How to Use the PHP pow() Function
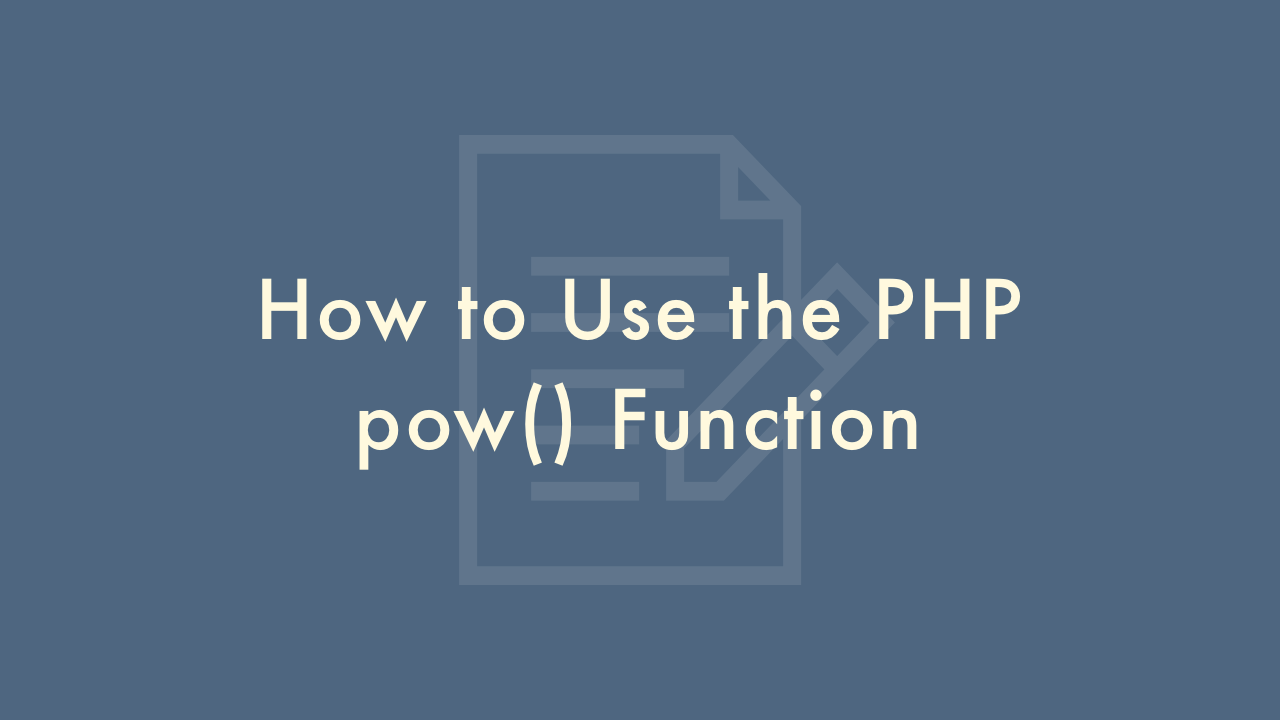
Contents
In this article, you will learn how to use the PHP pow() function.
PHP pow() Function
The pow() function in PHP is used to calculate the power of a number. The function takes two arguments: the base and the exponent. The function returns the result of the base raised to the power of the exponent.
The pow() function is a built-in function in PHP and can be used to perform mathematical calculations. It is commonly used to perform exponential calculations, where one number is raised to the power of another number.
Syntax:
pow($base, $exponent);
Example:
<?php
$result = pow(10, 2); // 100
$result = pow(2, 10); // 1024
$result = pow(3, 3); // 27
$result = pow(4, 0.5); // 2 (square root of 4)
?>
It’s important to note that the base and exponent arguments passed to the pow() function must be numeric values, either integers or floating-point numbers. If either of the arguments is not a numeric value, an error will occur.
In addition to the basic power calculation, the pow() function can also be used to perform modular arithmetic. To perform modular arithmetic, you can pass a third argument to the pow() function, which is the modulo. The result of the calculation will be the remainder when the result of the power calculation is divided by the modulo.
Here’s an example:
<?php
$result = pow(2, 10, 100); // 24
?>
In this example, 2 raised to the power of 10 is 1024. However, since we passed a third argument (100), the result of the calculation is the remainder when 1024 is divided by 100, which is 24.