How to Use the React componentDidUpdate() Method
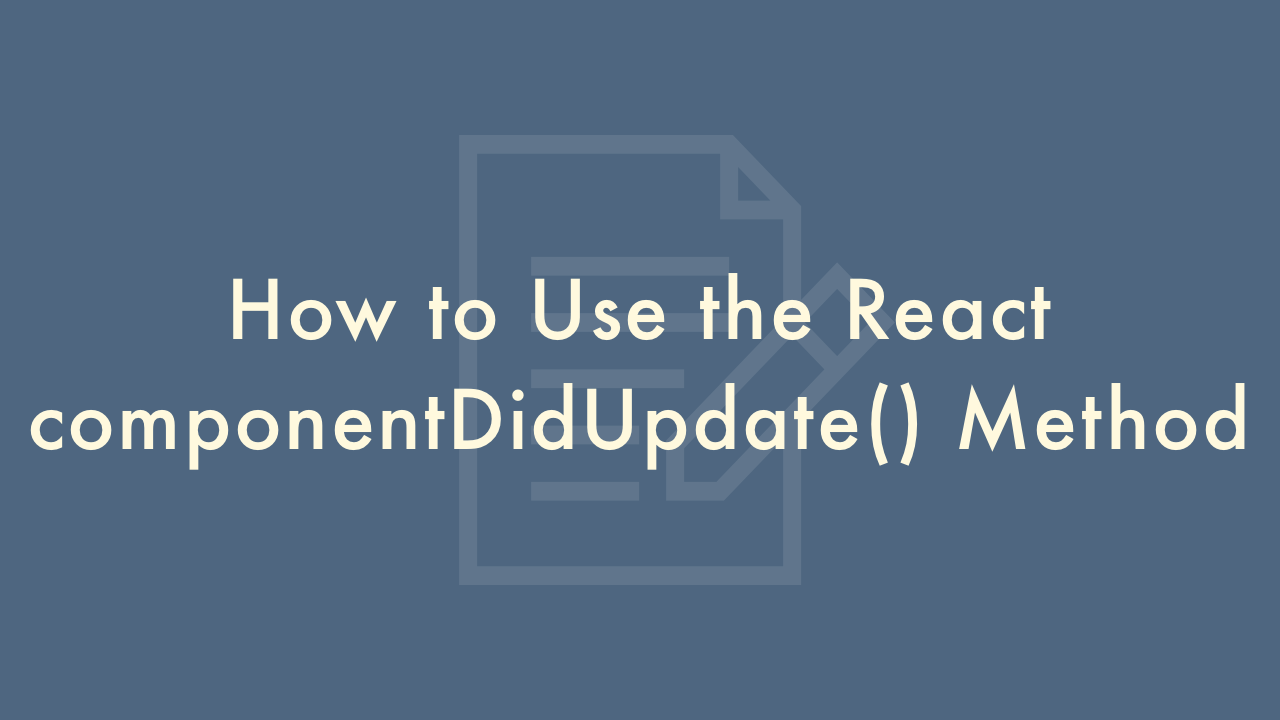
Contents
In this article, you will learn how to use the React componentDidUpdate() method.
Using the componentDidUpdate() method
The componentDidUpdate() method is a lifecycle method in React that gets called immediately after the component updates. It is often used to perform additional actions after a component has been updated, such as updating the component’s state or making API calls.
Here are the steps to use the componentDidUpdate() method:
- Define the componentDidUpdate() method within your React component class.
- In the componentDidUpdate() method, check whether the component’s props or state have changed. You can use the prevProps and prevState parameters to access the previous props and state values.
- If the props or state have changed, perform any necessary actions, such as updating the component’s state or making API calls.
- Be careful not to create an infinite loop by calling setState() within the componentDidUpdate() method. Always check that the new state is different from the previous state before calling setState().
Here’s an example of using the componentDidUpdate() method to make an API call after the component updates:
import React, { Component } from 'react';
class MyComponent extends Component {
state = {
data: null
}
componentDidMount() {
// Make initial API call
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data }));
}
componentDidUpdate(prevProps, prevState) {
// Check if state has changed
if (this.state.data !== prevState.data) {
// Make API call to update data
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data }));
}
}
render() {
return (
<div>
{this.state.data && (
<p>{this.state.data}</p>
)}
</div>
);
}
}
export default MyComponent;
In this example, the componentDidUpdate() method is used to check whether the component’s state has changed. If the state has changed, the method makes another API call to update the data. The updated data is then stored in the component’s state and rendered on the page.