How to Sort Arrays in PHP
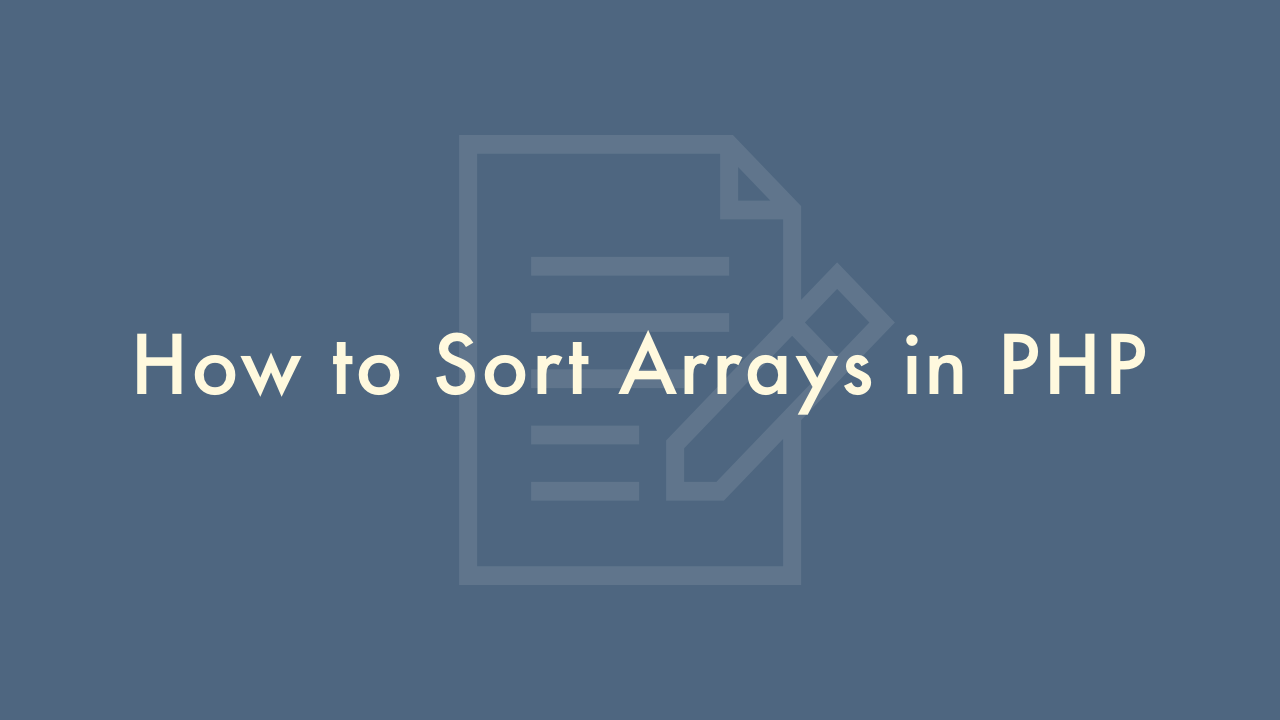
Contents
In this article, you will learn how to sort arrays in PHP.
PHP sort() Function
The sort() function sorts the elements of an array in ascending order. It reorders the keys of the array, so the first element will have an index of 0, the second element will have an index of 1, and so on.
Syntax:
sort($array);
Example:
<?php
$fruits = array("lemon", "orange", "banana", "apple");
sort($fruits);
print_r($fruits);
// Output:
// Array
// (
// [0] => apple
// [1] => banana
// [2] => lemon
// [3] => orange
// )
?>
PHP rsort() Function
The rsort() function sorts the elements of an array in descending order. It also reorders the keys of the array.
Syntax:
rsort($array);
Example:
<?php
$fruits = array("lemon", "orange", "banana", "apple");
rsort($fruits);
print_r($fruits);
// Output:
// Array
// (
// [0] => orange
// [1] => lemon
// [2] => banana
// [3] => apple
// )
?>
PHP asort() Function
The asort() function sorts the elements of an associative array in ascending order, preserving the association between the keys and values. This means that the key of each element will not be changed.
Syntax:
asort($array);
Example:
<?php
$fruits = array("a"=>"lemon", "b"=>"orange", "c"=>"banana", "d"=>"apple");
asort($fruits);
print_r($fruits);
// Output:
// Array
// (
// [d] => apple
// [c] => banana
// [a] => lemon
// [b] => orange
// )
?>
PHP arsort() Function
The arsort() function sorts the elements of an associative array in descending order, preserving the association between the keys and values.
Syntax:
arsort($array);
Example:
<?php
$fruits = array("a"=>"lemon", "b"=>"orange", "c"=>"banana", "d"=>"apple");
arsort($fruits);
print_r($fruits);
// Output:
// Array
// (
// [b] => orange
// [a] => lemon
// [c] => banana
// [d] => apple
// )
?>
PHP usort() Function
The usort() function allows you to sort an array using a custom comparison function. The comparison function takes two arguments, and it should return 0 if the two elements are equal, 1 if the first element should be sorted before the second element, or -1 if the second element should be sorted before the first element. The usort() function sorts the elements of the array based on the result of the comparison function.
Syntax:
usort($array, $comparison_function);
Example:
<?php
function cmp($a, $b) {
return strcmp($a["fruit"], $b["fruit"]);
}
$fruits = array(
array("fruit" => "lemon", "color" => "yellow"),
array("fruit" => "banana", "color" => "yellow"),
array("fruit" => "apple", "color" => "red"),
array("fruit" => "orange", "color" => "orange"),
);
usort($fruits, "cmp");
print_r($fruits);
// Output:
// Array
// (
// [0] => Array
// (
// [fruit] => apple
// [color] => red
// )
//
// [1] => Array
// (
// [fruit] => banana
// [color] => yellow
// )
//
// [2] => Array
// (
// [fruit] => lemon
// [color] => yellow
// )
//
// [3] => Array
// (
// [fruit] => orange
// [color] => orange
// )
// )
?>
These functions can be used to sort arrays of any data type, including arrays of numbers, strings, and objects.