How to Use the React getDerivedStateFromError() Method
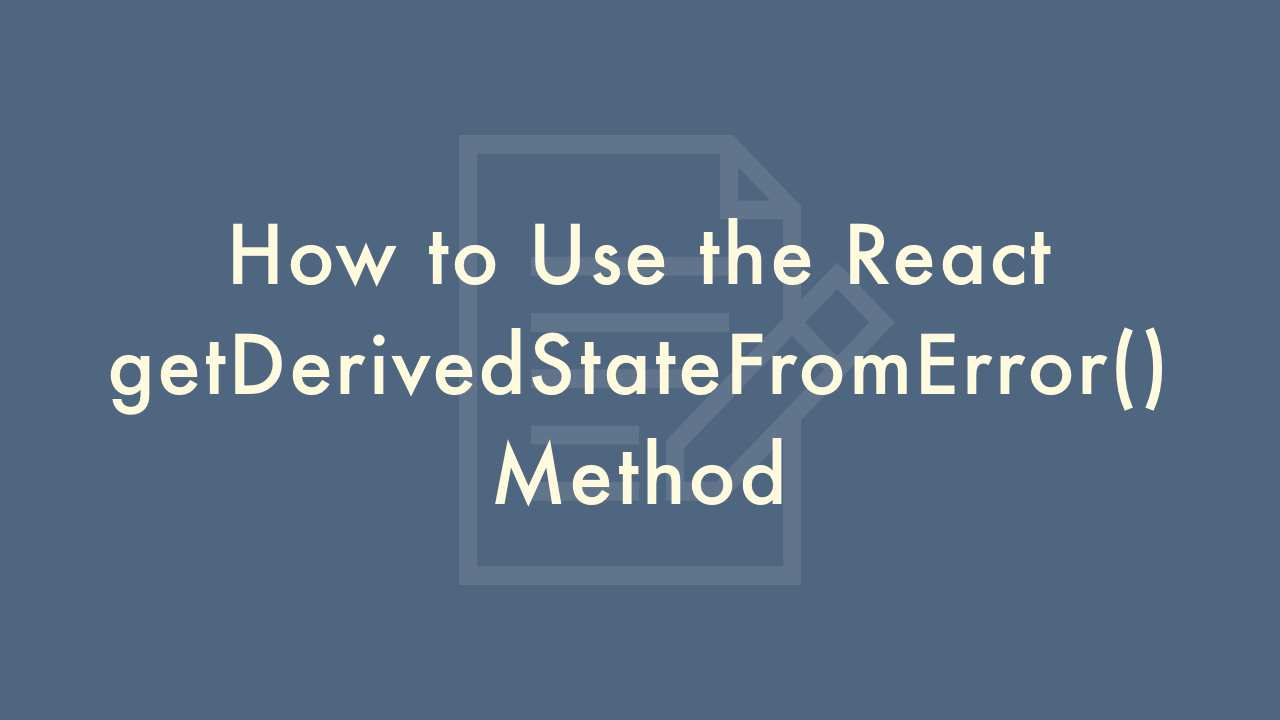
Contents
In this article, you will learn how to use the React getDerivedStateFromError() method.
Using the getDerivedStateFromError() method
The getDerivedStateFromError() method is a lifecycle method introduced in React 16. It is used to handle errors that occur during rendering, and it can be defined on a component class to catch errors thrown by any of its child components.
Here’s how you can use getDerivedStateFromError() in your React components:
Define the getDerivedStateFromError() method in your component class:
class MyComponent extends React.Component {
static getDerivedStateFromError(error) {
// Handle the error here
return { hasError: true };
}
// ...
}
In the method, you can do any error handling that’s necessary. For example, you can set state to indicate that an error has occurred:
static getDerivedStateFromError(error) {
return { hasError: true };
}
You can then use the hasError state to render an error message or a fallback UI:
render() {
if (this.state.hasError) {
return <div>Something went wrong!</div>;
}
// Render your component normally
return (
<div>
<ChildComponent />
</div>
);
}
Note that getDerivedStateFromError() is only called during the rendering phase. It is not called during event handling, or during the constructor() or componentDidMount() methods.
Also note that getDerivedStateFromError() does not catch errors that occur in event handlers or in asynchronous code. To handle those types of errors, you can use a try/catch block, or an error boundary.
Finally, remember to use getDerivedStateFromError() sparingly. It should only be used to handle errors that are unexpected or that cannot be handled in a more specific way. Overusing it can make your code harder to debug and maintain.