How to Use the React shouldComponentUpdate() Method
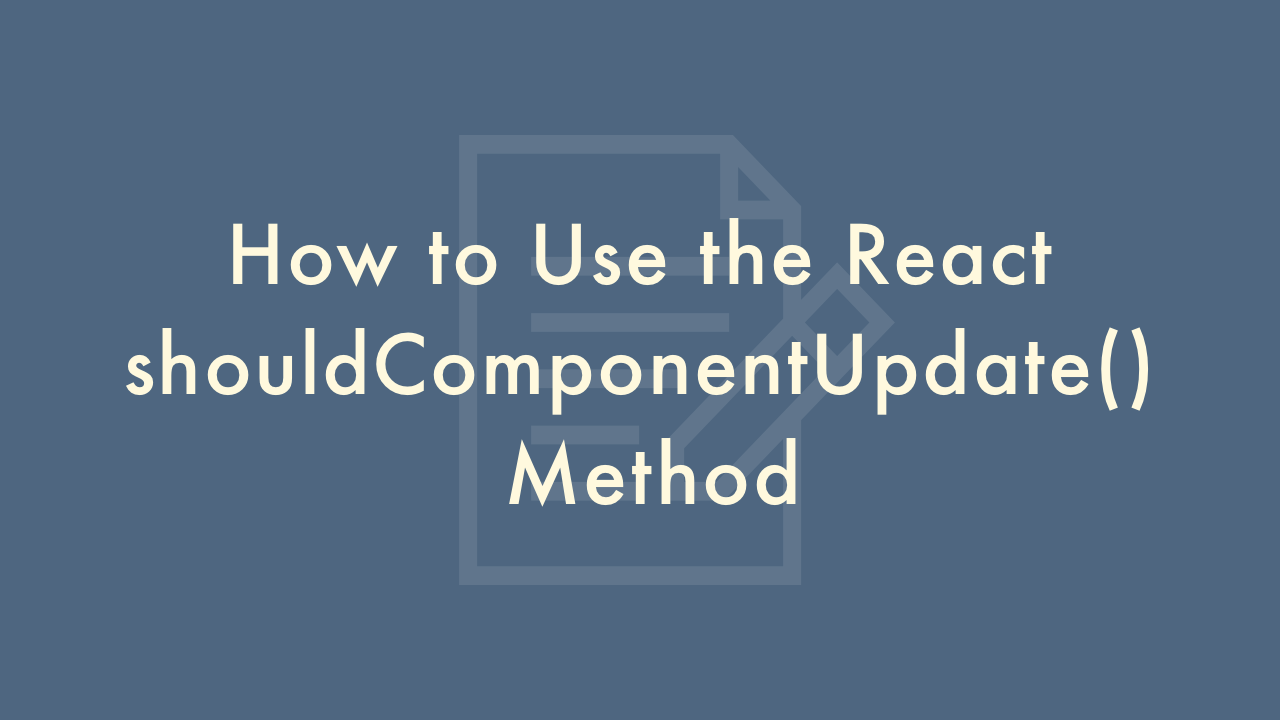
Contents
In this article, you will learn how to use the React shouldComponentUpdate() method.
Using the shouldComponentUpdate() method
The shouldComponentUpdate() method is a lifecycle method in React that allows you to optimize your component’s performance by determining whether or not it should re-render. By default, React will re-render a component whenever its props or state change. However, sometimes it’s not necessary to re-render a component, especially if the props or state haven’t changed.
The shouldComponentUpdate() method is called before the render() method whenever the props or state of a component change. It takes two arguments, nextProps and nextState, which represent the new props and state values that the component will receive. The method should return a boolean value indicating whether or not the component should re-render. If the method returns true, the component will re-render; if it returns false, the component will not re-render.
Here are some tips for using the shouldComponentUpdate() method effectively:
- Use shouldComponentUpdate() only when necessary: It’s important to remember that using shouldComponentUpdate() can make your code more complex and harder to maintain. Only use it when you need to optimize the performance of your component.
- Compare the current props and state with the new props and state: In the shouldComponentUpdate() method, you should compare the current props and state with the new props and state to determine if any changes have occurred. You can use the Object.is() method to compare objects in a performant way.
- Avoid unnecessary re-renders: If the props and state haven’t changed, there’s no need to re-render the component. You can return false from the shouldComponentUpdate() method to prevent unnecessary re-renders.
- Optimize the comparison process: If your component’s props and state are complex objects, comparing them can be time-consuming. You can optimize the comparison process by comparing only the properties that have changed, or by using a library like lodash or immutable.js.
Here’s an example of how to use the shouldComponentUpdate() method:
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
if (Object.is(this.props, nextProps) && Object.is(this.state, nextState)) {
return false; // no need to re-render
}
return true; // re-render
}
render() {
// component render logic
}
}
In this example, the shouldComponentUpdate() method compares the current props and state with the new props and state using the Object.is() method. If they are equal, the method returns false, indicating that there’s no need to re-render the component. If they are not equal, the method returns true, indicating that the component should re-render.