How to Use the React useCallback Hook
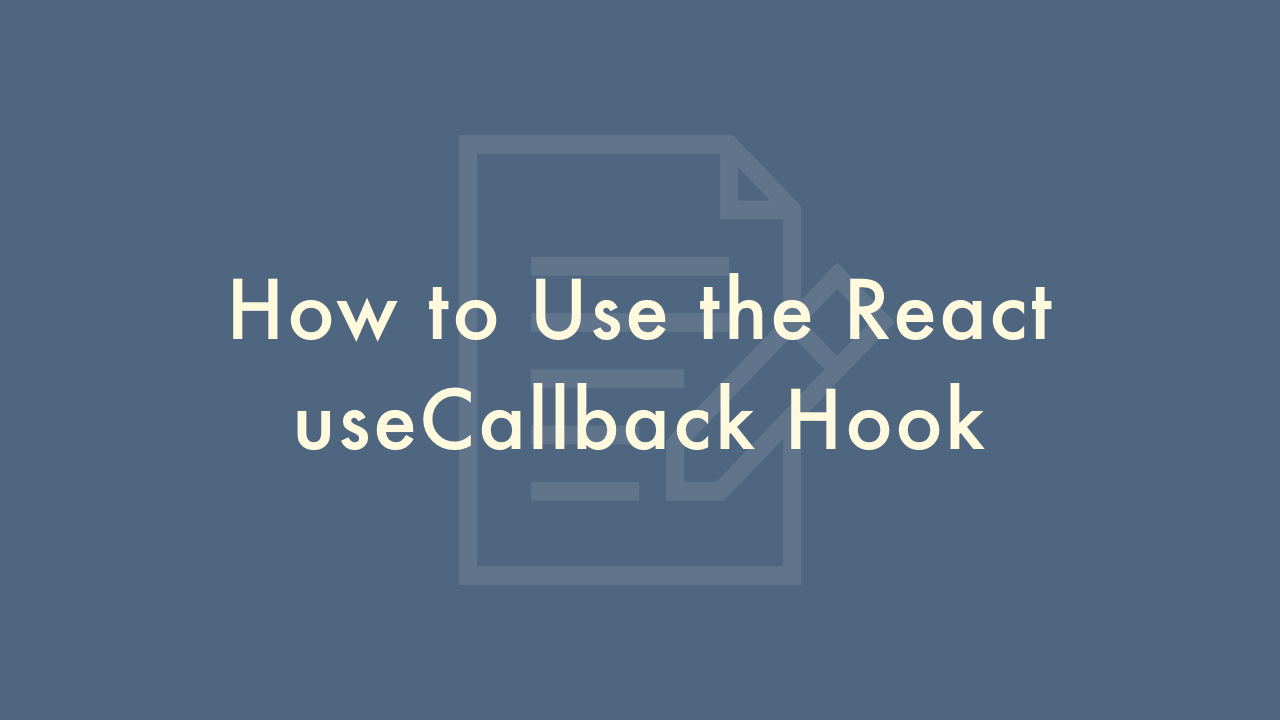
Contents
In this article, you will learn how to use the React useCallback hook.
Using the useCallback hook
React’s useCallback hook is a built-in function that can be used to optimize the performance of functional components that rely on callback functions, such as event handlers.
The useCallback hook memoizes a function and returns a memoized version of it. Memoization is the process of caching the result of a function call and reusing it when the same input is provided again. This can improve performance by reducing the number of times a function needs to be recreated.
Here’s how to use the useCallback hook in a React functional component:
Import the useCallback hook from the react library:
import React, { useCallback } from 'react';
Declare the callback function that you want to memoize:
const myCallback = (param) => {
// function code here
};
Use the useCallback hook to memoize the function:
const memoizedCallback = useCallback(myCallback, [param1, param2]);
The first argument to useCallback is the function that you want to memoize, and the second argument is an array of dependencies. The dependencies are used to determine whether to create a new memoized function or reuse an existing one.
If any of the dependencies change, the useCallback hook will create a new memoized function. If the dependencies don’t change, the previously memoized function will be reused.
Use the memoized callback function in your component:
return (
<button onClick={memoizedCallback}>Click me</button>
);
By using the memoized callback function, you can avoid unnecessary re-renders of your component.
Here are some tips for using the useCallback hook effectively:
- Only use useCallback when you need to optimize performance. If the callback function is simple and doesn’t have any dependencies, there’s no need to memoize it.
- Be careful when using useCallback with dependencies. If you include too many dependencies or dependencies that change frequently, you may defeat the purpose of memoization and end up with worse performance.
- Don’t rely solely on useCallback for performance optimization. There are other techniques, such as memoization and code splitting, that can also improve performance.