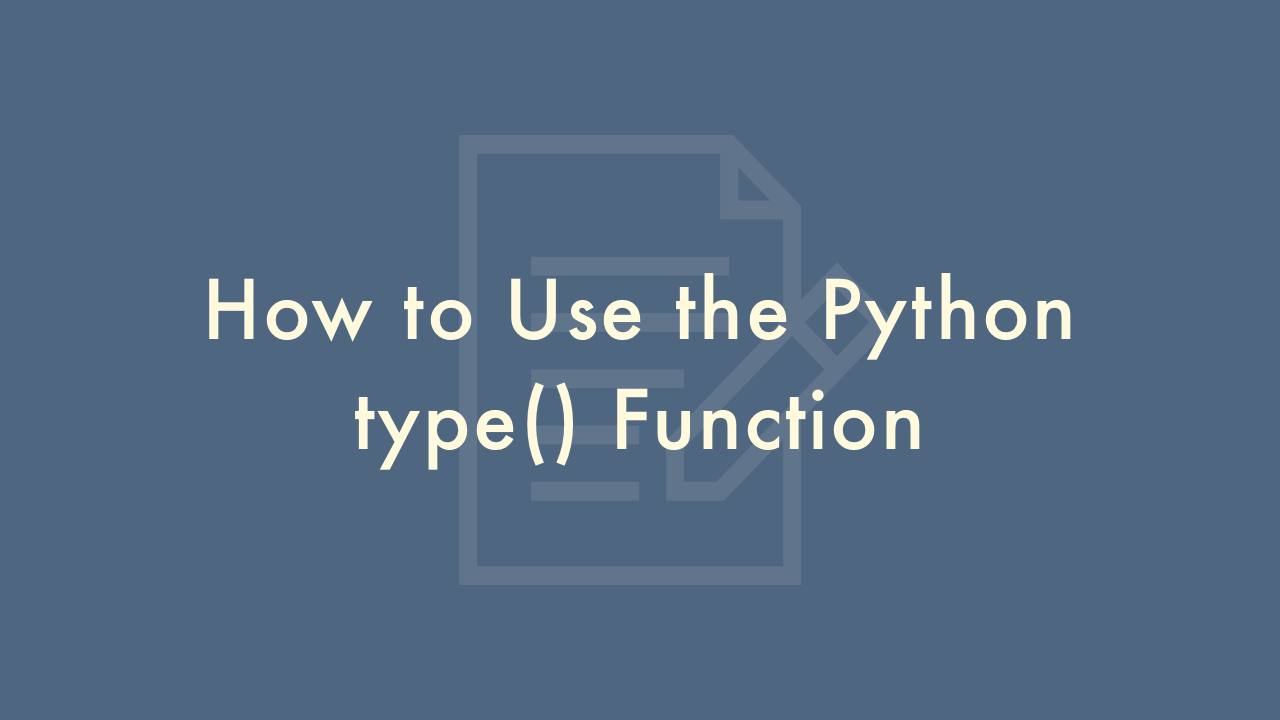
Contents
In this article, you will learn how to use the Python type() function.
Python type() Function
The type() function is a built-in function in Python that allows you to check the type of a variable or an object. Here are some ways you can use the type() function in your Python code:
Checking the type of a variable:
x = 42
print(type(x)) # <class 'int'>
In this example, type(x) returns <class ‘int’>, indicating that x is an integer.
Checking the type of an object:
lst = [1, 2, 3]
print(type(lst)) # <class 'list'>
In this example, type(lst) returns <class ‘list’>, indicating that lst is a list object.
Checking the type of a function:
def add_numbers(a, b):
return a + b
print(type(add_numbers)) # <class 'function'>
In this example, type(add_numbers) returns <class ‘function’>, indicating that add_numbers is a function.
Checking the type of a class:
class Person:
def __init__(self, name):
self.name = name
p = Person("Alice")
print(type(p)) # <class '__main__.Person'>
In this example, type(p) returns <class ‘__main__.Person’>, indicating that p is an instance of the Person class.
Overall, the type() function is a useful tool for checking the type of variables, objects, functions, and classes in your Python code.