How to Send Emails with the PHP mail() function
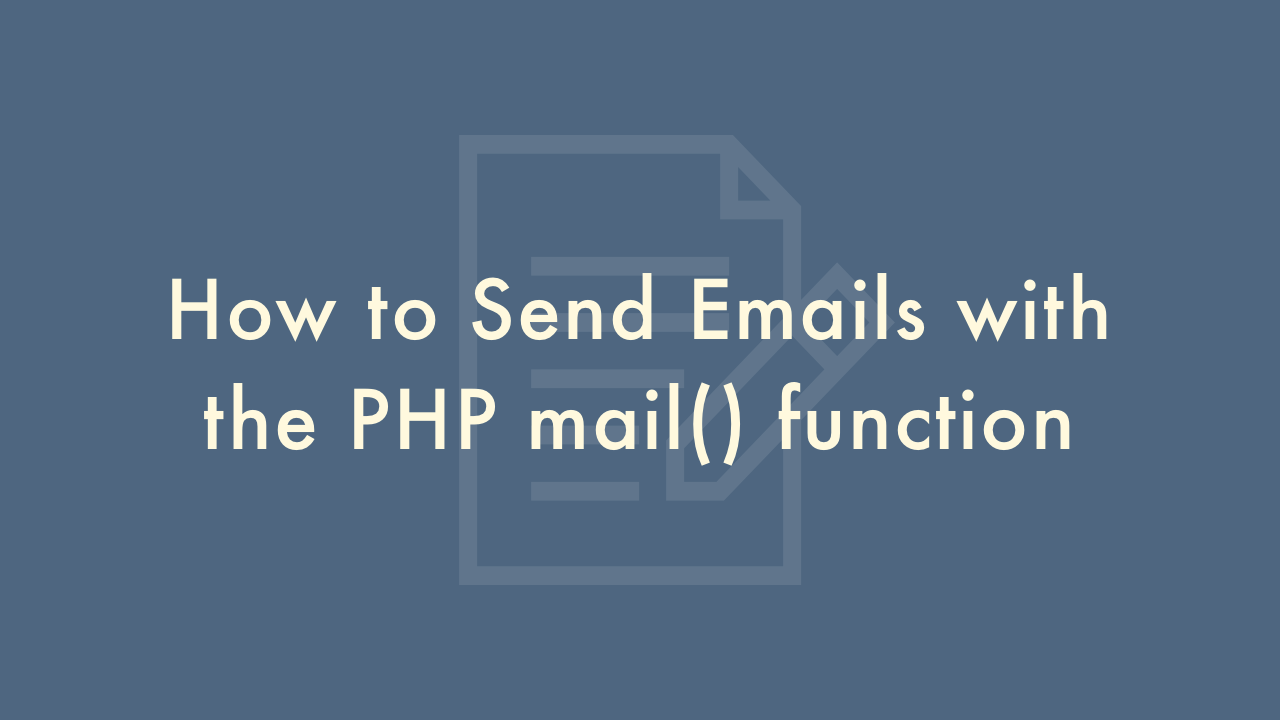
Contents
In this article, you will learn how to send Emails in PHP.
PHP mail() Function
To send an emails in PHP you can use the mail() function.
Syntax:
mail($to, $subject, $message, $headers, $parameters);
Parameters:
$to
: The recipient’s email address.
$subject
: The subject of the email.
$message
: The message body of the email.
$headers
: Additional headers (e.g. CC, BCC, From).
This parameter is optional.
$parameters
: Additional parameters (e.g. sendmail options).
This parameter is optional.
It’s worth noting that, to send an email through PHP’s built-in mail() function, your server must have a mail server installed and configured properly. If your server is not configured, you will have to use an external service to send emails, such as SendGrid, Amazon SES, or Gmail to send emails. There are also several libraries available that can be used to send email in PHP such as PHPMailer, SwiftMailer, and Zend_Mail.
Example
Here is an example of how to send emails with the mail() function:
<?php
$to = "recipient@example.com";
$subject = "Test";
$message = "Hello World!";
$headers = "From: sender@example.com" . "\r\n" . "CC: copy@example.com";
if (mail($to, $subject, $message, $headers)) {
echo "Email sent successfully.";
} else {
echo "Failed to send email.";
}
?>
To learn more about sending emails in PHP, you can read the official documentation for the mail() function on the PHP website:
http://php.net/manual/en/function.mail.php
You can also look into using a library such as PHPMailer, which is a popular and widely used library for sending emails in PHP. It provides an easy-to-use, object-oriented interface for sending emails and it also supports different transports such as sendmail, SMTP, and more.
You can find more information about the library and how to use it here:
https://github.com/PHPMailer/PHPMailer
Another popular library for sending emails in PHP is SwiftMailer. It provides an object-oriented API for sending emails and also supports sending email via different transports such as SMTP, sendmail, and more.
You can find more information about the library and how to use it here:
https://swiftmailer.symfony.com/
It’s important to note that when sending emails, you must ensure that you are in compliance with all relevant laws and regulations, including but not limited to the CAN-SPAM Act in the US and the General Data Protection Regulation (GDPR) in the EU.
You should also ensure that your email server is properly configured and that you are using a reputable email service provider to avoid your emails being marked as spam.