How to Use the React setState() Method
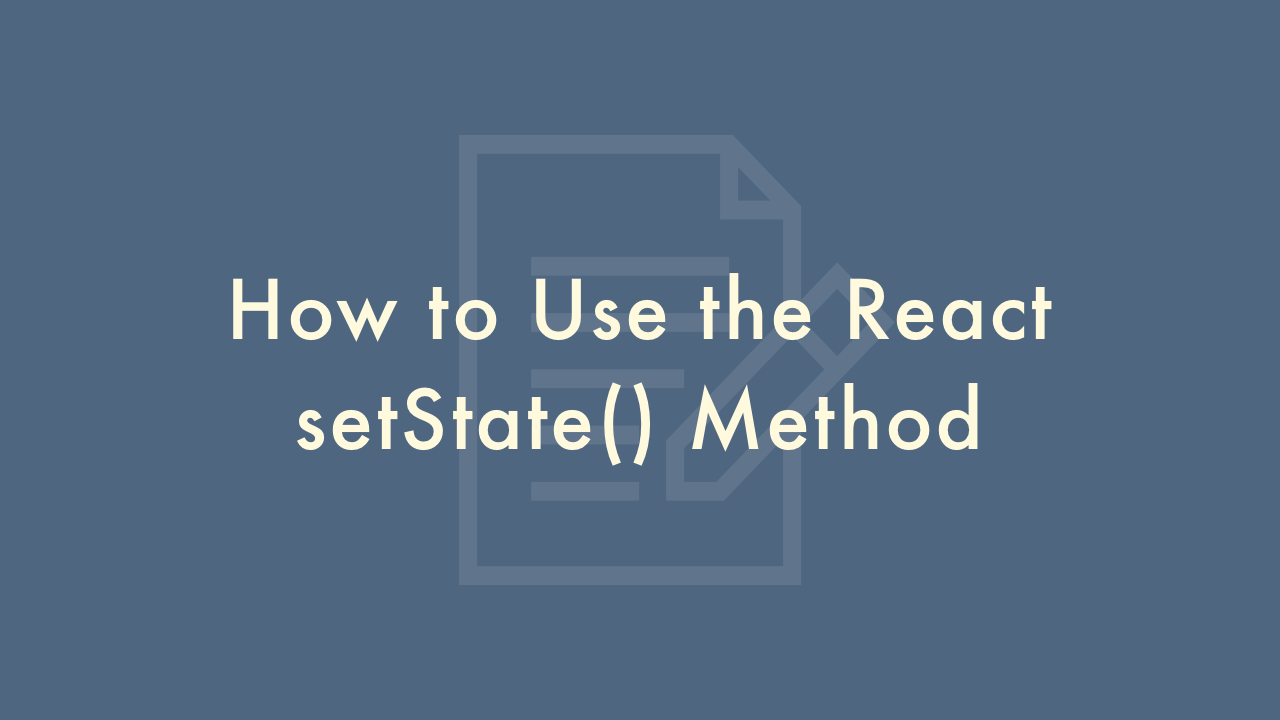
Contents
In this article, you will learn how to use the React setState() method.
Using the setState() method
React is a popular JavaScript library for building user interfaces. In React, state is used to manage component data and render updates. The setState() method is used to update the state of a React component.
Setting state with setState()
The setState() method is used to update the state of a React component. It takes an object as an argument, where the keys are the names of the state properties to be updated and the values are the new values of the properties. For example, if we have a state with a property count, we can update it using the setState() method as follows:
this.setState({ count: 1 });
When the state is updated using setState(), React will re-render the component and any child components that rely on the updated state will also be re-rendered.
Updating state based on previous state
Sometimes, we may want to update the state based on its previous value. For example, if we have a count state property, we may want to increment it by 1. We can achieve this by passing a function to setState(). The function receives the previous state as an argument and returns an object with the updated state values. Here’s an example:
this.setState(prevState => {
return { count: prevState.count + 1 };
});
Using this approach ensures that the state is updated based on the latest value, and not on an outdated value.
Using setState() asynchronously
The setState() method is asynchronous, which means that React may batch multiple state updates together for performance reasons. This can lead to unexpected behavior if we assume that state updates are always processed immediately. To address this, we can pass a callback function as a second argument to setState(). The callback function will be called after the state has been updated and the component has been re-rendered. Here’s an example:
this.setState({ count: 1 }, () => {
console.log('State updated!');
});
Passing a function to setState()
In addition to passing an object to setState(), we can also pass a function that returns an object. This can be useful if we want to compute the new state based on some external factors. For example, we may want to update the state based on the current time. Here’s an example:
this.setState(() => {
const now = new Date();
return { timestamp: now.toISOString() };
});