How to Use the React useLayoutEffect Hook
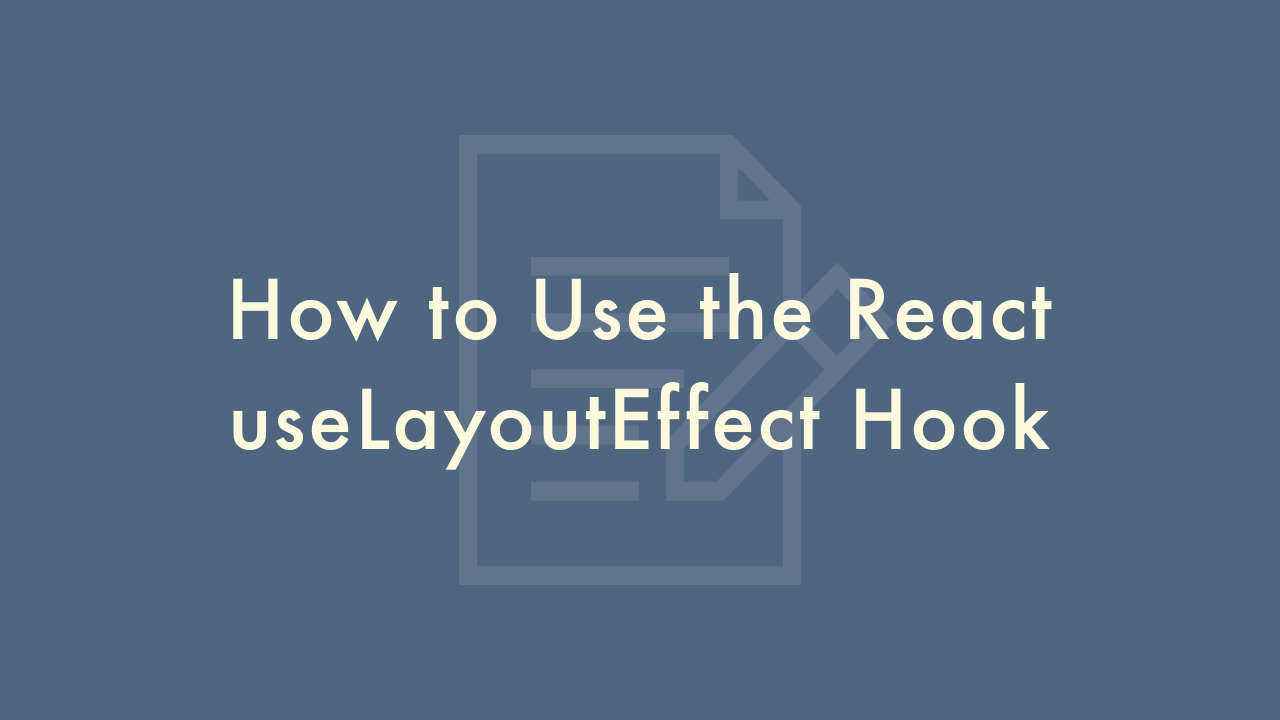
Contents
In this article, you will learn how to use the React useLayoutEffect hook.
Using the useLayoutEffect hook
The useLayoutEffect hook is similar to the useEffect hook in React, but it runs synchronously immediately after all DOM mutations have been processed, but before the browser has had a chance to paint those changes to the screen. This makes it ideal for scenarios where you need to read or manipulate the layout of your component’s DOM elements.
Here’s an example of how to use the useLayoutEffect hook:
import React, { useLayoutEffect, useState } from 'react';
function Example() {
const [height, setHeight] = useState(0);
useLayoutEffect(() => {
const headerEl = document.querySelector('.header');
const height = headerEl.getBoundingClientRect().height;
setHeight(height);
}, []);
return (
<div>
<header className="header">Header</header>
<div style={{ paddingTop: height }}>Content</div>
</div>
);
}
In this example, we want to adjust the padding of the content area to accommodate the height of the header element. We use useLayoutEffect to read the height of the header element using getBoundingClientRect(), and then set the height state variable using setHeight(). The empty dependency array [] passed to useLayoutEffect ensures that the effect runs only once, after the initial render.
Note that because useLayoutEffect runs synchronously before the browser has had a chance to paint the changes, it can cause a delay in the initial rendering of your component. If your effect doesn’t need to manipulate the layout of your component, you should use the useEffect hook instead.