How to Rename a File in PHP
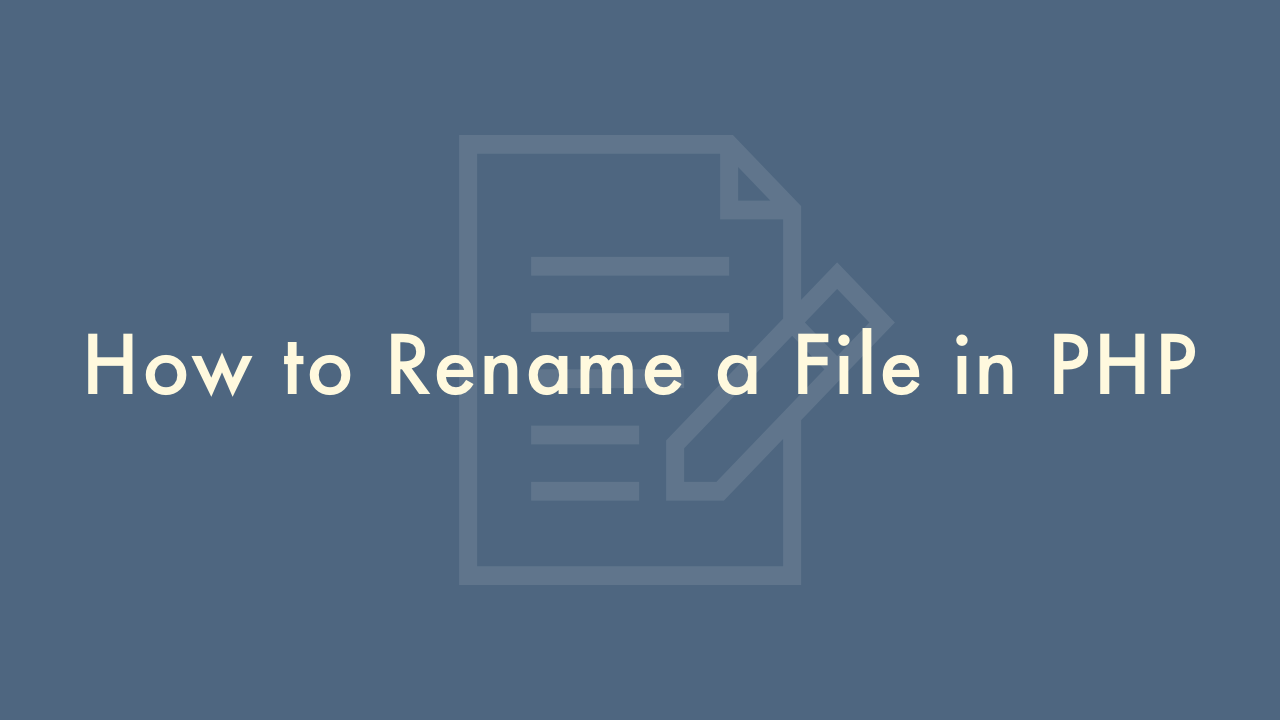
09/06/2021
Contents
In this article, you will learn how to rename a file in PHP.
PHP rename() Function
In PHP, you can rename a file using the rename() function.
The rename() function returns true on success and false on failure. You can use the if statement to check the return value and handle the error accordingly.
Syntax:
bool rename ( string $oldname, string $newname [, resource $context ] )
Parameters:
$oldname
: The original name of the file or directory that you want to rename.$newname
: The new name for the file or directory.$context
(optional): This is a stream context resource that can be used to modify the behavior of the rename() function.
Example:
<?php
$old_file_name = "old_file.txt";
$new_file_name = "new_file.txt";
if (rename($old_file_name, $new_file_name)) {
echo "File renamed successfully.";
} else {
echo "Error renaming file.";
}
?>
Here’s some additional information about the rename() function in PHP:
- The rename() function can be used to rename both files and directories.
- The rename() function works by calling the underlying operating system’s rename function, so it’s subject to the same restrictions as that function. For example, you can’t rename a file to a name that already exists, unless you use the overwrite parameter in some systems.
- If the new file name is on a different file system, rename() will not work and return false. You can use copy and unlink functions to move a file to a different file system.
- The rename() function also changes the file’s owner and permissions, so make sure you have the necessary permissions before renaming a file.
- If the file you’re renaming is open, the rename() function may fail. Make sure to close any open handles to the file before renaming it.