How to Use the React useState Hook
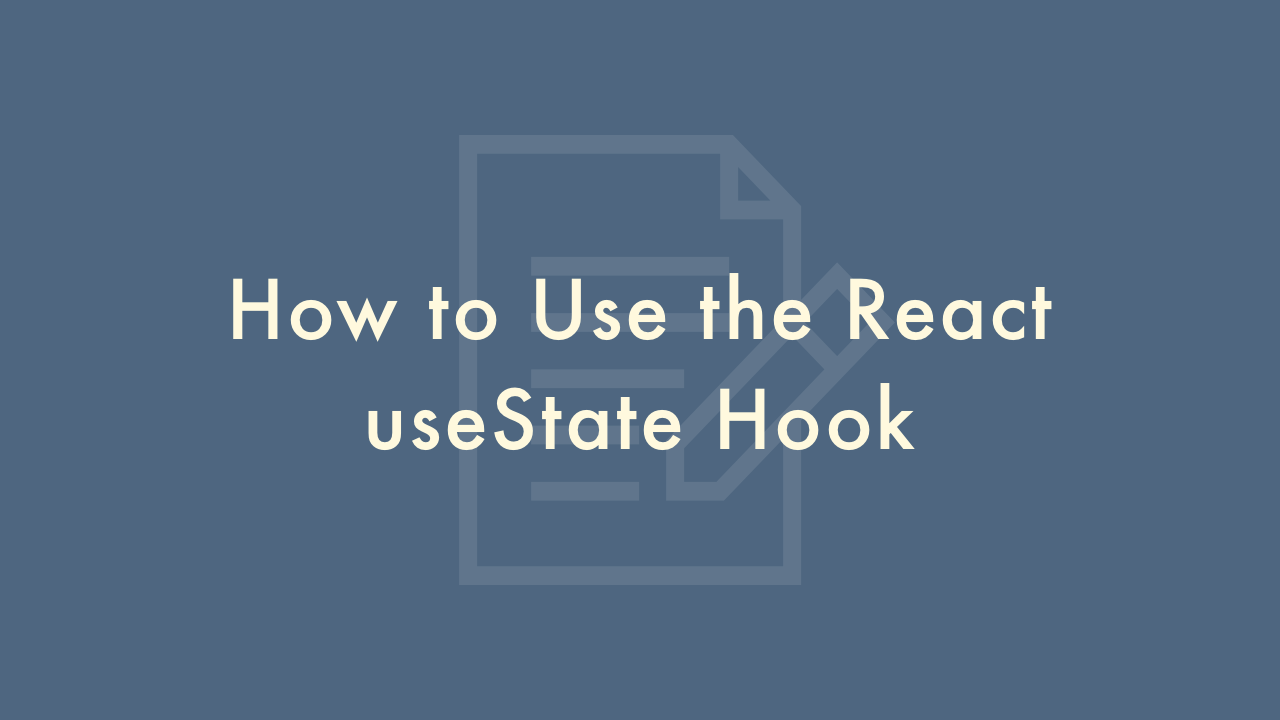
Contents
In this article, you will learn how to use the React useState hook.
Using the useState hook
The React useState hook is a powerful feature that allows developers to manage state within functional components.
Before diving into the implementation details, let’s understand what the useState hook is. The useState hook is a built-in hook in React that allows you to add state to your functional components. It takes an initial state value and returns an array with two elements:
- The current state value
- A function to update the state value
Syntax
The syntax for using the useState hook is as follows:
const [state, setState] = useState(initialState);
Here, state is the current state value, and setState is the function that updates the state value. The useState function takes an initial state value as its argument.
Example
To use the useState hook, you need to import it from the react module. Here’s an example of how to use the useState hook in a functional component:
import React, { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In the above example, we have created a functional component called Example and used the useState hook to add state to it. We have initialized the state value to 0 using useState(0).
We have also defined a count variable that holds the current state value and a setCount function that updates the state value. The setCount function takes the new state value as its argument.
In the return statement, we have rendered a paragraph element that displays the current state value and a button element that calls the setCount function when clicked. The setCount function updates the count variable by adding 1 to it.