How to Use the React getDerivedStateFromProps() Method
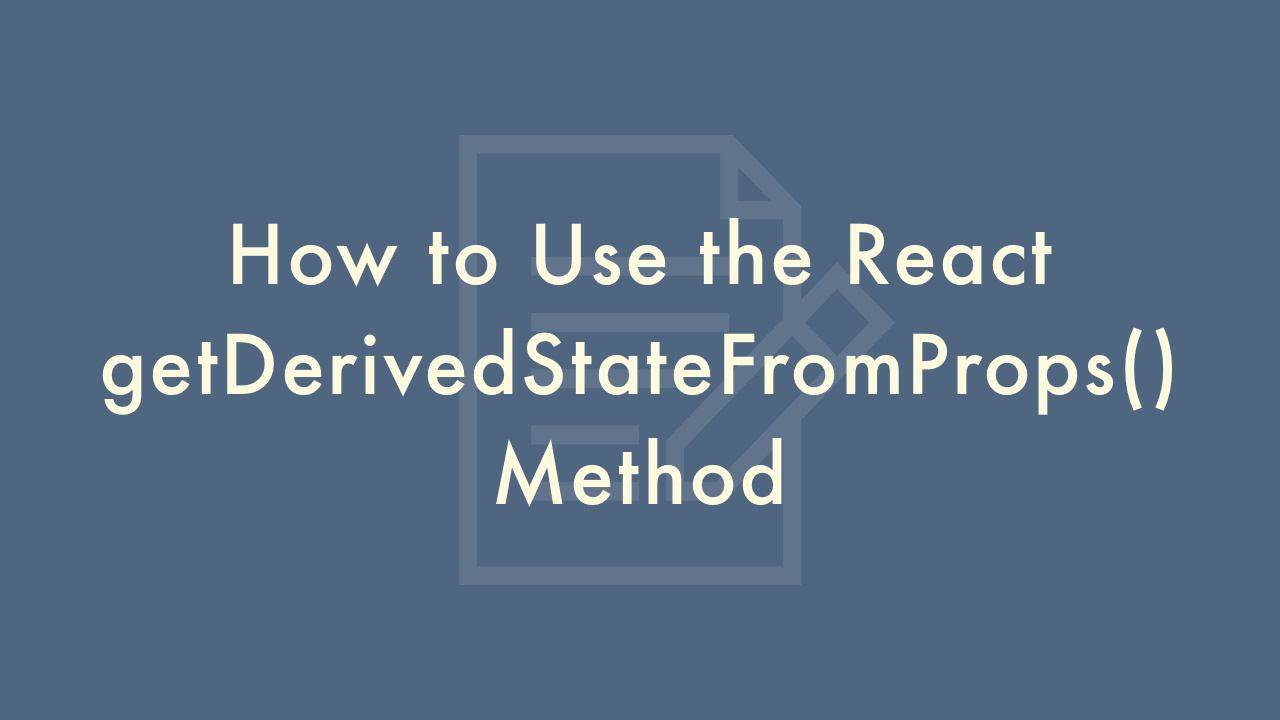
Contents
In this article, you will learn how to use the React getDerivedStateFromProps() method.
Using the getDerivedStateFromProps() method
The getDerivedStateFromProps() method is a lifecycle method in React that allows you to update the state of a component based on changes to its props. It is a static method that takes in two parameters: props and state, and returns an object that represents the updated state of the component.
Here is how you can use getDerivedStateFromProps() in your React components:
Declare the getDerivedStateFromProps() method inside your component class:
class MyComponent extends React.Component {
static getDerivedStateFromProps(props, state) {
// return an object that represents the updated state
}
state = {
// initial state
}
render() {
// render component
}
}
Define the logic for updating the state in the getDerivedStateFromProps() method. This method should return an object that represents the updated state of the component, or null if the state does not need to be updated.
static getDerivedStateFromProps(props, state) {
if (props.someProp !== state.someProp) {
return {
someState: props.someProp
};
}
return null;
}
In this example, the component checks if the value of the someProp prop has changed since the last time the component was rendered. If the value has changed, the method returns an object that updates the someState state with the new value of someProp.
Use the updated state in your component’s render method:
render() {
const { someState } = this.state;
return (
<div>{someState}</div>
);
}
In this example, the component renders the updated value of someState that was returned by the getDerivedStateFromProps() method.
Note that getDerivedStateFromProps() is called before the render() method. So, if you want to use the updated state in the render() method, you should access it using this.state instead of this.props.
render() {
const { someState } = this.state;
return (
<div>{someState}</div>
);
}
Finally, remember that getDerivedStateFromProps() is a static method, which means that you cannot access this inside it. If you need to access the component instance, you can use componentDidUpdate() instead.