How to Use the React useMemo Hook
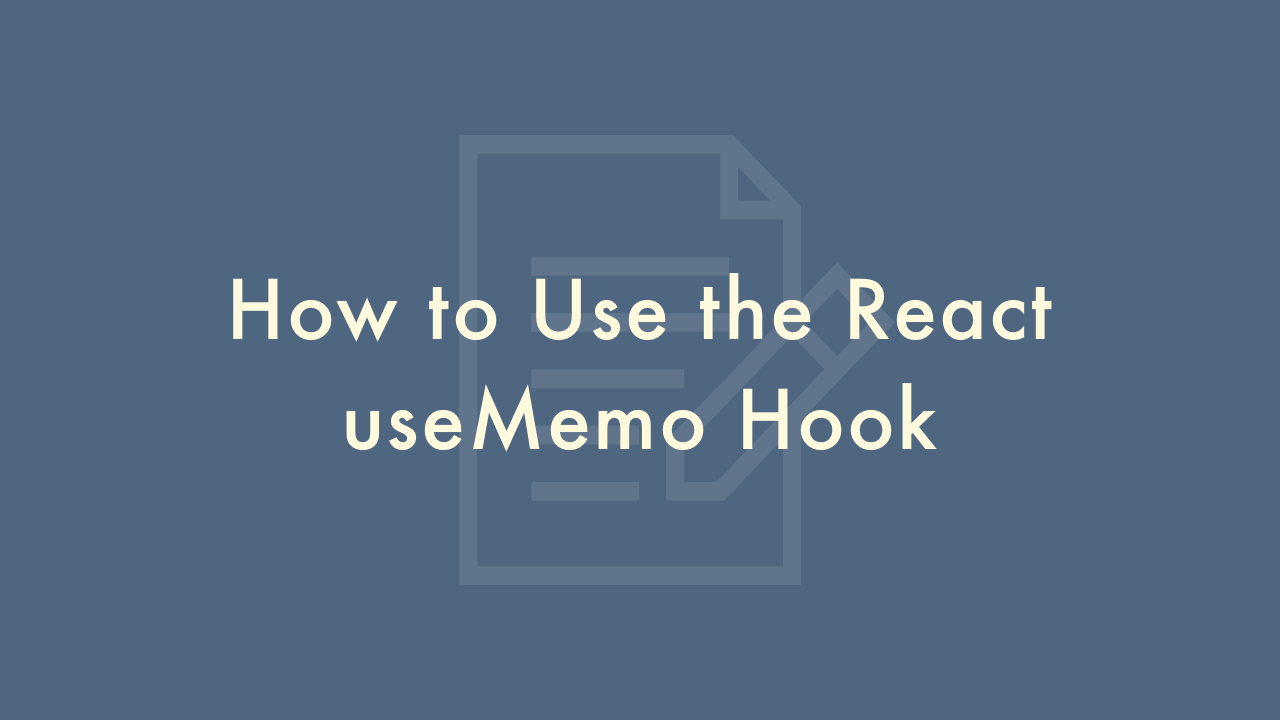
Contents
In this article, you will learn how to use the React useMemo hook.
Using the useMemo hook
The useMemo hook in React is used to memoize the result of a computation, so that it can be cached and returned without re-computing it on every render. This can be useful in situations where the computation is expensive and may not change frequently, or when you need to optimize the performance of your application.
Here’s how you can use the useMemo hook in your React application:
Import the useMemo hook from the react library:
import React, { useMemo } from 'react';
Define a function that performs the computation you want to memoize:
function expensiveComputation(a, b) {
// perform an expensive computation here
return a * b;
}
Inside your functional component, call the useMemo hook and pass in the computation function and an array of dependencies:
function MyComponent(props) {
const { a, b } = props;
const result = useMemo(() => expensiveComputation(a, b), [a, b]);
return {result};
}
In this example, expensiveComputation is called only when either a or b changes. The result is then cached and returned from the useMemo hook. If neither a nor b changes, the cached result is returned instead of re-computing it.
Here’s a breakdown of the useMemo hook parameters:
- The first parameter is a function that performs the computation you want to memoize.
- The second parameter is an array of dependencies that the computation function depends on. If any of the dependencies change, the computation function will be re-run.
- The hook returns the memoized value of the computation function.