What are React Hooks?
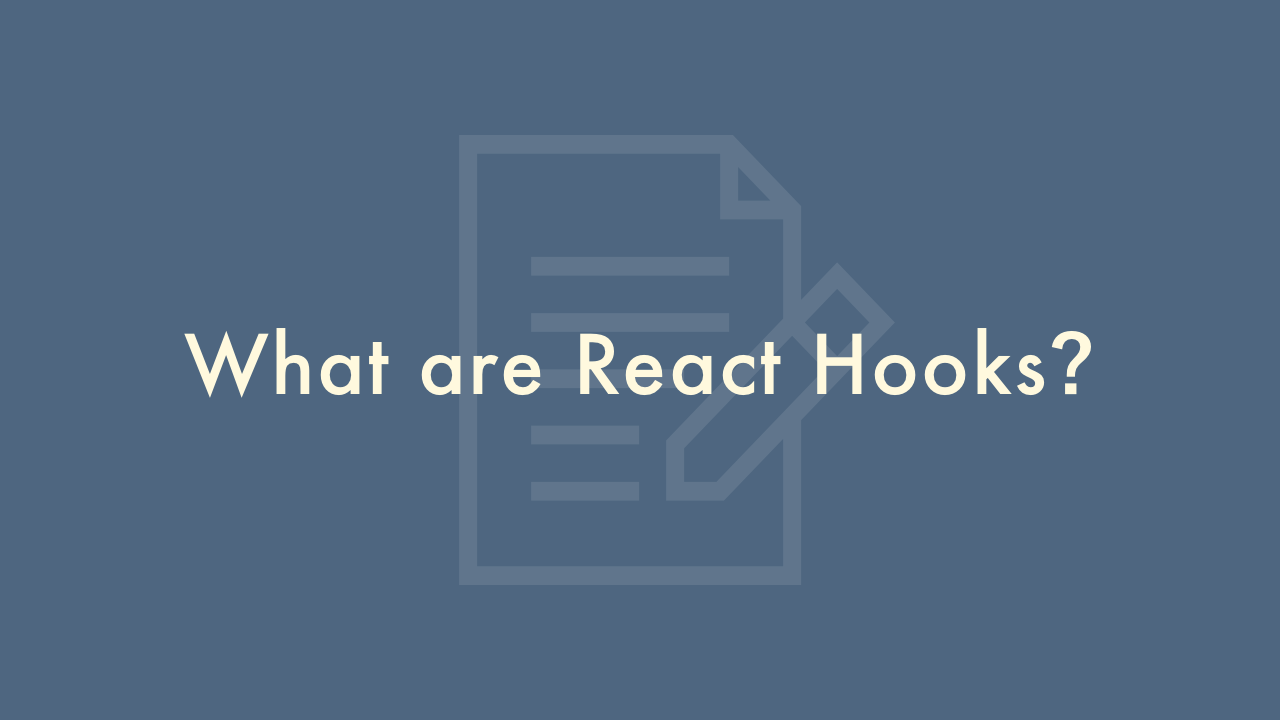
Contents
In this article, you will learn about React hooks.
What are React Hooks?
React Hooks is a feature introduced in React 16.8 that allows developers to use state and other React features without writing a class. Hooks are functions that let you use React state and lifecycle methods in functional components.
Before the introduction of hooks, the only way to add state to a functional component was to use the “useState” higher-order function. Hooks provide a more concise and readable way to manage state and other React features like context and lifecycle methods.
Some commonly used hooks are:
useState
: This hook allows us to add state to a functional component. It takes an initial value and returns a pair of values, the current state value and a function to update it.useEffect
: This hook is used to perform side effects such as fetching data or updating the DOM. It takes a function that is executed after every render and optionally a list of dependencies that control when the effect runs.useContext
: This hook is used to consume context created by a “Provider” component. It takes a context object created by “createContext” and returns the current value of the context.useMemo
: This hook is used to memoize expensive computations so that they are only executed when necessary. It takes a function and a list of dependencies and returns the memoized value.useCallback
: This hook is used to memoize functions so that they are only re-created when their dependencies change. It takes a function and a list of dependencies and returns a memoized version of the function.useRef
: This hook returns a mutable ref object that can be used to store a value that persists between renders. It’s often used to access the underlying DOM node of a component.useReducer
: This hook is an alternative to useState that allows you to manage more complex state that involves multiple values or has complex update logic. It takes a reducer function and an initial state value and returns the current state value and a dispatch function that can be used to update the state.useLayoutEffect
: This hook is similar to useEffect but it runs synchronously after all DOM mutations have been applied. This makes it useful for measuring the size or position of elements after they have been rendered.
Overall, hooks provide a simpler and more flexible way to manage state and other React features in functional components. They can help you write more concise and maintainable code, and they make it easier to share logic between components. If you’re new to React, learning hooks should be a top priority.