How to Use the PHP array_diff() Function
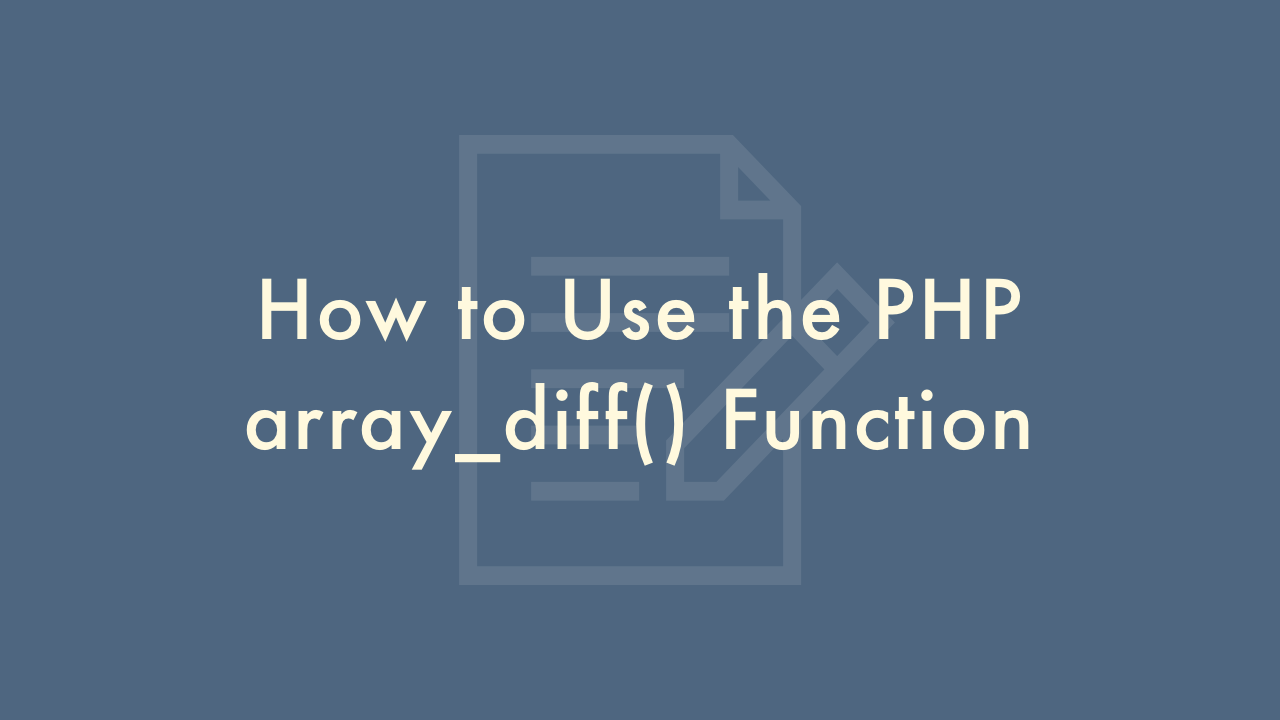
Contents
In this article, you will learn how to use the PHP array_diff() function.
PHP array_diff() Function
The array_diff() function compares two or more arrays and returns an array that contains the values that are not present in any of the other arrays. It is a useful function when you need to compare arrays and determine the unique values between them.
Syntax:
array_diff(array $array1, array $array2[, array $array3 [, ...]])
Example:
<?php
$array1 = array("a" => "green", "red", "blue");
$array2 = array("b" => "green", "yellow", "red");
$result = array_diff($array1, $array2);
print_r($result);
// Output:
// Array ( [1] => blue )
?>
In this example, array_diff is used to find the differences between two arrays $array1 and $array2. The result of this function is an array containing only elements from $array1 that are not present in $array2.
You can also compare arrays with different key types. If the arrays have string keys, the keys are used in the comparison. If the arrays have numeric keys, the values are compared in the order they appear in the arrays.
Here’s an example of comparing arrays with different key types:
<?php
$array1 = array("a" => "green", "b" => "red", "c" => "blue");
$array2 = array("b" => "green", "c" => "yellow", "d" => "red");
$result = array_diff($array1, $array2);
print_r($result);
// Output:
// Array ( [c] => blue )
?>
In this example, array_diff compares two arrays with string keys, $array1 and $array2. The result is an array containing only the key-value pair from $array1 that is not present in $array2.